- Checkmarx Documentation
- Checkmarx One
- Checkmarx One User Guide
- Scan Management
- SAST Query Editor
- SAST Query Language APIs
SAST Query Language APIs
The following is a list of APIs in SAST to interact with its query language to find vulnerability patterns to improve the accuracy of your scans. If you are using Checkmarx SAST On-Prem, use these APIs in Audit . If you are using Checkmarx One, use them in Query Editor.
Add
Adds to the current instance the given graph node, indexed by the given id.
Expand source
public void Add(int a, IGraph b)
a Id of the node to be added to the graph node.
b Graph node to be associated to the given Id.
ArgumentNullException : Thrown when parameter is a null reference.
This example demonstrates the CxList.Add(int, IGraph) method.
The input source code is:
Expand source
int b, a = 5; if (a == 33) b = 6;
The source code that uses CxList.Add method:
Expand source
CxList myList = All.FindByName(“a”); CSharpGraph nodeGraph = All.FindByName(“b”).GetFirstGraph(); myList.Add(nodeGraph.NodeId, nodeGraph); result = myList;
The resulting list will include the initial two “a”’s and the first b.
Add all the elements from the given CxList to the current instance.
Expand source
public void Add(CxList a)
a The CxList to be added to the current CxList instance.
ArgumentNullException : Thrown when parameter is a null reference.
This example demonstrates the CxList.Add(CxList) method.
The input source code is:
Expand source
int b, a = 5; if (a == 33) b = 6;
The source code that uses CxList.Add method:
Expand source
CxList list_a = All.FindByName(“a”); CxList list_b = All.FindByName(“b”); list_a.Add(list_b); result = list_a;
The resulting list will contain 4 elements.
Adds to the current instance all the elements from the given CxLists.
Expand source
public void Add(IEnumerable<CxList> a)
a A list of CxLists.
ArgumentNullException : Thrown when parameter is a null reference.
This example demonstrates the CxList.Add(IEnumerable<CxList>) method.
The input source code is:
Expand source
int b, a = 5; if (a == 33) b = 6;
The source code that uses CxList.Add method:
Expand source
CxList a = All.FindByName(“a”); CxList b = All.FindByName(“b”); CxList integers = All.FindByType(typeof(IntegerLiteral)); Result.AddRange(new List<CxList>(){a, b, integers});
The resulting list will include the two “a”’s, two “b”’s and the numbers 5, 33, 6.
Adds to the current instance all the elements from the given CxLists.
Expand source
public void Add(params CxList[] lists)
lists An array of CxLists.
ArgumentNullException : Thrown when parameter is a null reference.
This example demonstrates the CxList.Add(params CxList[]) method.
The input source code is:
Expand source
int b, a = 5; if (a == 33) b = 6;
The source code that uses CxList.Add method:
Expand source
CxList a = All.FindByName(“a”); CxList b = All.FindByName(“b”); CxList integers = All.FindByType(typeof(IntegerLiteral)); Result.Add(a, b, integers);
The resulting list will include the two "a"'s, two "b"'s and the numbers 5, 33, 6.
Supported from 9.1.0
Add the given pair to the current CxList instance.
Expand source
public void Add((KeyValuePair<int, IGraph> dic)
dic Pair to be added to the current CxList instance.
ArgumentNullException : Thrown when parameter is a null reference.
This example demonstrates the CxList.Add(KeyValuePair<int, IGraph>) method.
The input source code is:
Expand source
int b, a = 5; if (a == 33) b = 6;
The source code that uses CxList.Add method:
Expand source
CxList myList = All.FindByName(“a”); foreach(KeyValuePair<int, IGraph> entry in All.FindByName(“b”)) { myList.Add(entry); } result = myList;
The resulting list will contain 4 elements.
AddRange
Adds to the current instance all the elements from the given CxLists.
Expand source
public void AddRange(IEnumerable<CxList> lists)
lists A list of CxLists.
ArgumentNullException : Thrown when parameter is a null reference.
This example demonstrates the CxList.AddRange(IEnumerable<CxList>) method.
The input source code is:
Expand source
int b, a = 5; if (a == 33) b = 6;
The source code that uses CxList.AddRange method:
Expand source
CxList a = All.FindByName("a"); CxList b = All.FindByName("b"); CxList integers = All.FindByType(typeof(IntegerLiteral)); Result.AddRange(new List<CxList>(){a, b, integers});
The resulting list will include the two "a"'s, two "b"'s and the numbers 5, 33, 6.
Supported from 9.1.0
AddSupportForExpressionLanguageForFramework
add the support of a framework expression language
frameworkName the framework that we are mapping the marker to
AttributesIgnoreCase
Gets the elements with the specified System.Xml.Linq.XName.
doc The element.
name The System.String to match.
ignoreCase If set to true case will be ignored whilst searching for the System.Xml.Linq.XElement.
A System.Xml.Linq.XElement that matches the specified System.Xml.Linq.XName, or null.
CalcPragmaKey
function to calculate pragma unique id
nodeId None
None
CallingMethodOfAny
Returns a CxList which is a subset of “this” instance and are methods or constructors declarations which matches the given CxList elements.
Expand source
public CxList CallingMethodOfAny(CxList list)
cxList The list of elements containing the methods or constructors to look for their declaration.
ArgumentNullException : Thrown when parameter is a null reference.
This example demonstrates the CxList.CallingMethodOfAny(CxList) method.
The input source code is:
Expand source
void foo() { int goo = 3; int boo = 5; }
The source code that uses CxList.CallingMethodOfAny method:
Expand source
result = All.CallingMethodOfAny(All.FindByName ("oo"));
The result would consist of 1 item:
Expand source
foo (in void foo())
Clear
Clears the information in “this” instance.
Expand source
public bool Clear()
This method removes all the information stored in the List.
This example demonstrates the CxList.Clear() method.
Expand source
CxList MyList = All ; MessageBox.Show(MyList.Count.ToString()); MyList.Clear(); MessageBox.Show(MyList.Count.ToString());
ClearAdditionalNodes
Clears all the data allocated in the _GraphToNodeAdditional and _NodeToGraphAdditional data members for the current thread.
ClearPaths
remove all flow
ClearTopLevelQueryFlag
Call this method at the finally clause of every query.
Clone
create duplicate of this
None
Clone the current (this) CxList
Expand source
public CxList Clone ()
CxList containing a clone of the current (this) CxList
The source code that uses CxList.Clone method:
Expand source
CxList A = All.FindByType(typeof(UnknownReference)); CxList B = A; //B points to same elements as A CxList B = A.Clone(); //B has a copy (clone) of the elements in A
Concatenate
Concatenates two nodes into a flow.
Expand source
public CxList Concatenate (CxList list)
list A CxList containing one node only. This node will be concatenated to this instance.
A flow that starts with this instance node, and ends with the list parameter node.
ArgumentNullException : Thrown when parameter is a null reference.
This function calls CxList.Concatenate(list, false).
If either this instance or list parameter contains more than one node or contains flows, the function return value is undefined.
This function is deprecated, use ConcatenatePath instead.
Supported from v7.1.2
Concatenates two nodes into a flow.
Expand source
public CxList Concatenate (CxList list, bool _testFlow)
list A CxList containing one node only. This node will be concatenated to this instance.
testFlow If true, searches for a flow between this instance and list. Otherwise, connects the two nodes directly (more efficient).
A flow that starts with this instance node, and ends with the list parameter node.
ArgumentNullException : Thrown when parameter is a null reference.
If either this instance or list parameter contains more than one node or contains flows, the function return value is undefined.
This function is deprecated, use ConcatenatePath instead.
This example demonstrates the Concatenate(CxList, bool) method.
The input source code is:
Expand source
void main() { int a = 1; int b = 2; int c = a + b; printf("%d", c); }
The source code that uses CxList.Concatenate method:
Expand source
CxList one = All.FindByName("1"); CxList two = All.FindByName("2"); result = one.Concatenate(two, false);
The result would be:
Expand source
1 flow found: [1] -> [2]
Supported from 7.1.2
ConcatenateAllPaths
Concatenates all flows in this instance to all flows in list.
Expand source
public CxList ConcatenateAllPaths (CxList secondList, bool _testFlow)
secondList A CxList containing flows. These flow will be concatenated to the flows in this instance.
testFlow If true, searches for a flow between this instance and list. Otherwise, connects the two flows directly (more efficient).
A product of all flows in this instance with the ones in list parameter.
ArgumentNullException : Thrown when parameter is a null reference.
If this instance contains n flows in it and list contains m flows in it, the return set will contain nxm flows, where each flow from this instance will be concatenated to each flow from list.
This example demonstrates the ConcatenateAllPaths(CxList, bool) method.
The input source code is:
Expand source
void main() { int a = 1; int b = 2; }
The source code that uses CxList.ConcatenateAllPaths method:
Expand source
CxList one = All.FindByName("1"); CxList a = All.FindByShortName("a").FindByType(typeof(Declarator)); CxList flow1 = a.InfluencedBy(one); // [1] -> [a] CxList two = All.FindByName("2"); CxList b = All.FindByShortName("b").FindByType(typeof(Declarator)); CxList flow2 = b.InfluencedBy(two); // [2] -> [b] CxList flow = flow1 + flow2; result = flow.ConcatenateAllPaths(flow, false);
The result would be:
Expand source
4 flow found: [1] -> [a] -> [1] -> [a] [1] -> [a] -> [2] -> [b] [2] -> [b] -> [1] -> [a] [2] -> [b] -> [2] -> [b]
Supported from 7.1.2
Concatenates all flows in this instance to all flows in list.
Expand source
public CxList ConcatenateAllPaths (CxList secondList)
secondList A CxList containing flows. These flow will be concatenated to the flows in this instance.
A product of all flows in this instance with the ones in list parameter.
ArgumentNullException : Thrown when parameter is a null reference.
This function calls CxList.ConcatenateAllPaths(list, true).
If this instance contains n flows in it and list contains m flows in it, the return set will contain nxm flows, where each flow from this instance will be concatenated to each flow from list.
Supported from 7.1.2
ConcatenateAllSources
Concatenates the node in list to each node in this instance. Concatenation is node-to-node (doesn't support connecting flows).
Expand source
public CxList ConcatenateAllSources (CxList list, bool _testFlow)
list A CxList. It will be concatenated to each node in this instance.
testFlow If this parameter true -> test possible flow , otherwise connect directly.
Flows that starts with this instance nodes, and end with the list parameter node.
ArgumentNullException : Thrown when parameter is a null reference.
If the list parameter contains more than one node or contains flows or this instance contains flows, the function return value is undefined.
The number of the returned items is same as the number of items in this instance.
This function calls the Concatenate function for each item in this instance with list as parameter.
This example demonstrates the ConcatenateAllSources(CxList, bool) method.
The input source code is:
Expand source
void main() { int a = 1; int b = 2; }
The source code that uses CxList.ConcatenateAllSources method:
Expand source
CxList a = All.FindByShortName("a").FindByType(typeof(Declarator)); CxList b = All.FindByShortName("b").FindByType(typeof(Declarator)); CxList main = All.FindByShortName("main"); CxList list = a + b; result = list.ConcatenateAllSources(list, false);
The result would be:
Expand source
2 flow found: [a] -> [main] [b] -> [main]
Supported from 7.1.2
Concatenates the node in list to each node in this instance. Concatenation is node-to-node (doesn't support connecting flows).
Note: Currently is identical to calling ConcatenateAllSources with testFlow = false
Expand source
public CxList ConcatenateAllSources (CxList list)
list A CxList. It will be concatenated to each node in this instance.
Flows that starts with this instance nodes, and end with the list parameter node.
ArgumentNullException : Thrown when parameter is a null reference.
If the list parameter contains more than one node or contains flows or this instance contains flows, the function return value is undefined.
The number of the returned items is same as the number of items in this instance.
This function calls the Concatenate function for each item in this instance with list as parameter.
This example demonstrates the ConcatenateAllSources(CxList) method.
The input source code is:
Expand source
void main() { int a = 1; int b = 2; }
The source code that uses CxList.ConcatenateAllSources method:
Expand source
CxList a = All.FindByShortName("a").FindByType(typeof(Declarator)); CxList b = All.FindByShortName("b").FindByType(typeof(Declarator)); CxList main = All.FindByShortName("main"); CxList list = a + b; result = list.ConcatenateAllSources(list);
The result would be:
Expand source
2 flow found: [a] -> [main] [b] -> [main]
Supported from 7.1.2
ConcatenateAllTargets
Concatenates each node in list to the node in this instance. Concatenation is node-to-node (doesn't support connecting flows).
Expand source
public CxList ConcatenateAllTargets (CxList list, bool testFlow)
list A CxList. It will be concatenated to each node in this instance.
testFlow If this parameter true -> test possible flow , otherwise connect directly.
Flows that starts with this instance nodes, and end with the list parameter node.
ArgumentNullException : Thrown when parameter is a null reference.
1. If the this instance contains more than one node or contains flows or list contains flows, the function return value is undefined.
2. The number of the returned items is same as the number of items in list.
3. This function calls the Concatenate function for this instance with each item in list as parameter.
This example demonstrate the ConcatenateAllTargets(CxList, bool) method.
The input source code is:
Expand source
void main() { int a = 1; int b = 2; }
The source code that uses CxList.ConcatenateAllTargets method:
Expand source
CxList a = All.FindByShortName("a").FindByType(typeof(Declarator)); CxList b = All.FindByShortName("b").FindByType(typeof(Declarator)); CxList main = All.FindByShortName("main"); CxList list = a + b; result = list.ConcatenateAllTargets(list, false);
The result would be:
Expand source
2 flow found: [main] -> [a] [main] -> [b]
Supported from 7.1.2
Concatenates each node in list to the node in this instance. Concatenation is node-to-node (doesn't support connecting flows).
Note: Currently is identical to calling ConcatenateAllTargets with testFlow = false
Expand source
public CxList ConcatenateAllTargets (CxList list)
list A CxList. It will be concatenated to each node in this instance.
Flows that starts with this instance nodes, and end with the list parameter node.
ArgumentNullException : Thrown when parameter is a null reference.
If the this instance contains more than one node or contains flows or list contains flows, the function return value is undefined.
The number of the returned items is same as the number of items in list.
This function calls the Concatenate function for this instance with each item in list as parameter.
This example demonstrates the ConcatenateAllTargets(CxList) method.
The input source code is:
Expand source
void main() { int a = 1; int b = 2; }
The source code that uses CxList.ConcatenateAllTargets method:
Expand source
CxList a = All.FindByShortName("a").FindByType(typeof(Declarator)); CxList b = All.FindByShortName("b").FindByType(typeof(Declarator)); CxList main = All.FindByShortName("main"); CxList list = a + b; result = list.ConcatenateAllTargets(list);
The result would be:
Expand source
2 flow found: [main] -> [a] [main] -> [b]
Supported from 7.1.2
ConcatenatePath
Concatenates two flows into one connected flow.
Expand source
public CxList ConcatenatePath (CxList secondList, bool _testFlow)
secondList A CxList containing one flow only. This flow will be concatenated to this instance.
testFlow If true, searches for a flow between this instance and list. Otherwise, connects the two flows directly (more efficient).
A flow that starts with this instance flow, and ends with the list parameter flow.
ArgumentNullException : Thrown when parameter is a null reference.
Both this instance and list have to contain only one flow (or one node as a private case), otherwise return value is undefined.
This example demonstrates the ConcatenatePath(CxList, bool) method.
The input source code is:
Expand source
void main() { int a = 1; int b = 2; }
The source code that uses CxList.ConcatenatePath method:
Expand source
CxList one = All.FindByName("1"); CxList a = All.FindByShortName("a").FindByType(typeof(Declarator)); //Declarator is a new type defined in cXQL CxList flow1 = a.InfluencedBy(one); // [1] -> [a] CxList two = All.FindByName("2"); CxList b = All.FindByShortName("b").FindByType(typeof(Declarator)); CxList flow2 = b.InfluencedBy(two); // [2] -> [b] result = flow2.ConcatenatePath(flow1, false);
The result would be:
Expand source
1 flow found: [2] -> [b] -> [1] -> [a]
Supported from 7.1.2
Concatenates two flows into one connected flow.
Expand source
public CxList ConcatenatePath (CxList secondList)
secondList A CxList containing one flow only. This flow will be concatenated to this instance.
A flow that starts with this instance flow, and ends with the list parameter flow.
ArgumentNullException : Thrown when parameter is a null reference.
This function calls CxList.ConcatenatePath(list, true).
Both this instance and list have to contain only one flow (or one node as a private case), otherwise return value is undefined.
Supported from 7.1.2
Contained
Returns a subset of “this” instance whose elements are contained in the given list, filtered according to the given nodes type.
Expand source
public CxList Contained (CxList pathList, GetStartEndNodesType requestedType)
pathList The list where the method looks for the requested node type.
requestedType An enum matching the relevant GetStartEndNodes types, which are: EndNodesOnly, StartNodesOnly, StartAndEndNodes, AllNodes and AllButNotStartAndEnd.
A subset of “this” instance with elements from the requested nodes type.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrates the CxList.Contained(CxList, GetStartEndNodesType) method.
The input source code is:
Expand source
void foo() { int b = 2, a = 5, c; if (a > b) b = 3; c = b; }
The source code that uses CxList.Contained method:
Expand source
result = All.FindByShortName("b").Contained(All.InfluencedBy(All.FindById(50)), GetStartEndNodesType.AllNodes); //Id 50 is "3" in "b = 3;"
The result would consist of 2 items:
Expand source
b (from b = 3;) b (from c = b;)
The source code that uses CxList.Contained method:
Expand source
result = All.FindByShortName("a").Contained(All.InfluencedBy(All.FindById(50)), GetStartEndNodesType.EndNodesOnly); // Id 50 is "3" in "b = 3;"
The result would consist of 0 items.
Supported from 7.1.2
Contains
return true if input parameter is root of at least one graph.
key None
None
ControlInfluencedBy
Returns a ICxListProvider which is a subset of this instance and its elements are control influenced by the specified ICxListProvider.
list ICxListProvider control-influencing on this instance.
currAlg Influence algorithm to use. An enum matching the relevant options which are: OldAlgorithm, NewAlgorithm
A subset of this instance control influenced by the specified ICxListProvider.
Returns a ICxListProvider which is a subset of this instance and its elements are control influenced by the specified ICxListProvider.
list ICxListProvider control-influencing on this instance.
A subset of this instance control influenced by the specified ICxListProvider.
ControlInfluencedByAndNotSanitized
Returns a ICxListProvider which is a subset of this instance and its elements are control influenced by the specified ICxListProvider and not sanitized by the specified ICxListProvider.
red ICxListProvider control-influencing on this instance.
green ICxListProvider of sanitizations.
currAlg Influence algorithm to use. An enum matching the relevant options which are: OldAlgorithm, NewAlgorithm
A subset of this instance control influenced by the specified ICxListProvider and not sanitized.
Returns a ICxListProvider which is a subset of this instance and its elements are control influenced by the specified ICxListProvider and not sanitized by the specified ICxListProvider.
red ICxListProvider control-influencing on this instance.
green ICxListProvider of sanitizations.
A subset of this instance control influenced by the specified ICxListProvider and not sanitized.
ControlInfluencingOn
Returns a ICxListProvider which is a subset of this instance and its elements are control influencing on the specified ICxListProvider.
list ICxListProvider control-influenced by this instance.
currAlg Influence algorithm to use. An enum matching the relevant options which are: OldAlgorithm, NewAlgorithm
A subset of this instance control influencing on the specified ICxListProvider.
Returns a ICxListProvider which is a subset of this instance and its elements are control influencing on the specified ICxListProvider.
list ICxListProvider control-influenced by this instance.
A subset of this instance control influencing on the specified ICxListProvider.
ControlInfluencingOnAndNotSanitized
Returns a ICxListProvider which is a subset of this instance and its elements are control influenced by the specified ICxListProvider and not sanitized by the specified ICxListProvider.
red ICxListProvider control-influenced by this instance.
green ICxListProvider of sanitization.
A subset of this instance control influencing on the specified ICxListProvider and not sanitized.
Count
return number of paths in current node
return number of paths
CreateXmlNode
Return a CxList composed by CxXmlNode.
Expand source
public CxList CreateXmlNodes(XPathNavigator input, CxXmlDoc xmlDoc, int language, bool save, int depth = 1)
input Provides a cursor model for navigating XML data.
xmlDoc Document where the node will be created.
language Id of the language.
save Sprecifies if the node should be saved.
depth Deapth of search. Default value is 1.
Return a CxList with CxXmlNodes for the given XPath.
Save option set to true is deprecated since 9.4.0.
None
Expand source
// create an XPathDocument object XPathDocument xmlPathDoc = new XPathDocument(xmlFileName); // create a navigator for the xpath doc XPathNavigator xNav = xmlPathDoc.CreateNavigator(); result = cxXPath.CreateXmlNodes(xNav, xmlDoc, 1, false, 1); // Returns a CxList of nodes in a given CxXmlDoc.
Supported from 8.6.0
CxListImpl
This partial class is a container for methods related to MemberAccess operations.
CxSelectDomProperty
Returns a new CxList that includes selected property that exists in DOM type <T> and define by lambda. We can achieve the same effect by using another (old) interface.
Expand source
public CxList CxSelectDomProperty<T>(Func<T,IGraph> lambda) where T:CSharpGraph
T Dom object type that this method get property from it
lambda Method that define require DOM property.
New list of requested properties.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
These examples demonstrate using of CxList.CxSelectDomProperty(Func<T, IGraph>) method.
Get all TrueStatements of type IfStmt and Statements of IterationStmt:
Expand source
CxList False = Find_Always_False(); var cond = False.GetFathers(); var falseOfIf = cond.CxSelectDomProperty<IfStmt>(x => x.TrueStatements); var falseOfIteration = cond.CxSelectDomProperty<IterationStmt>(x => x.Statements); var falseBlock = falseOfIf + falseOfIteration;
Get some data based on "Left" property of AssignExpr:
Expand source
CxList curNodes = assignsExpr.CxSelectDomProperty<AssignExpr>(x =>x.Left); left.Add(All.GetByAncs(curNodes));
Supported from v9.2.0
CxSelectElementValues
Returns list of requires property values of dom object of <TDomObject> and return list of <TOutput>. For more details see example.Main purpose of this method is hide internal CxList structure(data).
Expand source
public List<TOutput> CxSelectElementValues<TDomObject,TOutput> (Func<TDomObject,TOutput> lambda) where TDomObject:CSharpGraph
TDomObject
TOutput
lambda Method that define property to extract from require dom object.
New List of all required values.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrates using of CxList.CxSelectElementValues(Func<TDomObject,TOutput>) method.
Compare parameter name of two methods (implementation and declaration) If name of parameters are different add to result method declaration and method implementation
(query “R16_04_Different_Identifiers_In_Function_Definition_And_Prototype” CPP Misra):
Expand source
var curListNames = curParams.CxSelectElementValues<ParamDecl, string>(x => x.Name); var compListNames = compParams.CxSelectElementValues<ParamDecl, string>(x => x.Name); for (int i = 0; i<curListNames.Count; i++) { if (String.Compare(curListNames[i], compListNames[i]) != 0) { result.Add(curMethodDecl + compMethodDecl); break; } }
Supported from v9.2.0
CxSelectElements
Returns a new CxList of all required elements of input CxList. Main purpose of interface is hide internal DOM and CxList structures.
Expand source
public CxList CxSelectElements<T>(Func<T,IGraph> lambda, int option) where T:CSharpGraph
T Dom object type that this method get property from it
lambda Method that define require DOM property.
option If option is -1 → iterate on all possible elements.
If option is 0 → return only first element.
New list of requested properties.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
These examples demonstrate using of CxList.CxSelectElements(Func<T, IGraph>, int) method.
Get first element of Indices of input CxList (query "Value_Shadowing" C# medium):
Expand source
CxList variables = All.FindByType(typeof(IndexerRef)); CxList problematic = variables.FindByTypes(new string[] {"Request","HttpRequest"}); result = problematic.CxSelectElements<IndexerRef>(x=>x.Indices,0);
query Find_Array_Indexes (GO, general):
Expand source
CxList arraysOrSlices = Find_IndexerRefs(); result = arraysOrSlices.CxSelectElements<IndexerRef>(x=>x.Indices);
Supported from v9.2.0
DataInfluencedBy
Returns a CxList which is a subset of this instance and its elements are data influenced by the CxList specified in the first parameter using the influence algorithm specified in the second parameter.
Expand source
public CxList DataInfluencedBy(CxList influencing, InfluenceAlgorithmCalculation algorithm)
list CxList data-influencing on “this” instance.
currAlg An enum matching the relevant InfluenceAlgorithmCalculation options which are: OldAlgorithm , NewAlgorithm.
A subset of “this” instance data influenced by the specified CxList.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrates the CxList.DataInfluencedBy(CxList, InfluenceAlgorithmCalculation) method.
The input source code is:
Expand source
int b, a = 5; if (a > 3) b = a;
The source code that uses CxList.DataInfluencedBy method:
Expand source
CxList five = All.FindByName("5"); result = All.DataInfluencedBy(five);
The result would be:
Expand source
6 items found: a (in a = 5), a (in a > 3), > (in a > 3), a (in b = a), = (in b = a), b (in b = a)
Returns a CxList which is a subset of “this” instance and its elements are data influenced by the CxList specified in parameter.
This call is equivalent to the following calls and it is recommended to use the short call format by default:
DataInfluencedBy(list, InfluenceAlgorithmCalculation.OldAlgorithm)
Expand source
public CxList DataInfluencedBy(CxList influencing)
list CxList data-influencing on “this” instance.
A subset of “this” instance data influenced by the specified CxList.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrates the CxList.DataInfluencedBy(CxList) method.
The input source code is:
Expand source
int b, a = 5; if (a > 3) b = a;
The source code that uses CxList.DataInfluencedBy method:
Expand source
CxList five = All.FindByName("5"); result = All.DataInfluencedBy(five);
The result would be:
Expand source
6 items found: a (in a = 5), a (in a > 3), > (in a > 3), a (in b = a), = (in b = a), b (in b = a)
DataInfluencingOn
Returns a CxList which is a subset of “this” instance and its elements are data influencing on the CxList specified in the first parameter using the influence algorithm specified in the second parameter.
Expand source
public CxList DataInfluencingOn(CxList influenced, InfluenceAlgorithmCalculation algorithm)
list CxList data-influenced by “this” instance.
currAlg An enum matching the relevant InfluenceAlgorithmCalculation options which are: OldAlgorithm , NewAlgorithm.
A subset of “this” instance data influencing on the specified CxList.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrate the CxList.DataInfluencingOn(CxList, CxList.InfluenceAlgorithmCalculation) method.
The input source code is:
Expand source
int b, a = 5; if (a > 3) b = a;
The source code that uses CxList.DataInfluencingOn method:
Expand source
CxList b = All.FindByName("*.b"); result = All.DataInfluencingOn(b);
The result would be:
Expand source
3 items found: a (in b = a), a (in a = 5), 5 (in a = 5)
Returns a CxList which is a subset of “this” instance and its elements are data influencing on the CxList specified in parameter.
This call is equivalent to the following calls and it is recommended to use the short call format by default:
DataInfluencingOn(list, InfluenceAlgorithmCalculation.OldAlgorithm)
Expand source
public CxList DataInfluencingOn(CxList influenced)
list CxList data-influenced by “this” instance.
A subset of “this” instance data influencing on the specified CxList.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrate the CxList.DataInfluencingOn(CxList, InfluenceAlgorithmCalculation) method.
The input source code is:
Expand source
int b, a = 5; if (a > 3) b = a;
The source code that uses CxList.DataInfluencingOn method:
Expand source
CxList b = All.FindByName("*.b"); result = All.DataInfluencingOn(b, CxList.InfluenceAlgorithmCalculation.NewAlgorithm);
The result would be:
Expand source
3 items found: a (in b = a), a (in a = 5), 5 (in a = 5)
DefinitionNotNullOrEmpty
Checks if a given definition is not null or empty
graphDefinition None
None
DoNotSearchInComments
Replaces comments of specific languages with spaces, distinguishing between the language comment types.
curFile The name of the file to process
fileStr The contents of the file
The contents of the file with comments replaced with white spaces
ElementsIgnoreCase
Gets the elements with the specified System.Xml.Linq.XName.
element The element.
name The System.Xml.Linq.XName to match.
ignoreCase If set to true case will be ignored whilst searching for the System.Xml.Linq.XElement.
A System.Xml.Linq.XElement that matches the specified System.Xml.Linq.XName, or null.
ExtractFromSOQL
Extracts the parameters of the given keyword from a SOQL statement into a list.
Expand source
public List<string> ExtractFromSOQL(string keyword)
keyword The SOQL keyword to extract.
A list with the parameters of the keyword.
This example demonstrates the CxList.ExtractFromSOQL() method.
The input source code is:
Expand source
int b = 0; String a = "select* from table where x=" + b; List <String> result = All.ExtractFromSOQL("select");
The source code that uses CxList.ExtractFromSOQL() method:
Expand source
result = All.CallingMethodOfAny(All.FindByName ("oo"));
The result would consist of 1 item:
Expand source
["*"]
Extracts the parameters of a SOQL statement into a dictionary.
Expand source
public Dictionary<String, List<String>> ExtractFromSOQL()
A dictionary with keys that match SOQL keywords and their relevant parameters.
This example demonstrates the CxList.ExtractFromSOQL() method.
The input source code is:
Expand source
int b = 0; String a = "select* from table where x=" + b; Dictionary <String, List<String>> result = All.ExtractFromSOQL();
The source code that uses CxList.ExtractFromSOQL() method:
Expand source
result = All.CallingMethodOfAny(All.FindByName ("oo"));
The result would consist of 3 results:
Expand source
{ "select" : "*", "from" : "table", "where" : "x=" }
FillGraphsList
Fill graphs from one root element.
Expand source
public void FillGraphsList (CSharpGraph graphRoots)
graphRoot CSharpGraph instance to be filled with Graphs.
None.
ArgumentNullException : parameter is a null reference
This example demonstrates the CxList. FillGraphsList () method.
With any Input source code, the method can be called after a Query.
Expand source
first=All.GetFirstGraph(); FillGraphsList(first);
At this point, the first is filled with the Graphs.
Filter
This method implements a new filter. It returns subset of “this”. It is very similar to “Where” of LINQ.
Expand source
public CxList Filter(Func<DOMProperties, bool> condition);
condition Lambda method that define filter condition.
A subset of "this" instance, with elements that fulfilled lambda condition in the given CxList.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
These examples demonstrate using of CxList.Filter(Func<DOMProperties, bool>) method.
Get all Dom object with short name less than 50 characters:
Expand source
result = tempResult.Filter(x => x.ShortName.Length < 50);
This example demonstrates using of the CxList.Filter() and GetDOMPropertiesOfFirst methods.
Expand source
CxList sanitizers = All.NewCxList(); // Get all the elements that appear only after the position (line) of the header foreach(CxList header in good_content_header_methods) { int HeaderLineNumber = header.GetDOMPropertiesOfFirst().Line; CxList methods_after_header = possible_sanitizers.GetByAncs(header.GetFathers().GetFathers()); sanitizers.Add(methods_after_header.Filter(x => x.Line >= HeaderLineNumber)); }
Supported from v9.2.0
FilterByDomProperty
Returns a CxList which is a subset of “this” instance, with elements that match input lambda method.
Expand source
public CxList FilterByDomProperty<T>(Func<T, bool> condition) where T: CSharpGraph;
T Node element type
condition Lambda method that define/implement require condition.
A subset of “this” instance, with elements that fulfilled lambda condition in the given CxList.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrates using of CxList.FilterByDomProperty<(Func<T, bool>) method.
Get all AssignExpr with operator equal to AdditionAssign:
Expand source
CxList assignAdd = assignments. FilterByDomProperty<AssignExpr>(x =>x.Operator == AssignOperator.AdditionAssign);
Supported from v9.2.0
FilterPlugins
Returns a CxList which is a subset of “this” instance, with elements that are objects from CxEngine Plugins removed.
Expand source
public CxList FilterPlugins(void);
A subset of "this" instance, with elements that are declared in the CxEngine plugins removed.
The return value may be empty (Count = 0).
These examples demonstrate using of CxList.FilterPlugins() method.
Consider processing C code
Expand source
Void main(void) { Void* ptr = malloc(sizeof(int)); return 0; }
The source code that uses CxList.FilterPlugins method:
Expand source
CxList mallocDef = All.FindByType<MethodDecl>().FindByName("malloc"); // this list contains one node, the definition of malloc in stdlib.h CxList localMallocDef = mallocDef.FilterPlugin(); // this list would be empty
Supported from v9.5.1
FindAllMembers
Returns a ICxListProvider which is a subset of this instance, with elements that are members of the declarations in the specified ICxListProvider.
declarationsList List of declarations from which the members we want to find
A subset of this instance, with elements that are members of declarationsList.
Returns a CxList which is a subset of this instance, with elements that are members of the declarations in the specified CxList.
targetList List were the members will be found
declarationsList List of declarations from which the members we want to find
None
FindAllReferences
Returns a CxList which is a subset of “this” instance, with elements that are references of the given CxList, excluding elements in the second CxList.
Expand source
public CxList FindAllReferences(CxList ids, CxList exclude)
ids The CxList whose references are to be found.
exclude The CxList whose elements will be ignored and excluded.
A subset of “this” instance, with elements that are references of the given CxList.
ArgumentNullException : parameter is a null reference
The return value may be empty (Count = 0).
This example demonstrates the CxList.FindAllReferences() method.
The input source code is:
Expand source
int b, a = 5; if (a > 3) b = a;
The source code that uses CxList.FindAllReferences method:
Expand source
result = All.FindAllReferences(All.FindById(36), All.FindById(30)); //a in (a = 5), b in (int b)
The result would consist of 3 items:
Expand source
a (in a = 5) a (in a > 5) a (in b = a)
Returns a CxList which is a subset of “this” instance, with elements that are references of the given CxList.
Expand source
public abstract CxList FindAllReferences(CxList Ids)
ids The CxList whose references are to be found.
A subset of “this” instance, with elements that are references of the given CxList.
The return value may be empty (Count = 0).
This example demonstrates the CxList.FindAllReferences() method.
The input source code is:
Expand source
int b, a = 5; if (a > 3) b = a;
The source code that uses CxList.FindAllReferences method:
Expand source
result = All.FindAllReferences(All.FindById(36)); //a in (a = 5)
The result would consist of 3 items;
Expand source
a (in a = 5), a (in a > 5), a (in b = a)
Returns a ICxListProvider which is a subset of this instance, with elements that are references of the specified ICxListProvider.
ids Id of the object whose references to be found.
useOld Whether or not to use the old, less efficient algorithm. Used for comparing performance purposes only.
A subset of this instance, with elements that are references of the specified ICxListProvider.
FindByAbstractValue
Returns a CxList which is a subset of this instance whose elements have an abstract value that fulfills the criterion.
Expand source
public CxList FindByAbstractValue (Func<IAbstractValue, bool> criterion)
criterion Lambda method that can filter required items from this CxList according to their abstract value. This function have one parameter of type IAbstractValue and returns bool.
A subset of this instance whose elements have match the requested criterion.
These examples demonstrate the CxList.FindByAbstractValue(Func<IAbstractValue, bool>) method.
Find all DOM elements whose abstract value is an integer inside the interval 0,10:
Expand source
IAbstractValue intervalZeroToTen = new IntegerIntervalAbstractValue(0,10); CxList res = All.FindByAbstractValue(abstractValue => abstractValue.IncludedIn(intervalZeroToTen, true));
Find all DOM elements for which the integer 0 in inside their abstract value:
Expand source
IAbstractValue zero = new IntegerIntervalAbstractValue(0); CxList res = All.FindByAbstractValue(abstractValue => zero.IncludedIn(abstractValue));
Find all DOM elements whose abstract value has a given type:
Expand source
CxList res = All.FindByAbstractValue(abstractValue => abstractValue is StringAbstractValue);
The input source code is:
Expand source
int counter = 0; int x = counter + 5; string str = "a"; string secondStr = str + "b";
The source code that uses CxList.FindByAbstractValue method:
Expand source
CxList a = All. FindByAbstractValue(abstractValue => abstractValue is IntegerIntervalAbstractValue); CxList b = All.FindByAbstractValue(abstractValue => abstractValue is StringAbstractValue); result = a; // result now holds 0, '+', 'counter' and 5 in int counter = 0; int x = counter + 5; result = b; // result now holds "a", '+', 'str' and "b" in string str = "a"; string secondStr = str + "b";
The input source code is:
Expand source
var y; int counter = 0; int x = counter + 5; y();
The source code that uses CxList.FindByAbstractValue method:
Expand source
IAbstractValue zeroAbsValue = new IntegerIntervalAbstractValue(0); result = All. FindByAbstractValue(abstractValue => zeroAbsValue.IncludedIn(abstractValue)); /* result now holds 0 and 'counter' in int x = counter + 5; And also contains y in: (because y has AnyAbstractValue which includes 0) y(); */ result = All. FindIncludedAbstractValue(abstractValue => zeroAbsValue.IncludedIn(abstractValue,true)); // result now holds 0 and 'counter' in int x = counter + 5; // it does not contain y because we asked for strictTypeMatch
The input source code is:
Expand source
int counter = 0; int x = counter + 5; function foo() { } // void method foo();
The source code that uses CxList.FindByAbstractValue method:
Expand source
IAbstractValue zeroToFiveAbsValue = new IntegerIntervalAbstractValue(0,5); result = All.FindIncludedInAbstractValue(abstractValue => abstractValue.IncludedIn(zeroToFiveAbsValue)); /* result now holds 0, 5, 'counter' and the sum (counter + 5) in int counter = 0; int x = counter + 5; And also contains foo in: foo(); // because y has AnyAbstractValue which includes [0,5] */ result = All.FindIncludedInAbstractValue(abstractValue => abstractValue.IncludedIn(zeroToFiveAbsValue, true)); // result now holds 0, 5, 'counter' and the sum of (counter + 5) in int x = counter + 5; // it does not contain foo because we asked for strictTypeMatch
Supported from version 8.6.0
FindByAbstractValues
Returns a CxList which is a subset of this instance whose elements have an abstract value equal to the abstract value of one element in the sources CxList.
Expand source
public CxList FindByAbstractValues(CxList sources)
sources A CxList.
A subset of this instance whose elements have an abstract value equal to the abstract value of one element in the sources CxList.
These examples demonstrate the CxList.FindByAbstractValue(CxList) method.
The input source code is:
Expand source
string str = "a"; string secondStr = str + "b";
The source code that uses CxList.FindByAbstractValues method:
Expand source
result = All.FindByAbstractValues(All.FindByType(typeof(StringLiteral))); // result now holds "a", 'str' and "b" in string str = "a"; string secondStr = str + "b";
Supported from version 8.4.2
FindByAssignmentSide
Returns a ICxListProvider which is a subset of this instance and its elements are being on the specified side of an assignment expression.
side side of the assignment expression.
A subset of this instance on the specified side of an assignment expression.
FindByCustomAttribute
Returns a ICxListProvider which is a subset of this instance and its elements are custom attributes of the specified name.
name The attribute name.
A subset of this instance with custom attributes of the specified name.
FindByExactMemberAccess
Returns a ICxListProvider which is a subset of this instance and its elements are the ones which their specified member is accessed.
Expand source
public CxList FindByExactMemberAccess(string Name)
name Contains both the name of the type and the name of the accessed member in the qualified notation (eg. "CheckBoxList.SelectedValue").
A subset of “this” instance where its elements are the ones which their given member is accessed.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
These examples demonstrate CxList.FindByExactMemberAccess(string) method.
The input source code is:
Expand source
MyClass a; int b; a.DataMember = 3; b = a.Method();
The source code that uses CxList.FindByExactMemberAccess method:
Expand source
result = All.FindByExactMemberAccess("MyClass.DataMember");
Notice that the result would consist of 1 item:
Expand source
a.DataMember (in a.DataMember = 3)
Expand source
result = All.FindByExactMemberAccess("Class.DataMember");
The result would consist of 0 items, because "Class" is not equal to "MyClass".
Supported from 8.2.0
Receives a qualified notation string (e.g. "T.M") where T is a type name and M is a member name.
Returns a CxList which is a subset of "this" instance containing the elements with name equal to M and belong to a type whose name equals T.This search allows both case-sensitive and non case-sensitive searches.
Expand source
public CxList FindByExactMemberAccess(string Name, bool caseSensitive)
name Contains both the name of the type and the name of the accessed member in qualified notation (eg. "CheckBoxList.SelectedValue").
caseSensitive Boolean which indicates to the search to be (or not) case sensitive.
A subset of “this” instance where its elements are the ones which their specified member is accessed.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
These examples demonstrate the CxList.FindByExactMemberAccess(string, bool) method.
The input source code is:
Expand source
MyClass a; int b; a.DataMember = 3; b = a.Method();
The source code that uses CxList.FindByExactMemberAccess method:
Expand source
result = All.FindByExactMemberAccess("MyClass.dataMember", true);
Notice that the result would consist of 0 items because the search is case-sensitive.
Expand source
result = All.FindByExactMemberAccess("MyClass.dataMember", false);
The result would consist of 1 item:
Expand source
a.DataMember (in a.DataMember = 3)
Expand source
result = All.FindByExactMemberAccess("Class.dataMember", false);
The result would consist of 0 items, because “Class” is not equals to “MyClass”.
Supported from 8.2.0
Returns a CxList which is a subset of “this” instance containing the elements with name equal to the string in second parameter belong to a type whose name equals the string in the first parameter.This is a case-sensitive search by parameters.
For a non case-sensitive search, use the FindByExactMemberAccess Method(string, string, bool) instead.
Expand source
public CxList FindByExactMemberAccess(string TargetTypeName, string MemberName)
targetTypeName Contains the name of the accessed type (eg. "CheckBoxList").
memberName Contains the name of the accessed member (eg. "SelectedValue").
A subset of “this” instance where its elements are the ones which their specified member is accessed.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
These examples demonstrate the CxList.FindByExactMemberAccess(string, string) method.
The input source code is:
Expand source
MyClass a; int b; a.DataMember = 3; b = a.Method();
The source code that uses CxList.FindByExactMemberAccess method:
Expand source
result = All.FindByExactMemberAccess("MyClass", "DataMember");
Notice that the result would consist of 1 item:
Expand source
a.DataMember (in a.DataMember = 3)
Expand source
result = All.FindByExactMemberAccess("Class", "DataMember");
The result would consist of 0 items, because "Class" is not equals to "MyClass".
Supported from 8.2.0
Returns a CxList which is a subset of “this” instance containing the elements with name equal to the string in second parameter and belong to a type whose name equals the string in the first parameter.This search allows both case-sensitive and non case-sensitive searches by both parameters.
Expand source
public CxList FindByExactMemberAccess(string TargetTypeName, string MemberName, bool CaseSensitive)
targetTypeName Contains the name of the accessed type (eg. "CheckBoxList").
memberName Contains the name of the accessed member (eg. "SelectedValue").
caseSensitive Boolean which indicates to the search to be (or not) case sensitive.
A subset of “this” instance where its elements are the ones which their specified member is accessed.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
These examples demonstrate the CxList.FindByExactMemberAccess(string, string, bool) method.
The input source code is:
Expand source
MyClass a; int b; a.DataMember = 3; b = a.Method();
The source code that uses CxList.FindByExactMemberAccess method:
Expand source
result = All.FindByExactMemberAccess("MyClass", "dataMember", true);
Notice that the result would consist of 0 item because it is a case-sensitive search.
Expand source
result = All.FindByExactMemberAccess("MyClass", "dataMember", false);
The result would consist of 1 item:
Expand source
a.DataMember (in a.DataMember = 3)
Expand source
result = All.FindByExactMemberAccess("Class", "dataMember", false);
The result would consist of 0 items, because "Class" is not equals to "MyClass".
Supported from 8.2.0
FindByExactMemberAccesses
Returns a CxList which is a subset of "this" instance with nodes that are part of a member/target pair (typical example: target.member) and have a direct member in the CxList parameter "members". Returns a CxList which is a subset of this instance and its elements are the ones which their specified members and targets are accessed. It only works if all the Members are mutual for all the Targets (Types) e.g. myClass1.method1(); myClass1.method2(); myClass2.method1(); myClass2.method2();
targets Contains the name of the accessed target types
members Contains the name of the accessed members
caseSensitive None
A subset of this instance and its elements are the ones which their specified members and targets are accessed
Returns a CxList which is a subset of this instance and its elements are the ones which their specified members and a single target accessed. It only works if all the Members are mutual for all the Targets (Types) e.g. myClass1.method1(); myClass1.method2(); myClass1.method3();
target Contains the name of the accessed target
members Contains the name of the accessed members
caseSensitive None
A subset of this instance and its elements are the ones which their specified members and a single target accessed
Returns a CxListImpl which is a subset of this instance and its elements are MemberAccesses specified on the parameter <paramref name="names"></paramref>.
names A list of strings that contain both the name of the type and the name of the accessed member in qualified notation (eg. "CheckBoxList.SelectedValue"). Prefix and suffix wild card * are permitted.
caseSensitive Boolean which indicates to the search to be (or not) case sensitive.
A subset of this instance where its elements are the ones which their specified member is accessed.
FindByExtendedType
Returns a CxList which is a subset of "this" instance and the type of its elements match the type specified as parameter.
Expand source
public CxList FindByExtendedType (string extendedType)
extendedType The extended type of the objects to be found. Prefix and postfix wildcard * are supported.
A subset of "this" instance and its elements are those with type specified by the parameter.
ArgumentException : parameter is a null reference
The return may be empty (Count = 0).
This example demonstrates the CxList.FindByExtendedType() method.
The input source code is:
Expand source
MyClass a; MyClassExtended b; int c; a.DataMember = 3; c = a.Method();
The source code that uses CxList.FindByExtendedType method:
Expand source
result = All.FindByExtendedType("MyClass*");
The result would consists of 4 items:
Expand source
a (in MyClass a) b (in MyClassExtended b) a (in a.DataMember = 3) a (in c = a.Method())
FindByFathers
Returns a ICxListProvider which is a subset of this instance and its elements are those that their CxDOM are in the specified ICxListProvider
fathers CxDOM Fathers.
A subset of this instance and its elements are those that their CxDOM-Fathers are in the specified ICxListProvider.
FindByFieldAttributes
Returns a ICxListProvider which is a subset of this instance and its elements are modified by the modifier (private, external, etc).
attributes Attribute of the fields to be found.
A subset of this instance and its elements are those with attribute attrib.
FindByFileId
Returns a ICxListProvider which is a subset of this instance and its elements are in a given source code file.
fileId Id of the file
A subset of this instance in a given file name.
FindByFileName
Returns a ICxListProvider which is a subset of this instance and its elements are in a given source code file.
fileName String of the file name.
A subset of this instance in a given file name.
FindByFileNames
Returns a CxList, which is a subset of “this” instance and its elements are in a given array of source code files.
Expand source
public CxList FindByFileNames(params string[] FileNames)
fileNames Parameters with file names.
A subset of "this” instance with elements from a given list of file names.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrates the CxList.FindByFileNames(string[]) method.
Expand source
//file myCode1.cs class Cl1 { void foo() { int i; } } //file myCode2.cs class Cl2 { void bar() { int i; } }
The source code that uses CxList.FindByFileNames method:
Expand source
result = All.FindByFileNames("*myCode1.cs", "*myCode2.cs");
The result would consist of 10 items:
Expand source
Cl1 void, foo, int, i, Cl2 void, bar, int, i
Supported from 9.4
FindByFiles
Returns a subset of 'this' instance, where its DOM objects are on the same file(s) as the DOM objects in the 'source' CxList.
Expand source
public CxList FindByFiles(CxList source)
source A CxList that have DOM objects in the required files.
Returns Return a subset of 'this' instance, where its DOM objects are on the same file(s) as the DOM objects in the 'source' CxList.
ArgumentNullException : Thrown when parameter is a null reference.
This example demonstrates the CxList.FindByFiles(CxList) method.
Expand source
CxList a = All.FindByFileName("*method.js*"); CxList b = All.FindByFileName("*method.json"); result = a.FindByFiles(b); // Return a subset of 'a' where the objects are of the same file as the objects of b.
Supported from 8.4.0
FindById
Finds all objects with the specified id. This method is mainly used to find all the uses of a code element (e.g. variable, class).
Expand source
public CxList FindById (int id)
nodeId id number to be found.
A subset of "this" instance and its elements that have the specified id number.
ArgumentNullException : parameter is a null reference
The return value may be empty(Count = 0).
This example demonstrates the CxList.FindById() method.
The input source code is:
Expand source
a = 3; b = 4; if (a == 4) b = a - 1;
The source code that uses CxList.FindById method:
Expand source
result = All.FindById(60);
The result would be -
Expand source
1 item found: b ( in b = a - 1)
Finds an object with the specified ids.
nodeIds Passes as parameters directly the id numbers.
A subset of this instance with the specified id numbers.
FindByInitialization
Returns a CxList which is a subset of “this” instance and contains elements initialized by the given CxList.
Expand source
public CxList FindByInitialization(CxList InitializersList)
initializationList A CxList with initializers to search in “this” instance.
A subset of “this” instance containing declarators initialized by the specified CxList.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrate the CxList.FindByInitialization(CxList) method.
The input source code is:
Expand source
int b = 5;
The source code that uses CxList.FindByInitialization method:
Expand source
CxList declarators = All.FindByType(typeof(Declarator)); result= declarators.FindByInitialization(All);
The result would consist of 1 item:
Expand source
b
Supported from v1.8.1
FindByLanguage
Returns a CxList which is a subset of “this” instance whose elements are from the given language.
Expand source
public CxList FindByLanguage (string languageName)
languageName Language name to search.
A subset of “this” instance whose elements are from the given language.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrates the CxList.FindByLanguage(CxList) method.
The input source code is:
Expand source
//file myCode.cs class myCode { } //file MyCode.java class MyCode { }
The source code that uses CxList.FindByLanguage method:
Expand source
result = All.FindByLanguage ("Java");
The result would consist of 1 item:
Expand source
myCode (class MyCode)
FindByMemberAccess
Receives a qualified notation string (e.g. “T.M”) where T is a type name and M is a member name.
Returns a CxList which is a subset of “this” instance containing the elements with name equal to M and belong to a type whose name ends with T.This search allows both case-sensitive and non case-sensitive searches.
For a search by exact target type name, use FindByExactMemberAccess(string) instead.
Expand source
public CxList FindByMemberAccess(string Name, bool CaseSensitive = true)
name Contains both the name of the type and the name of the accessed member in qualified notation (eg. "CheckBoxList.SelectedValue"). Prefix and suffix wild card* are permitted.
caseSensitive Boolean which indicates to the search to be (or not) case sensitive.
targetComparisonType StringComparison approach
A subset of “this” instance where its elements are the ones which their specified member is accessed.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
These examples demonstrate the CxList.FindByMemberAccess(string, bool) method.
The input source code is:
Expand source
MyClass a; int b; a.DataMember = 3; b = a.Method();
The source code that uses CxList.FindByMemberAccess method:
Expand source
result = All.FindByMemberAccess("MyClass.dataMember", true);
Notice that the result would consist of 0 items because the search is case-sensitive.
Expand source
result = All.FindByMemberAccess("Class.dataMember", false);
Notice that the result would consist of 1 item:
Expand source
a.DataMember (in a.DataMember = 3)
This is so, because MyClass ends with Class.
Expand source
result = All.FindByMemberAccess("MyClass.met*", true);
Notice that the result would consist of 0 items because the search is case-sensitive.
Expand source
result = All.FindByMemberAccess("Class.dataMember", false);
Notice that the result would consist of 1 item:
Expand source
a.Method(in b = a.Method())
Supported from 1.8.1
Returns a CxList which is a subset of “this” instance containing the elements with name equal to the string in the second parameter and belong to a type whose name ends with the string in the first parameter. This is a case-sensitive search by both parameters.
Expand source
public CxList FindByMemberAccess(string TargetTypeName, string MemberName, bool CaseSensitive = true)
targetTypeName Contains the name of the accessed type (eg. "CheckBoxList").
memberName Contains the name of the accessed member (eg. "SelectedValue").
caseSensitive Boolean which indicates to the search to be (or not) case sensitive.
targetComparisonType StringComparison approach
A subset of “this” instance where its elements are the ones which their specified member is accessed.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
These examples demonstrate the CxList.FindByMemberAccess(string, string, bool) method.
The input source code is:
Expand source
MyClass a; int b; a.DataMember = 3; b = a.Method();
The source code that uses CxList.FindByMemberAccess method:
Expand source
result = All.FindByMemberAccess("MyClass", "dataMember", true);
Notice that the result would consist of 0 items because the search is case-sensitive.
Expand source
result = All.FindByMemberAccess("MyClass", "dataMember", false);
The result would consist of 1 item:
Expand source
a.DataMember (in a.DataMember = 3)
This is so, because MyClass ends with Class.
Expand source
result = All.FindByMemberAccess("MyClass", "met*", true);
Notice that the result would consist of 0 items because the search is case-sensitive.
Expand source
result = All.FindByMemberAccess("MyClass", "met*", false);
the result would consist of 1 item:
Expand source
a.Method(in b = a.Method())
FindByMemberAccesses
Receives an array of qualified notation strings (e.g. “T.M”) where T is a type name and M is a member name.
Returns a CxList which is a subset of “this” instance containing the elements with name equal to M and belong to a type whose name ends with T, for any strings in the array. This search allows both case-sensitive and non case-sensitive searches.
Expand source
public CxList FindByMemberAccesses(string [] Names, bool CaseSensitive = true)
names An array of strings where each contains both the name of the type and the name of the accessed member in qualified notation (eg. "CheckBoxList.SelectedValue"). Prefix and suffix wild card* are permitted.
caseSensitive Boolean which indicates to the search to be (or not) case sensitive. This Boolean is true by default.
targetComparisonType StringComparison approach
A subset of “this” instance where its elements are the ones which their specified members are accessed.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
These examples demonstrate the CxList.FindByMemberAccesses(string, bool) method.
The input source code is:
Expand source
MyClass a; int b; a.DataMember = 3; b = a.Method();
The source code that uses CxList.FindByMemberAccesses method:
Expand source
string []memberAccesses = new string[]{“Class.dataMember”, “MyClass.Met*”} result = All.FindByMemberAccesses(memberAccesses, true);
Notice that the result would consist of 1 item because the search is case-sensitive:
Expand source
a.Method()
Expand source
result = All.FindByMemberAccesses(memberAccesses);
The result would consist of 2 items:
Expand source
a.DataMember a.Method
This is so, because MyClass ends with Class.
Supported from 8.2.0
Receives a qualified notation string (e.g. “T.M”) where T is a type name and M is a member name.
Returns a CxList which is a subset of “this” instance containing the elements with name equal to M and belong to a type whose name equals T.This is a case sensitive search.
For a non case-sensitive search, use the FindByExactMemberAccess (string, bool) instead. Returns a CxList which is a subset of this instance and its elements are the ones which their specified members and targets are accessed. It only works if all the Members are mutual for all the Targets (Types) e.g. myClass1.method1(); myClass1.method2(); myClass2.method1(); myClass2.method2();
targets Contains the name of the accessed target types
members Contains the name of the accessed members
caseSensitive Whether to perform case-sensitive search (defaults to true)
targetComparisonType StringComparison approach (defaults to StringComparison.OrdinalIgnoreCase)
A subset of this instance and its elements are the ones which their specified members and targets are accessed
Returns a CxList which is a subset of this instance and its elements are the ones which their specified members and a single target accessed. It only works if all the Members are mutual for all the Targets (Types) e.g. myClass1.method1(); myClass1.method2(); myClass1.method3();
target Contains the name of the accessed target
members Contains the name of the accessed members
caseSensitive Whether to perform case-sensitive search (defaults to true)
targetComparisonType StringComparison approach (defaults to StringComparison.OrdinalIgnoreCase)
A subset of this instance and its elements are the ones which their specified members and a single target accessed
FindByMethodReturnType
Returns a CxList which is a subset of “this” instance and its elements are method declarators of a given return type.
Expand source
public CxList FindByMethodReturnType(string type)
type The return type name string.
A subset of “this” instance with method declarators of a given return type.
ArgumentNullException : Thrown when parameter is a null reference.
1. If the this instance contains more than one node or contains flows or list contains flows, the function return value is undefined.
2. The number of the returned items is same as the number of items in list.
3. This function calls the Concatenate function for this instance with each item in list as parameter.
This example demonstrate the CxList.FindByMethodReturnType(string) method.
The input source code is:
Expand source
MyType foo() { ... }
The source code that uses CxList.FindByMethodReturnType method:
Expand source
result = All.FindByMethodReturnType("MyType");
The result would consist of 1 item:
Expand source
foo
FindByName
Returns a CxList which is a subset of “this” instance and its elements are the ones which their name is the given parameter(optionally with wildcards) and is not shorter than minLength and not longer than maxLength.
Expand source
public CxList FindByName(string nodeName, int minLength, int maxLength)
nodeName Contains the name of the objects. Prefix and postfix wildcard * are supported.
minLength Minimum length of the searched strings.
maxLength Maximum length of the searched strings.
A subset of “this” instance and its elements are the ones which their name is the given parameter, according to the given length interval.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrate the CxList.FindByName(string, int, int) method.
The input source code is:
Expand source
MyClass a; int b; a.DataMember = 3; b = a.Method();
The source code that uses CxList.FindByName method:
Expand source
result = All.FindByName("*Me*", 3, 7);
The result would consist of 1 item:
Expand source
Method (in b = a.Method())
Supported from v2.0.5
Returns a CxList which is a subset of “this” instance and its elements are the ones which their names are equal to the given list.
Expand source
public CxList FindByName(CxList nodesList)
nodesList The list of nodes containing the names to be found.
A subset of “this” instance and its elements are the ones which the name is contained in the given list.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrate the CxList.FindByName(CxList) method.
The input source code is:
Expand source
MyClass a; int b; a.DataMember = 3; b = a.Method();
The source code that uses CxList.FindByName method:
Expand source
result = All.FindByName(All.FindByType(typeof(MemberAccess)));
The result would consist of 3 items:
Expand source
a (in MyClass a) a (in a.DataMember = 3) a (in b = a.Method())
Returns a CxList which is a subset of “this” instance and its elements are the ones which their names are equal to the list given.
Expand source
public CxList FindByName(CxList nodesList, bool CaseSensitive)
nodesList The list of nodes containing the names to be found.
caseSensitive Boolean which indicates to the search to be (or not) case sensitive.
A subset of “this” instance and its elements are the ones which their name is contained in the given list, according to the specified case sensitivity comparison criteria.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrate the CxList.FindByName(CxList, bool) method.
The input source code is:
Expand source
MyClass A; int a; A.DataMember = 3; a = A.Method();
The source code that uses CxList.FindByName method:
Expand source
result = All.FindByName(All.FindByType(typeof(MemberAccess)), false));
The result would consist of 5 items:
Expand source
A (in MyClass A) a (in int a) A (in A.DataMember = 3) a (in a = A.Method()) A (in a = A.Method())
Returns a CxList which is a subset of “this” instance and its elements are the ones which their name is the given parameter.
Expand source
public CxList FindByName(string name)
nodeName The name of the objects to look for. Prefix and postfix wildcard * are supported.
A subset of “this” instance and its elements are the ones which their name is the given parameter.
ArgumentNullException : Thrown when parameter is a null reference.
1. If the this instance contains more than one node or contains flows or list contains flows, the function return value is undefined.
2. The number of the returned items is same as the number of items in list.
3. This function calls the Concatenate function for this instance with each item in list as parameter.
This example demonstrate the CxList.FindByName(string) method.
The input source code is:
Expand source
MyClass a; int b; a.DataMember = 3; b = a.Method();
The source code that uses CxList.FindByName method:
Expand source
result = All.FindByName("*Member*");
The result would consist of 1 item:
Expand source
a.DataMember (in a.DataMember = 3)
Returns a CxList which is a subset of “this” instance and its elements are the ones which their name is the given parameter, according to the specified comparison criteria.
Expand source
public CxList FindByName(string name, bool CaseSensitive)
nodeName Contains the name of the objects. Prefix and postfix wildcard * are supported.
caseSensitive Boolean which indicates to the search to be (or not) case sensitive.
A subset of “this” instance and its elements are the ones which their name is the given parameter, according to the given comparison criteria.The caseSensitive boolean value defines the ability to search using case sensitive or case insensitive comparison.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrate the CxList.FindByName(string, bool) method.
The input source code is:
Expand source
MyClass a; int b; a.DataMember = 3; b = a.Method();
The source code that uses CxList.FindByName method:
Expand source
result = All.FindByName("*member*", true);
The result would consist of 0 items.
Expand source
result = All.FindByName("*member*", false);
The result would consist of 1 item:
Expand source
a.DataMember (in a.DataMember = 3)
Supported from v1.8.1
Returns a CxList which is a subset of “this” instance and its elements are the ones which their name is the given parameter. The comparison method specified in parameter is used for matching.
Expand source
public CxList FindByName(string name, StringComparison comparisonType)
nodeName The name of the objects to look for. Prefix and postfix wildcard * are supported.
comparisonType StringComparison type to be used in name comparison. One of the following values: CurrentCulture, CurrentCultureIgnoreCase, InvariantCulture, InvariantCultureIgnoreCase, Ordinal, OrdinalIgnoreCase
A subset of “this” instance and its elements are the ones which their name is the given parameter.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrate the CxList.FindByName(string, StringComparison) method.
The input source code is:
Expand source
MyClass a; int b; a.DataMember = 3; b = a.Method();
The source code that uses CxList.FindByName method:
Expand source
result = All.FindByName("*member*", StringComparison.OrdinalIgnoreCase));
The result would consist of 1 item:
Expand source
DataMember (in a.DataMember = 3)
Supported from v1.8.1
FindByNames
Returns a CxList which is a subset of “this” instance and its elements are the ones which their names are equal to the list given.
Expand source
public CxList FindByNames(params String[] nodeNames)
nodeNames A list of strings containing the names to be found.
A subset of “this” instance and its elements are the ones which their name is contained in the given list, according to the specified case sensitivity comparison criteria. CaseSensitive is by default is true.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrate the CxList.FindByNames(params String[]) method.
The input source code is:
Expand source
MyClass A; int a; A.DataMember = 3; a = A.Method();
The source code that uses CxList.FindByNames method:
Expand source
result = All.FindByNames("*MEMBER","A.Method");
The result would consist of 3 items:
Expand source
A (in A.DataMember = 3) a (in a = A.Method()) A (in a = A.Method())
Supported from v9.4.0
Returns a CxList which is a subset of “this” instance and its elements are the ones which their names are equal to the list given.
Expand source
public CxList FindByNames(String[] nodeNames, bool CaseSensitive = true)
nodeNames A list of strings containing the names to be found.
caseSensitive Boolean which indicates to the search to be (or not) case sensitive.
A subset of “this” instance and its elements are the ones which their name is contained in the given list, according to the specified case sensitivity comparison criteria.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrate the CxList.FindByNames(String[], bool) method.
The input source code is:
Expand source
MyClass A; int a; A.DataMember = 3; a = A.Method();
The source code that uses CxList.FindByNames method:
Expand source
string[] str = new string[2] {"*MEMBER","A.Method"}; result = All.FindByNames(str);
The result would consist of 3 items:
Expand source
A (in A.DataMember = 3) a (in a = A.Method()) A (in a = A.Method())
Supported from v9.4.0
Returns a CxList which is a subset of “this” instance and its elements are the ones which their names are equal to the list given.
nodeNames A list of strings containing the names to be found.
stringComparison The StringComparison type to compare strings.
A subset of “this” instance and its elements are the ones which their name is contained in the given list, according to the specified comparison method.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
FindByNumberOfParameters
Returns a CxList which is a subset of "this" instance and its elements are nodes (method declarations, constructor, etc) with the number of parameters given.
Expand source
public CxList FindByNumberOfParameters (int numParams)
numParams int with the number of parameters.
A subset of this instance with nodes (method declarations, constructor, etc) that has the number of parameters given.
ArgumentNullException : parameter is a null reference
The return value may be empty (Count = 0).
This example demonstrates the CxList.FindByNumberOfParameters() method.
The input source code is:
Expand source
foo("myVar");
The source code that uses CxList.FindByNumberOfParameters method:
Expand source
CxList var = All.FindByShortName("myVar"); result = All.FindByNumberOfParameters(1);
the result would consist of 1 item:
foo
Supported from v9.4
FindByParameterName
Returns a CxList which is a subset of "this", containing only MethodInvokeExpr DOM node where their arguments are labeled according to the given value.
Expand source
public CxList FindByParameterName (string paramName)
paramName String containing the name of the parameter belonging to the resultant methods.
A subset of "this" instance where its elements are MethodInvokeExpr and ObjectCreateExpr and these contain arguments labelled according to 'paramName'.
ArgumentNullException : parameter is a null reference
The return value may be empty (Count = 0).
This example demonstrates the CxList. FindByParameterName(string) method.
The input source code is:
Expand source
class NamedExample { static void Main(string [] args) { PrintOrderDetails(sellerName:"Gift Shop",31,productName:"Red Mug"); } }
The source code that uses CxList.FindByParameterName method:
Expand source
result = All.FindByParameterName("sellerName");
The resul would consist in 1 item:
Expand source
PrintOrderDetails
Returns a CxList which is a subset of "this", containing only MethodInvokeExpr DOM node where their arguments on a given position are labeled according to the given value.
Expand source
public CxList FindByParameterName (string paramName, int paramPosition)
paramName String containing the name of the parameter belonging to the resultant methods.
paramPosition Zero based index indicating the position of argument named 'paramName'.
A subset of "this" instance where its elements are MethodInvokeExpr and ObjectCreateExpr and these contain arguments labelled according to 'paramName' in the indicated position by 'paramPosition'.
ArgumentNullException : parameter is a null reference
The return value may be empty (Count = 0).
This example demonstrates the CxList. FindByParameterName(string, int) method.
The input source code is;
Expand source
class NamedExample { static void Main(string [] args) { PrintOrderDetails(sellerName:"Gift Shop",31,productName:"Red Mug"); } }
The source code that uses CxList.FindByParameterName method:
Expand source
result = All.FindByParameterName ("sellerName", 1);
Would have no results because there's no argument named "sellerName" on the first position of the method call.
However,
Expand source
result = All.FindByParameterName ("productName", 1);
Would result in 1 item:
Expand source
PrintOrderDetails
Because there's an argument on the second position(zero based) called "productName"
FindByParameterValue
Returns a ICxListProvider which is a subset of this instance and its parameters values are as specified.
paramNo Zero-based index of the parameter.
paramValue The value of the parameter.
opr One of the following values: BinaryOperator.IdentityEquality, BinaryOperator.IdentityInequality.
useAbstractValue Boolean which indicates to use the string abstract value.
Returns a ICxListProvider which is a subset of this instance and its parameters values are as specified.
Returns a CxList which is a subset of “this” instance and its elements are methods whose parameters values (referred by their index) are equal(or not).
Expand source
public CxList FindByParameterValue(int paramNo1, int paramNo2, BinaryOperator opr)
paramNo1 Zero-based index of the parameter.
paramNo2 Zero-based index of the parameter.
opr One of the followings: BinaryOperator.IdentityEquality, BinaryOperator.IdentityInequality.
A subset of “this” instance whose parameter values are equal or not equal (depending on the operator chosen).
ArgumentNullException : parameter is a null reference
The return value may be empty (Count = 0).
This example demonstrates the CxList.GetParameters() method.
The input source code is:
Expand source
foo(1, i, 1);
The source code that uses CxList.FindByParameterValue method:
Expand source
CxList methods = All.FindByType(typeof(MethodInvokeExpr)); result = All.FindByParameterValue(0, 2, BinaryOperator.IdentityEquality);
the result would consist of 1 item:
Expand source
foo (first parameter value is equal to the third one)
FindByParameters
Returns a CxList which is a subset of “this” instance and its elements are methods of the given CxList with the specified parameters.
Expand source
public CxList FindByParameters (CxList paramList)
paramList CxList of method parameters.
A subset of "this" instance with methods whose parameters are given in the list.
ArgumentNullException : parameter is a null reference
The return value may be empty (Count = 0).
This example demonstrates the CxList.FindByParameters() method.
The input source code is:
Expand source
foo("myVar");
The source code that uses CxList.FindByParameters method:
Expand source
CxList var = All.FindByShortName("myVar"); result = All.FindByParameters(var);
The result would consist of 1 item:
Expand source
foo
FindByPointerType
Returns a CxList which is a subset of “this” instance and its elements are of the type pointer of the specified type of code element.
Expand source
public CxList FindByPointerType(string type, int maxDepth, bool caseSensitive)
type type of the parameter
maxLevels Zero-based maximum depth to look for. Default value is 0 meaning that it will look all the PointerTypeRef levels until it finds a TypeRef
caseSensitive Default value is true
A subset of "this" instance and its elements are of the type pointer of the specified type of code element.
ArgumentNullException : type is a null reference
The return value may be empty (Count = 0).
This example demonstrates the CxList.FindByPointerType method.
The input source code is:
Expand source
var i *int
The source code that uses CxList.FindByPointerType method:
Expand source
result = All.FindByPointerType(“int”);
the result would consist of 2 items:
Expand source
*int – pointer i – Declarator
Supported from v8.5.0
Returns a CxList which is a subset of “this” instance and its elements are of the type pointer of the specified type of code element
Expand source
public CxList FindByPointerType(string type, bool caseSensitive)
type type of the parameter
caseSensitive Default value is true
A subset of "this" instance and its elements are of the type pointer of the specified type of code element.
ArgumentNullException : type is a null reference
The return value may be empty (Count = 0).
This example demonstrates the CxList.FindByPointerType method.
The input source code is:
Expand source
var i *int
The source code that uses CxList.FindByPointerType method:
Expand source
result = All.FindByPointerType("int");
the result would consist of 2 items:
Expand source
*int - pointer I - Declarator
Supported from v8.5.0
FindByPointerTypes
Returns a CxList which is a subset of "this" instance and its elements are of the type pointer of the specified types of code element
Expand source
public CxList FindByPointerType(string[] type, bool caseSensitive)
types types of the parameter
caseSensitive Default value is true
A subset of "this" instance and its elements are of the type pointer of the specified types of code element.
ArgumentNullException : type is a null reference
The return value may be empty (Count = 0).
This example demonstrates the CxList.FindByPointerType method.
The input source code is:
Expand source
var i * int
The source code that uses CxList.FindByPointerType method:
Expand source
result = All.FindByPointerTypes(new string[]{"int","string"});
the result would consist of 2 items:
Expand source
*int - pointer i - Declarator
Supported from v8.5.0
Returns a CxList which is a subset of "this" instance and its elements are of the type pointer of the specified types of code element
Expand source
public CxList FindByPointerTypes(string[] type, int maxLevels, bool caseSensitive)
type types of the parameter
maxLevels Zero-based maximum depth to look for. Default value is 0 meaning that it will look all the PointerTypeRef levels until it finds a TypeRef
caseSensitive Default value is true
A subset of “this” instance and its elements are of the type pointer of the specified types of code element.
ArgumentNullException : type is a null reference
The return value may be empty (Count = 0).
This example demonstrates the CxList.FindByPointerTypes method.
The input source code is:
Expand source
var i *int
The source code that uses CxList.FindByPointerTypes method:
Expand source
result = All.FindByPointerTypes(new string[]{"int","string"});
the result would consist of 2 items:
Expand source
*int - pointer i - Declarator
Supported from v8.5.0
FindByPosition
Returns a CxList which is a subset of "this" instance and its elements are located in the given file and line number.
Expand source
public CxList FindByPosition(string file, int line)
file File name in the source code.
line Line number in the source code.
A subset of "this" instance which is located in the given file and line.
ArgumentNullException : parameter is a null reference
The return value may be empty (Count = 0).
This example demonstrates the CxList.FindByPosition() method.
The input source code is (file name "MyCode.java"):
Expand source
MyClass a; int b; a.DataMember = 5; b = a.Method();
The source code that uses CxList.FindByPosition method:
Expand source
result = All.FindByPosition (“MyCode.java”, 3);
the result would consist of 1 item:
Expand source
5 (in a.DataMember = 5)
Returns a CxList which is a subset of “this” instance and its elements are located in the given file, line and column.
Expand source
public CxList FindByPosition(string file, int line, int col)
file File name in the source code.
line Line number in the source code.
col Column number in the source code.
A subset of "this" instance which is located in the specified file, line and column.
ArgumentNullException : parameter is a null reference
The return value may be empty (Count = 0).
This example demonstrates the CxList.FindByPosition() method.file name "MyCode.java"
The input source code is:
Expand source
MyClass a; int b; a.DataMember = 5; b = a.Method();
Expand source
result = All.FindByPosition (“MyCode.java”, 3, 16);
the result would be -
Expand source
1 item found: 5 (in a.DataMember = 5)
Returns a CxList which is a subset of "this" instance and its elements are in the given line number.
Expand source
public CxList FindByPosition(int line)
line The line number.
A subset of "this" instance with elements from the given line.
ArgumentNullException : parameter is a null reference
The return value may be empty (Count = 0).
This example demonstrates the CxList. FindByPosition() method.
The input source code is:
Expand source
int b, a = 5; if (a > 3) b = 6;
The source code that uses CxList.FindByPosition method:
Expand source
result = All.FindByPosition(2);
the result would consist of 4 items:
if
a,
>,
3
Returns a CxList which is a subset of “this” instance and its elements are located in the given line and column number.
Expand source
public CxList FindByPosition(int line, int col)
line Line number in the source code.
col Column number in the source code.
A subset of "this" instance with elements from the specified line and column.
ArgumentNullException : parameter is a null reference
The return value may be empty (Count = 0).
This example demonstrates the CxList.FindByPosition() method.
The input source code is:
Expand source
MyClass a; int b; a.DataMember = 3; b = a.Method();
The source code that uses CxList.FindByPosition method:
Expand source
result = All.FindByPosition (3, 16);
the result would consist of 1 item:
Expand source
3 (in a.DataMember = 3)
Returns a CxList which is a subset of “this” instance and its elements are in the given line/column and with the given length.
Expand source
public CxList FindByPosition(int line, int col, int length)
line The line number.
col The column number.
length The element length.
A subset of "this" instance with elements from the specified line, column and with the given length.
ArgumentNullException : parameter is a null reference
The return value may be empty (Count = 0).
This example demonstrates the CxList. FindByPosition() method.
The input source code is:
Expand source
int b, a = 5; if (a==33) b = 6;
The source code that uses CxList.FindByPosition method:
Expand source
result = All.FindByPosition(2, 5, 1);
the result would consist of 1 item:
Expand source
a
FindByPositions
Finds the elements of "this" instance at positions given in the pragmas list.
Expand source
public CxList FindByPositions(SortedList pragmas, int extendMatch, bool oneOnly)
pragmas A sorted list containing the pragmas to match.
extendMatch Defines the closeness of the matching results: 0 => ExactMatch: find exact match 1 => FindInLine: extend search to objects in closest position within same line 2 => FindClosestMatch: extend match to closest position within the same file
oneOnly If true, it returns one result per position.
The elements from "this" instance that are at the required positions.
ArgumentNullException : First parameter is a null reference
The return value may be empty (Count = 0).
This example demonstrates the CxList.FindByPositions() method.
The input source code is:
Expand source
int b, a = 5; if (a == 33) b = 6;
The source code that uses CxList.FindByPositions method:
Expand source
CxList list = All.FindByShortName("b"); SortedList sorted = new SortedList(new PragmaComparer()); foreach (KeyValuePair<int, IGraph> dic in list.data) { sorted.Add(dic.Value.LinePragma, null); } result = All.FindByPositions(sorted, 1, true);
the result would consist of 2 items:
Expand source
b (in int b) b (in b = 6)
Finds the elements of "this" instance at positions given in the list using the proximity given in parameter.
positions A list containing the pragmas to match.
extendMatchEnum Defines the closeness of the matching results. One of the following values: ExactMatch: find exact match FindInLine: extend search to objects in closest position within same line FindClosestMatch: extend match to closest position within the same file
oneOnly If true, it returns one result per position.
The elements of "this" instance that are at the given positions.
ArgumentNullException : First parameter is a null reference
The return value may be empty (Count = 0).
This example demonstrates the CxList. FindByPositions() method.
The input source code is:
Expand source
int b, a = 5; if (a == 33) b = 6;
The source code that uses CxList.FindByPositions method:
Expand source
CxList list = All.FindByName(“b”); result = All.FindByPositions(list, CxPositionProximity.FindInLine, false);
The result would be all the elements in the 5 lines closer to lines that appear variable b:
Expand source
2 items found b (in int b) b (in b = 6)
Finds the elements of “this” instance at positions given in the pragmas list using the proximity from the parameter.
Expand source
public CxList FindByPositions(SortedList<LinePragma,object> pragmas, CxPositionProximity extendMatch, bool oneOnly)
pragmas A list containing the pragmas to match.
extendMatchEnum Defines the closeness of the matching results. One of the following values: ExactMatch: find exact match FindInLine: extend search to objects in closest position within same line FindClosestMatch: extend match to closest position within the same file
oneOnly If true, it returns one result per position.
The elements from the current instance that are at the required positions.
ArgumentNullException : parameter is a null reference
The return value may be empty (Count = 0).
This example demonstrates the CxList. FindByPositions() method.
The input source code is:
Expand source
int b, a = 5; if (a == 33) b = 6;
The source code that uses CxList.FindByPositions method:
Expand source
CxList list = All.FindByName(“b”); SortedList<LinePragma, object> sorted = new SortedList<LinePragma, object>(new DataCollections.PragmaComparer()); foreach (KeyValuePair<int, IGraph> dic in list.data) { sorted.Add(dic.Value.LinePragma, null); } result = All.FindByPositions(sorted, CxList.CxPositionProximity.FindInLine, true);
The result would consist of 2 items:
Expand source
b (in int b) b (in b = 6)
Finds the elements of “this” instance at positions given in the pragmas list using the proximity given in parameter.
pragmas A list containing the pragmas to match.
extendMatchEnum Defines the closeness of the matching results. One of the following values: ExactMatch: find exact match FindInLine: extend search to objects in closest position within same line FindClosestMatch: extend match to closest position within the same file
oneOnly If true, it returns one result per position.
farLines Acceptable line distance to look for (the default setting is 5).
The elements from "this" instance that are at the given positions.
ArgumentNullException : parameter is a null reference
The return value may be empty (Count = 0).
This example demonstrates the CxList. FindByPositions() method.
The input source code is:
Expand source
int b, a = 5; if (a == 33) b = 6;
The source code that uses CxList.FindByPositions method:
Expand source
CxList list = All.FindByName(“b”); SortedList<LinePragma, object> sorted = new SortedList<LinePragma, object>(new DataCollections.PragmaComparer()); foreach (KeyValuePair<int, IGraph> dic in list.data) { sorted.Add(dic.Value.LinePragma, null); } result = All.FindByPositions(sorted, CxList.CxPositionProximity.FindInLine, true, 5);
The result would consist of 2 items:
Expand source
b (in int b) b (in b = 6)
Finds the elements of "this" instance at lines of files given in parameter.
Expand source
public CxList FindByPositions(List<KeyValuePair<int, string>> lines)
lines A list of pairs line/filename to search the elements.
The subset of elements from “this” instance that are in the files given at the lines requested.
ArgumentNullException : parameter is a null reference
The return value may be empty (Count = 0).
This example demonstrates the CxList. FindByPositions() method.
The input source code is (file name is “MyCode.cs”:
Expand source
int b, a = 5; if (a == 33) b = 6;
The source code that uses CxList.FindByPositions method:
Expand source
KeyValuePair<int,string> position = new KeyValuePair<int, string>(3,”path\\MyCode.cs”); List<KeyValuePair<int, string>> list = new List<KeyValuePair<int, string>>(); list.Add(position); result = All.FindByPositions(list);
The result would consist of 3 items:
Expand source
b = 6
FindByRegex
return DOM objects by searching the source file with regex
expression the regex search string
cxOptions an enum matching the relevant CxRegexOptions which are: SearchInComments, DoNotSearchInStringLiterals, AllowOverlaps and SearchOnlyInComments
regularOptions options to add to the regular expression (case sensitivity, etc.)
extendedResults is filled with the strings of the matches
farLines The line distance to look for matches in comments (the default is 5).
None
Returns a CxList which is a subset of this instance and its elements match the specified regular expression string. This call is equivalent to the following calls and it is recommended to use the short call format by default:
FindByRegex(expression, null)
FindByRegex(expression, CxRegexOptions.None)
FindByRegex(expression, CxRegexOptions.None, RegexOptions.None)
FindByRegex(expression, CxRegexOptions.None, RegexOptions.None, null)
FindByRegex(expression, CxRegexOptions.None, RegexOptions.None, null, 5)
FindByRegex(expression, false, true, false)
FindByRegex(expression, false, true, false, null)
FindByRegex(expression, CxRegexOptions.None, null)
Expand source
public CxList FindByRegex(string expression)
expression Regular expression string.
A subset of this instance matches the given regular expression.
ArgumentNullException : parameter is a null reference
The return value may be empty (Count = 0)
The following code example shows how you can use the FindByRegex method.
This example demonstrates the CxList.FindByRegex() method.
The input source code is:
Expand source
int a = 5; if (a > 3) foo(a);
The source code that uses CxList.FindByRegex method:
Expand source
result = All.FindByRegex(@"(\s)?foo\(");
The result would be -
Expand source
1 item found: foo
Supported from: CxAudit v1.8.1
Returns a CxList which is a subset of this instance and its elements match the specified regular expression string, according to specified flag parameters. This call is equivalent to the following calls and it is highly recommended to use the enum instead of the confusing flags:
FindByRegex(expression, searchInComments, searchInStringLiterals, recursive, null)
The 3 flags are translated to CxRegexOptions enum in the following way(bitmask supported) :
(false, false, false) => CxRegexOptions.DoNotSearchInStringLiterals
(false, false, true) => CxRegexOptions.DoNotSearchInStringLiterals | CxRegexOptions.AllowOverlaps
(false, true, false) => CxRegexOptions.None
(false, true, true) => CxRegexOptions.AllowOverlaps
(true, false, false) => CxRegexOptions.SearchInComments | CxRegexOptions.DoNotSearchInStringLiterals
(true, false, true) => CxRegexOptions.SearchInComments | CxRegexOptions.DoNotSearchInStringLiterals | CxRegexOptions.AllowOverlaps
(true, true, false) => CxRegexOptions.SearchInComments
(true, true, true) => CxRegexOptions.SearchInComments | CxRegexOptions.AllowOverlaps
After translating the flags to CxRegexOptions enum this call is equivalent to the following calls:
FindByRegex(expression, cxRegexOptions)
FindByRegex(expression, cxRegexOptions, RegexOptions.None)
FindByRegex(expression, cxRegexOptions, RegexOptions.None, null)
FindByRegex(expression, cxRegexOptions, RegexOptions.None, null, 5)
FindByRegex(expression, cxRegexOptions, null)
Expand source
public CxList FindByRegex(string expression , bool searchInComments, bool searchInStringLiterals, bool recursive)
expression Regular expression string.
searchInComments Positive if searching inside comments is desired.
searchInStringLiterals Positive if searching inside comments is desired.
recursive Positive if searching inside comments is desired.
A subset of this instance matches the given regular expression according to the additional parameters.
The return value may be empty (Count = 0).
The following code example shows how you can use the FindByRegex method.
This example demonstrates the CxList.FindByRegex() method.
The input source code is:
Expand source
int a = 5; if (a > 3) foo(a);
The source code that uses CxList.FindByRegex method:
Expand source
result = All.FindByRegex(@"(\s)?foo\(", false, true, false);
The result would be -
Expand source
1 item found: foo
Supported from v1.8.1
Returns a CxList which is a subset of this instance and its elements match the specified regular expression string, according to specified Checkmarx Regex Options defined in the second parameter.
This call is equivalent to the following calls and it is recommended to use the short call format by default:
FindByRegex(expression, cxRegexOptions, RegexOptions.None)
FindByRegex(expression, cxRegexOptions, RegexOptions.None, null)
FindByRegex(expression, cxRegexOptions, RegexOptions.None, null, 5)
FindByRegex(expression, cxRegexOptions, null)
Expand source
public CxList FindByRegex(string expression , CxRegexOptions cxOptions)
expression Regular expression string.
cxOptions An enum matching the relevant CxRegexOptions which are: None, SearchInComments, DoNotSearchInStringLiterals, AllowOverlaps and SearchOnlyInComments
A subset of this instance matches the given regular expression according to the additional parameters.
ArgumentNullException : Expression parameter is a null reference
The return value may be empty (Count = 0).
The following code example shows how you can use the FindByRegex method.
This example demonstrates the CxList.FindByRegex() method.
The input source code is:
Expand source
int a = 5; if (a > 3) foo(a);
The source code that uses CxList.FindByRegex method:
Expand source
result = All.FindByRegex(@"(\s)?foo\(", CxList.CxRegexOptions.None);
The result would be -
Expand source
1 item found: foo
Supported from v1.8.1
Returns a CxList which is a subset of this instance and its elements match the specified regular expression string, according to specified Regex Options defined in the parameters(Checkmarx regex options and standard regex options).
This call is equivalent to the following calls and it is recommended to use the short call format by default:
FindByRegex(expression, cxRegexOptions, regexOptions, null)
FindByRegex(expression, cxRegexOptions, regexOptions, null, 5)
Expand source
public CxList FindByRegex(string expression , CxRegexOptions cxOptions, RegexOptions regularOptions)
expression Regular expression string.
cxOptions An enum matching the relevant CxRegexOptions which are: None, SearchInComments, DoNotSearchInStringLiterals, AllowOverlaps and SearchOnlyInComments
regularOptions Options to add to the regular expression (case sensitivity, etc.) In addition to the user-defined regular-expression-options in this arguments, the algorithm also uses the following regex-options by default: RegexOptions.Multiline, RegexOptions.Singleline.
A subset of this instance matches the given regular expression according to the additional parameters.
ArgumentNullException : Expression parameter is a null reference
The return value may be empty (Count = 0).
The following code example shows how you can use the FindByRegex method.
This example demonstrates the CxList.FindByRegex() method.
The input source code is:
Expand source
int a = 5; if (a > 3) foo(a)
The source code that uses CxList.FindByRegex method:
Expand source
result = All.FindByRegex(@"(\s)?foo\(", CxList.CxRegexOptions.None, System.Text.RegularExpressions.RegexOptions.None);
The result would be -
Expand source
1 item found: foo
Supported from v1.8.1
Returns a CxList which is a subset of this instance and its elements match the specified regular expression string, and fill the extended results parameter with the strings of the matches.
This query search source files with regex, and return the closest same line DOM object to the matches. If no such object exists, returns the closest object in a successive line.
Search does not include searching inside comments and string literals, and regex matches are not allowed to overlap. The matching strings are returned in the extendedResults parameter.
This call is equivalent to the following calls:
FindByRegex(expression, CxRegexOptions.None, RegexOptions.None, cxList)
FindByRegex(expression, CxRegexOptions.None, RegexOptions.None, cxList, 5)
FindByRegex(expression, false, true, false, cxList)
Using the Boolean flags option is not recommended, use the enums instead.
FindByRegex(expression, CxRegexOptions.None, cxList)
Expand source
public CxList FindByRegex(string expression , CxList extendedResults)
expression Regular expression string.
extendedResults extendedResults parameter is filled with the strings of the matches.
A subset of this instance matches the given regular expression according to the additional parameters.
ArgumentNullException : Expression parameter is a null reference
The return value may be empty (Count = 0).
The following code example shows how you can use the FindByRegex method.
This example demonstrates the CxList.FindByRegex() method.
The input source code is:
Expand source
int a = 5; if (a > 3) foo(a);
The source code that uses CxList.FindByRegex method:
Expand source
result = All.FindByRegex(@"(\s)?foo\(", All.NewCxList());
The result would be -
Expand source
1 item found foo
Supported from v1.8.1
Returns a CxList which is a subset of this instance and its elements match the specified regular expression string, according to specified flag parameters and fill the extended results parameter with the strings of the matches: The 3 flags are translated to CxRegexOptions enum in the following way (bitmask supported):
(false, false, false) => CxRegexOptions.DoNotSearchInStringLiterals
(false, false, true) => CxRegexOptions.DoNotSearchInStringLiterals | CxRegexOptions.AllowOverlaps
(false, true, false) => CxRegexOptions.None
(false, true, true) => CxRegexOptions.AllowOverlaps
(true, false, false) => CxRegexOptions.SearchInComments | CxRegexOptions.DoNotSearchInStringLiterals
(true, false, true) => CxRegexOptions.SearchInComments | CxRegexOptions.DoNotSearchInStringLiterals | CxRegexOptions.AllowOverlaps
(true, true, false) => CxRegexOptions.SearchInComments
(true, true, true) => CxRegexOptions.SearchInComments | CxRegexOptions.AllowOverlaps
After translating the flags to CxRegexOptions enum this call is equivalent to the following calls: (It is highly recommended to use the enum instead of the confusing flags)
FindByRegex(expression, cxRegexOptions, RegexOptions.None, cxList)
FindByRegex(expression, cxRegexOptions, RegexOptions.None, cxList, 5)
FindByRegex(expression, cxRegexOptions, cxList)
Expand source
public CxList FindByRegex(string expression , bool searchInComments, bool searchInStringLiterals, bool recursive, CxList extendedResults)
expression Regular expression string.
searchInComments Positive if searching inside comments is desired.
searchInStringLiterals Positive if searching inside string literals is desired.
recursive Positive if searching inside string literals is desired.
extendedResults Positive if searching inside string literals is desired.
A subset of this instance matches the given regular expression according to the additional parameters.
ArgumentNullException : Expression parameter is a null reference
The return value may be empty (Count = 0).
The following code example shows how you can use the FindByRegex method.
This example demonstrates the CxList.FindByRegex() method.
The input source code is:
Expand source
int a = 5; if (a > 3) foo(a);
The source code that uses CxList.FindByRegex method:
Expand source
result = All.FindByRegex(@"(\s)?foo\(", false, true, false, All.NewCxList());
The result would be -
Expand source
1 item found foo
Supported from v1.8.1
Returns a CxList which is a subset of this instance and its elements match the specified regular expression string, according to specified Checkmarx Regex Options defined in the second parameter, and also fill the extended results parameter with the strings of the matches.
This call is equivalent to the following calls and it is recommended to use the short call format by default:
FindByRegex(expression, cxRegexOptions, RegexOptions.None, cxList)
FindByRegex(expression, cxRegexOptions, RegexOptions.None, cxList, 5)
Expand source
public CxList FindByRegex(string expression , CxRegexOptions cxOptions, CxList extendedResults)
expression Regular expression string.
cxOptions An enum matching the relevant CxRegexOptions which are: None, SearchInComments, DoNotSearchInStringLiterals, AllowOverlaps and SearchOnlyInComments
extendedResults extendedResults parameter is filled with the strings of the matches.
A subset of this instance matches the given regular expression according to the additional parameters.
ArgumentNullException : Expression parameter is a null reference
The return value may be empty (Count = 0).
The following code example shows how you can use the FindByRegex method.
This example demonstrates the CxList.FindByRegex() method.
The input source code is:
Expand source
int a = 5; if (a > 3) foo(a);
The source code that uses CxList.FindByRegex method:
Expand source
result = All.FindByRegex(@"(\s)?foo\(", CxList.CxRegexOptions.None, All.NewCxList());
The result item would be -
Expand source
1 item found: foo
Supported from v1.8.1
Returns a CxList which is a subset of this instance and its elements match the specified regular expression string, according to specified Regex Options defined in the parameters(Checkmarx regex options and standard regex options), and also fill the extended results parameter with the strings of the matches. This call is equivalent to the following call and it is recommended to use the short call format by default: FindByRegex(expression, cxRegexOptions, regexOptions, cxList, 5)
Expand source
public CxList FindByRegex(string expression , CxRegexOptions cxOptions, RegexOptions regularOptions, CxList extendedResults)
expression Regular expression string.
cxOptions An enum matching the relevant CxRegexOptions which are: None, SearchInComments, DoNotSearchInStringLiterals, AllowOverlaps and SearchOnlyInComments
regularOptions Options to add to the regular expression (case sensitivity, etc.) In addition to the user-defined regular-expression-options in this arguments, the algorithm also uses the following regex-options by default: RegexOptions.Multiline, RegexOptions.Singleline.
extendedResults extendedResults parameter is filled with the strings of the matches.
A subset of this instance matches the given regular expression according to the additional parameters.
ArgumentNullException : Expression parameter is a null reference
The return value may be empty (Count = 0).
The following code example shows how you can use the FindByRegex method.
This example demonstrates the CxList.FindByRegex() method.
The input source code is:
Expand source
int a = 5; if (a > 3) foo(a);
The source code that uses CxList.FindByRegex method:
Expand source
result = All.FindByRegex(@"(\s)?foo\(", CxList.CxRegexOptions.None, System.Text.RegularExpressions.RegexOptions.None, All.NewCxList());
The result would be -
Expand source
1 item found: foo
Supported from v1.8.1
return DOM objects by searching the source file with regex
expression the regex search string
cxOptions an enum matching the relevant CxRegexOptions which are: SearchInComments, DoNotSearchInStringLiterals, AllowOverlaps and SearchOnlyInComments
regularOptions options to add to the regular expression (case sensitivity, etc.)
extendedResults is filled with the strings of the matches
farLines The line distance to look for matches in comments (the default is 5).
None
FindByRegexExt
Find in files by regular expression regardless of DOM and language
expression Regular expression pattern
regFileFilter Mask of the files to search
cxOptions Search in comments
regularOptions Options for regular expression
None
Find by regular expression in all files of the project regardless of DOM and language.
Expand source
public CxList FindByRegexExt(string pattern)
expression Regular expression pattern.
A list of matches for given regular expression in all project files.
ArgumentNullException : Thrown when parameter is a null reference.
The results are not related to DOM so they can't be compared to DOM objects returned by other functions. Results can't be used as parameters to other queries. The return value may be empty (Count = 0).
This example demonstrate the CxList.FindByRegexExt(string) method.
The input source code is:
Expand source
int a = 5; if (a > 3) foo(a); else FOO(a); // foo(a) /* foo */
The source code that uses CxList.FindByRegexExt method:
Expand source
result = All.FindByRegexExt(@"(\s)?foo");
The result would consist of 3 items:
Expand source
foo // foo /* foo
Supported from version 7.1.8 and 7.1.6HF5
Find by regular expression in all files of the project regardless of DOM and language.
Expand source
public CxList FindByRegexExt(string expression, string fileMask)
expression Regular expression pattern.
fileMask File mask for search. Control characters "*" and "?" are supported.
For example: "." looks in all files and "*.aspx" looks in aspx files.
A list of matches for given regular expression in all project files.
ArgumentNullException : Thrown when parameter is a null reference.
The results are not related to DOM so they can't be compared to DOM objects returned by other functions. Results can't be used as parameters to other queries. The return value may be empty (Count = 0).
This example demonstrate the CxList.FindByRegexExt(string, string) method.
The input source code is:
Expand source
int a = 5; if (a > 3) foo(a); else FOO(a); // foo(a) /* foo */
The source code that uses CxList.FindByRegexExt method:
Expand source
result = All.FindByRegexExt(@"(\s)?foo", “*.cs”);
The search would be only on *.cs files and the result would consist of 3 items:
Expand source
foo // foo /* foo
Supported from version 7.1.8 and 7.1.6HF5
Find by regular expression in all files of the project regardless of DOM and language.
Expand source
public CxList FindByRegexExt(string expression, string fileMask, CxRegexOptions cxOptions)
expression Regular expression pattern.
fileMask File mask for search. Control characters "*" and "?" are supported.
For example: "." looks in all files and "*.aspx" looks in aspx files.
cxOptions An enum matching the relevant CxRegexOptions which are: None , SearchInComments , DoNotSearchInStringLiterals , AllowOverlaps and SearchOnlyInComments.
A list of matches for given regular expression in cho
ArgumentNullException : Thrown when parameter is a null reference.
The results are not related to DOM so they can't be compared to DOM objects returned by other functions. Results can't be used as parameters to other queries. The return value may be empty (Count = 0). Default values relevant only from version 7.1.8.
This example demonstrate the CxList.FindByRegexExt(string, string, CxRegexOptions) method.
The input source code is:
Expand source
int a = 5; if (a > 3) foo(a); else FOO(a); // foo(a) /* foo */
The source code that uses CxList.FindByRegexExt method:
Expand source
result = All.FindByRegexExt(@"(\s)?foo", "*.*", RegexOptions.IgnoreCase);
The search would be only on *.cs files and the result would consist of 2 items:
Expand source
foo FOO
Supported from version 7.1.8 and 7.1.6HF5
Find by regular expression in all files of the project regardless of DOM and language.
Expand source
public CxList FindByRegexExt(string expression, string fileMask, bool searchInComments)
expression Regular expression pattern.
fileMask File mask for search. Control characters "*" and "?" are supported.
For example: "." looks in all files and "*.aspx" looks in aspx files.
searchInComments Allow or not search in comments.
A list of matches for given regular expression in chosen project files including or excluding results in comments.
ArgumentNullException : Thrown when parameter is a null reference.
The results are not related to DOM so they can't be compared to DOM objects returned by other functions. Results can't be used as parameters to other queries. The return value may be empty (Count = 0). Default values relevant only from version 7.1.8.
This example demonstrate the CxList.FindByRegexExt(string, string, bool) method.
The input source code is:
Expand source
int a = 5; if (a > 3) foo(a); else FOO(a); // foo(a) /* foo */
The source code that uses CxList.FindByRegexExt method:
Expand source
result = All.FindByRegexExt(@"(\s)?foo","*.cs",false);
The result would consist of 3 items:
Expand source
foo // foo /* foo
Supported from version 7.1.8 and 7.1.6HF5
Find by regular expression in all files of the project regardless of DOM and language.
Expand source
public CxList FindByRegexExt(string expression, string fileMask = "*.*", CxList.CxRegexOptions cxOptions = CxList.CxRegexOptions.None, RegexOptions regularOptions = RegexOptions.None)
expression Regular expression pattern
fileMask Mask of the files to search
cxOptions Checkmarx regex options
regularOptions Options for regular expression
A list of matches for given regular expression in chosen project files including or excluding results in comments with regex build with specified options.
ArgumentNullException : Thrown when parameter is a null reference.
The results are not related to DOM so they can't be compared to DOM objects returned by other functions. Results can't be used as parameters to other queries. The return value may be empty (Count = 0). Default values relevant only from version 7.1.8.
This example demonstrate the CxList.FindByRegexExt(string, string, CxRegexOptions, RegexOptions) method.
The input source code is:
Expand source
int a = 5; if (a > 3) foo(a); else FOO(a); // foo(a) /* foo */
The source code that uses CxList.FindByRegexExt method:
Expand source
result = All.FindByRegexExt(@"(\s)?foo", "*.*", CxRegexOptions.None, RegexOptions.IgnoreCase);
All files in the source code would be searched and the result would consist of 2 items:
Expand source
foo FOO
Supported from version 7.1.8 and 7.1.6HF5
None
Find by regular expression in all files of the project regardless of DOM and language.
Expand source
public CxList FindByRegexExt(string expression, string fileMask = "*.*", bool searchInComments = true, RegexOptions regularOptions = RegexOptions.None)
expression Regular expression pattern.
fileMask Default value: ".".
File mask for search. Control characters "*" and "?" are supported.
For example: "." looks in all files and "*.aspx" looks in aspx files.
searchInComments Default value: true.
Allow or not search in comments.
regularOptions Default value: RegexOptions.None.
Options for regular expression build from first parameter.
A list of matches for given regular expression in chosen project files including or excluding results in comments with regex build with specified options.
ArgumentNullException : Thrown when parameter is a null reference.
The results are not related to DOM so they can't be compared to DOM objects returned by other functions. Results can't be used as parameters to other queries. The return value may be empty (Count = 0). Default values relevant only from version 7.1.8.
This example demonstrate the CxList.FindByRegexExt(string, string, bool, RegexOptions) method.
The input source code is:
Expand source
int a = 5; if (a > 3) foo(a); else FOO(a); // foo(a) /* foo */
The source code that uses CxList.FindByRegexExt method:
Expand source
result = All.FindByRegexExt(@"(\s)?foo","*.*","*.json",false,RegexOptions.IgnoreCase);
All files in the source code would be searched except the *.json files and the result would consist of 2 items:
Expand source
foo FOO
Supported from version 7.1.8 and 7.1.6HF5
Find by regular expression in all files of the project regardless of DOM and language.
Expand source
public CxList FindByRegexExt(string expression, string fileMask = "*.*", bool searchInComments = true, CxList.CxRegexOptions cxOptions = CxList.CxRegexOptions.None, RegexOptions regularOptions = RegexOptions.None)
expression Regular expression pattern.
fileMask Default value: ".".
File mask for search. Control characters "*" and "?" are supported.
For example: "." looks in all files and "*.aspx" looks in aspx files.
searchInComments Default value: true.
Allow or not search in comments.
cxOptions An enum matching the relevant CxRegexOptions which are: None , SearchInComments , DoNotSearchInStringLiterals , AllowOverlaps and SearchOnlyInComments.
regularOptions Default value: RegexOptions.None.
Options for regular expression build from first parameter.
A list of matches for given regular expression in chosen project files including or excluding results in comments with regex build with specified options.
ArgumentNullException : Thrown when parameter is a null reference.
The results are not related to DOM so they can't be compared to DOM objects returned by other functions. Results can't be used as parameters to other queries. The return value may be empty (Count = 0). Default values relevant only from version 7.1.8.
This example demonstrate the CxList.FindByRegexExt(string, string, bool, CxRegexOptions, RegexOptions) method.
The input source code is:
Expand source
int a = 5; if (a > 3) foo(a); else FOO(a); // foo(a) /* foo */
The source code that uses CxList.FindByRegexExt method:
Expand source
result = All.FindByRegexExt(@"(\s)?foo","*.*",false, CxRegexOptions.None, RegexOptions.IgnoreCase);
All files in the source code would be searched and the result would consist of 2 items:
Expand source
foo FOO
Supported from version 7.1.8 and 7.1.6HF5
Find by regular expression in all files of the project regardless of DOM and language.
Expand source
public CxList FindByRegexExt(string expression, List<string> fileMaskList, bool searchInComments = true, CxList.CxRegexOptions cxOptions = CxList.CxRegexOptions.None, RegexOptions regularOptions = RegexOptions.None)
expression Regular expression pattern.
fileMaskList List of File masks for search. Control characters "*" and "?" are supported.
For example: "." looks in all files and "*.aspx" looks in aspx files.
searchInComments Default value: true.
Allow or not search in comments.
cxOptions An enum matching the relevant CxRegexOptions which are: None , SearchInComments , DoNotSearchInStringLiterals , AllowOverlaps and SearchOnlyInComments.
regularOptions Default value: RegexOptions.None.
Options for regular expression build from first parameter.
A list of matches for given regular expression in chosen project files including or excluding results in comments with regex build with specified options.
ArgumentNullException : Thrown when parameter is a null reference.
The results are not related to DOM so they can't be compared to DOM objects returned by other functions. Results can't be used as parameters to other queries. The return value may be empty (Count = 0). Default values relevant only from version 7.1.8.
This example demonstrate the CxList.FindByRegexExt(string, List<string>, bool, CxRegexOptions, RegexOptions) method.
The input source code is:
Expand source
int a = 5; if (a > 3) foo(a); else FOO(a); // foo(a) /* foo */
The source code that uses CxList.FindByRegexExt method:
Expand source
result = All.FindByRegexExt(@"(\s)?foo", new List<string>{"*.cs", "*.js"}, false, CxRegexOptions.None, RegexOptions.IgnoreCase);
Files in the source code with .cs and .js extensions would be included in the search and the result would consist of 2 items:
Expand source
foo FOO
Supported from version 8.0.0
Find by regular expression in all files of the project regardless of DOM and language.
Expand source
public CxList FindByRegexExt(string expression, string fileMask, string fileExclMask)
expression Regular expression pattern.
fileMask File mask for search. Control characters "*" and "?" are supported.
For example: "." looks in all files and "*.aspx" looks in aspx files.
fileExclMask File mask for excluding files with the specified extensions from the search. Control characters "*" and "?" are supported.
For example: If "." is used as the value for fileMask, "*.json" can be used for fileExclMask to exclude all the JSON files from the search.
A list of matches for given regular expression in all project files.
ArgumentNullException : Thrown when parameter is a null reference.
The results are not related to DOM so they can't be compared to DOM objects returned by other functions. Results can't be used as parameters to other queries. The return value may be empty (Count = 0).
This example demonstrate the CxList.FindByRegexExt(string, string, string) method.
The input source code is:
Expand source
int a = 5; if (a > 3) foo(a); else FOO(a); // foo(a) /* foo */
The source code that uses CxList.FindByRegexExt method:
Expand source
result = All.FindByRegexExt(@"(\s)?foo", ”*.*”, “*.json”);
All files in the source code would be searched except the *.json files and the result would consist of 3 items:
Expand source
foo // foo /* foo
Supported from version 9.4.5
Find by regular expression in all files of the project regardless of DOM and language.
Expand source
public CxList FindByRegexExt(string expression, string fileMask, string fileExclMask, CxRegexOptions cxOptions)
expression Regular expression pattern.
fileMask File mask for search. Control characters "*" and "?" are supported.
For example: "." looks in all files and "*.aspx" looks in aspx files.
fileExclMask File mask for excluding files with the specified extensions from the search. Control characters "*" and "?" are supported.
For example: If "." is used as the value for fileMask, "*.json" can be used for fileExclMask to exclude all the JSON files from the search.
cxOptions An enum matching the relevant CxRegexOptions which are: None , SearchInComments , DoNotSearchInStringLiterals , AllowOverlaps and SearchOnlyInComments.
A list of matches for given regular expression in chosen project files including or excluding results in comments with regex build with specified options.
ArgumentNullException : Thrown when parameter is a null reference.
The results are not related to DOM so they can't be compared to DOM objects returned by other functions. Results can't be used as parameters to other queries. The return value may be empty (Count = 0). Default values relevant only from version 9.4.5.
This example demonstrate the CxList.FindByRegexExt(string, string, string, CxRegexOptions) method.
The input source code is:
Expand source
int a = 5; if (a > 3) foo(a); else FOO(a); // foo(a) /* foo */
The source code that uses CxList.FindByRegexExt method:
Expand source
result = All.FindByRegexExt(@"(\s)?foo", ”*.*”, “*.json”, RegexOptions.IgnoreCase));
All files in the source code would be searched except the *.json files and the result would consist of 2 items:
Expand source
foo FOO
Supported from version 9.4.5
Find by regular expression in all files of the project regardless of DOM and language.
Expand source
public CxList FindByRegexExt(string expression, string fileMask, string fileExclMask, bool searchInComments)
expression Regular expression pattern.
fileMask File mask for search. Control characters "*" and "?" are supported.
For example: "." looks in all files and "*.aspx" looks in aspx files.
fileExclMask File mask for excluding files with the specified extensions from the search. Control characters "*" and "?" are supported.
searchInComments Allow or not search in comments.
A list of matches for given regular expression in chosen project files including or excluding results in comments.
ArgumentNullException : Thrown when parameter is a null reference.
The results are not related to DOM so they can't be compared to DOM objects returned by other functions. Results can't be used as parameters to other queries. The return value may be empty (Count = 0). Default values relevant only from version 9.4.5.
This example demonstrate the CxList.FindByRegexExt(string, string, string, bool) method.
The input source code is:
Expand source
int a = 5; if (a > 3) foo(a); else FOO(a); // foo(a) /* foo */
The source code that uses CxList.FindByRegexExt method:
Expand source
result = All.FindByRegexExt(@"(\s)?foo","*.*","*.json",false);
All files in the source code would be searched except the *.json files and the result would consist of 1 item:
Expand source
foo
Supported from version 9.4.5
Find by regular expression in all files of the project regardless of DOM and language.
Expand source
public CxList FindByRegexExt(string expression, string fileMask = "*.*", string fileExclMask = "", CxRegexOptions cxOptions = CxRegexOptions.None, RegexOptions regularOptions = RegexOptions.None)
expression Regular expression pattern.
fileMask Default value: ".".
File mask for search. Control characters "*" and "?" are supported.
For example: "." looks in all files and "*.aspx" looks in aspx files.
fileExclMask File mask for excluding files with the specified extensions from the search. Control characters "*" and "?" are supported.
cxOptions An enum matching the relevant CxRegexOptions which are: None , SearchInComments , DoNotSearchInStringLiterals , AllowOverlaps and SearchOnlyInComments.
regularOptions Default value: RegexOptions.None.
Options for regular expression build from first parameter.
A list of matches for given regular expression in chosen project files including or excluding results in comments with regex build with specified options.
ArgumentNullException : Thrown when parameter is a null reference.
The results are not related to DOM so they can't be compared to DOM objects returned by other functions. Results can't be used as parameters to other queries. The return value may be empty (Count = 0). Default values relevant only from version 9.4.5.
This example demonstrate the CxList.FindByRegexExt(string, string, string, CxRegexOptions, RegexOptions) method.
The input source code is:
Expand source
int a = 5; if (a > 3) foo(a); else FOO(a); // foo(a) /* foo */
The source code that uses CxList.FindByRegexExt method:
Expand source
result = All.FindByRegexExt(@"(\s)?foo","*.*","*.json",CxRegexOptions.None,RegexOptions.IgnoreCase);
All files in the source code would be searched except the *.json files and the result would consist of 2 items:
Expand source
foo FOO
Supported from version 9.4.5
None
Find by regular expression in all files of the project regardless of DOM and language.
Expand source
public CxList FindByRegexExt(string expression, string fileMask = "*.*", string fileExclMask = "", bool searchInComments = true, RegexOptions regularOptions = RegexOptions.None)
expression Regular expression pattern.
fileMask File mask for search. Control characters "*" and "?" are supported.
For example: "." looks in all files and "*.aspx" looks in aspx files.
fileExclMask File mask for excluding files with the specified extensions from the search. Control characters "*" and "?" are supported.
searchInComments Default value: true.
Allow or not search in comments.
regularOptions Default value: RegexOptions.None.
Options for regular expression build from first parameter.
A list of matches for given regular expression in chosen project files including or excluding results in comments with regex build with specified options.
ArgumentNullException : Thrown when parameter is a null reference.
The results are not related to DOM so they can't be compared to DOM objects returned by other functions. Results can't be used as parameters to other queries. The return value may be empty (Count = 0). Default values relevant only from version 9.4.5.
This example demonstrate the CxList.FindByRegexExt(string, string, string, bool, RegexOptions) method.
The input source code is:
Expand source
int a = 5; if (a > 3) foo(a); else FOO(a); // foo(a) /* foo */
The source code that uses CxList.FindByRegexExt method:
Expand source
result = All.FindByRegexExt(@"(\s)?foo","*.*","*.json",false,RegexOptions.IgnoreCase);
All files in the source code would be searched except the *.json files and the result would consist of 2 items:
Expand source
foo FOO
Supported from version 9.4.5
Find by regular expression in all files of the project regardless of DOM and language.
Expand source
public CxList FindByRegexExt(string expression, string fileMask = "*.*", string fileExclMask = "", bool searchInComments = true, CxRegexOptions cxOptions = CxRegexOptions.None, RegexOptions regularOptions = RegexOptions.None)
expression Regular expression pattern.
fileMask File mask for search. Control characters "*" and "?" are supported.
For example: "." looks in all files and "*.aspx" looks in aspx files.
fileExclMask File mask for excluding files with the specified extensions from the search. Control characters "*" and "?" are supported.
searchInComments Default value: true.
Allow or not search in comments.
cxOptions An enum matching the relevant CxRegexOptions which are: None , SearchInComments , DoNotSearchInStringLiterals , AllowOverlaps and SearchOnlyInComments.
regularOptions Default value: RegexOptions.None.
Options for regular expression build from first parameter.
A list of matches for given regular expression in chosen project files including or excluding results in comments with regex build with specified options.
ArgumentNullException : Thrown when parameter is a null reference.
The results are not related to DOM so they can't be compared to DOM objects returned by other functions. Results can't be used as parameters to other queries. The return value may be empty (Count = 0). Default values relevant only from version 9.4.5.
This example demonstrate the CxList.FindByRegexExt(string, string, string, bool, CxRegexOptions, RegexOptions) method.
The input source code is:
Expand source
int a = 5; if (a > 3) foo(a); else FOO(a); // foo(a) /* foo */
The source code that uses CxList.FindByRegexExt method:
Expand source
result = All.FindByRegexExt(@"(\s)?foo","*.*","*.json",false,CxRegexOptions.None,RegexOptions.IgnoreCase);
All files in the source code would be searched except the *.json files and the result would consist of 2 items:
Expand source
foo FOO
Supported from version 9.4.5
Find by regular expression in all files of the project regardless of DOM and language.
Expand source
public CxList FindByRegexExt(string expression, List<string> fileMaskList, List<string> fileExclMaskList, bool searchInComments = true, CxRegexOptions cxOptions = CxRegexOptions.None, RegexOptions regularOptions = RegexOptions.None)
expression Regular expression pattern.
fileMaskList List of File masks for search. Control characters "*" and "?" are supported.
For example: "." looks in all files and "*.aspx" looks in aspx files.
fileExclMaskList List of File masks for excluding files with the specified extensions from the search. Control characters "*" and "?" are supported.
searchInComments Default value: true.
Allow or not search in comments.
cxOptions An enum matching the relevant CxRegexOptions which are: None , SearchInComments , DoNotSearchInStringLiterals , AllowOverlaps and SearchOnlyInComments.
regularOptions Default value: RegexOptions.None.
Options for regular expression build from first parameter.
A list of matches for given regular expression in chosen project files including or excluding results in comments with regex build with specified options.
ArgumentNullException : Thrown when parameter is a null reference.
The results are not related to DOM so they can't be compared to DOM objects returned by other functions. Results can't be used as parameters to other queries. The return value may be empty (Count = 0). Default values relevant only from version 9.4.5.
This example demonstrate the CxList.FindByRegexExt(string, List<string>, List<string>, bool, CxRegexOptions, RegexOptions) method.
The input source code is:
Expand source
int a = 5; if (a > 3) foo(a); else FOO(a); // foo(a) /* foo */
The source code that uses CxList.FindByRegexExt method:
Expand source
result = All.FindByRegexExt(@"(\s)?foo", new List<string>{"*.cs", "*.js*"}, new List<string>{"*.json"}, false, CxRegexOptions.None, RegexOptions.IgnoreCase);
Files in the source code with .cs and .js* extensions would be included in the search, except the *.json files and the result would consist of 2 items:
Expand source
foo FOO
Supported from version 9.4.5
FindByRegexSecondOrder
Filters a CxList of Comments DOM objects according to a check of whether a Comment object contain a match to the provided regex expression, and returns closest DOM object to those that pass the filter. Used in C\C++ MISRA Preset queries in order to validate comments style.
Expand source
public CxList FindByRegexSecondOrder(string expression , CxList extendedResults)
expression Regular expression search string.
inputList The comments CxList that's should be filtered.
A subset of this instance matches the given regular expression according to the additional parameters.
ArgumentNullException : Expression parameter is a null reference
The following code example shows how you can use the FindByRegexSecondOrder method
This example demonstrates the CxList.FindByRegexSecondOrder() method.
The input source code is taken from MISRA Code_Commented_Out query:
Expand source
/* Function comment is compliant. * / void mc2_0202(void ) { use_int32(0); // Comment Not Compliant }
The source code that uses CxList.FindByRegexSecondOrder method:
Expand source
// Find all comments ending with } or ; CxList extendedResult = All.NewCxList(); // All /* */ comments CxList res = All.FindByRegex(@"/\*.*?\*/", true, false, false, extendedResult); // Search results for } or ; at end of comment result = All.FindByRegexSecondOrder(@"[;{}]\s*\*/", extendedResult);
The result will be the commented out function which is found out by this regex
Supported from v1.8.1
FindByReturnType
Returns a CxList which is a subset of this instance and its elements are of the specified type.
Expand source
public CxList FindByReturnType(String type, bool stripPointerAndRefFromReturnType = true)
type The type of the objects to be found.
stripPointerAndRefFromReturnType true – the result will include methods that return Type* as well as methods that return Type.
A subset of this instance and its elements are of the specified return type.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrate the CxList.FindByReturnType(string, bool) method.
The input source code is:
Expand source
public class a { int bla() { int b, a = 5; if (a == 33) b = 6; return b; } }
The source code that uses CxList.FindByReturnType method:
Expand source
result = All.FindByReturnType ("int");
The result would consist of 1 item:
Expand source
bla() (in int bla())
Supported from v1.8.1
FindByReturnTypes
Returns a CxList which is a subset of this instance and its elements are of the specified list of types.
Expand source
public CxList FindByReturnType(params string[] Types)
types The types of the objects to be found
A subset of this instance and its elements are of the specified return type.
ArgumentNullException : parameter is a null reference
The return value may be empty (Count = 0).
The following code example shows how you can use the FindByReturnTypes method.
This example demonstrates the CxList.FindByReturnTypes() method.
The input source code is:
Expand source
public class a { int bla() { int b, a = 5; if (a == 33) b = 6; return b; } boolean test() { return true; } }
The source code that uses CxList.FindByReturnTypes method:
Expand source
String[] types = new String[]{"int", "boolean"}; result = All.FindByReturnTypes(types);
The result would be -
Expand source
2 items found: bla() (in int bla()) test() (in boolean test())
Supported from v9.4.0
Returns a CxList which is a subset of this instance and its elements are of the specified list of types.
Expand source
public CxList FindByReturnType(String[] Types, bool stripPointerTypeRefFromReturnType = true)
types The types of the objects to be found
stripPointerTypeRefFromReturnType true – the result will include methods that return Type* as well as methods that return Type
A subset of this instance and its elements are of the specified return type.
ArgumentNullException : parameter is a null reference
parameter is a null reference
The following code example shows how you can use the FindByReturnTypes method.
This example demonstrates the CxList.FindByReturnTypes() method.
The input source code is:
Expand source
public class a { int bla() { int b, a = 5; if (a == 33) b = 6; return b; } boolean test() { return true; } }
The source code that uses CxList.FindByReturnTypes method:
Expand source
String[] types = new String[]{"int", "boolean"}; result = All.FindByReturnTypes(types);
The result would be -
Expand source
2 items found: bla() (in int bla()) test() (in boolean test())
Supported from v9.4.0
FindByShortName
Returns a CxList which is a subset of this instance and its elements are the ones which their short name is the specified string.
Expand source
public CxList FindByShortName(CxList nodesList)
nodesList The short names of the objects to look for. Prefix and postfix wildcard * are supported.
Returns a CxList which is a subset of this instance and its elements are the ones which their short name is the specified string.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrate the CxList.FindByShortName(CxList) method.
The input source code is:
Expand source
class Program { static void Main(string[] args) { Customer c = new customer(); } } class Customer { } class User { }
The source code that uses CxList.FindByShortName method:
Expand source
CxList classes = All.FindByType(typeof(ClassDecl)); CxList types = All.FindByType(typeof(TypeRef)); CxList classesWithInstances = classes - classes.FindByShortName(types);
The result would consist of 3 items.
Expand source
Customer ( in class Customer{}) Program(in class Program) User ( in class User{})
Supported from v1.8.1
Returns a CxList which is a subset of this instance and its elements are the ones which their short name is the specified string, according to the specified comparison criteria.
Expand source
public CxList FindByShortName(CxList nodesList, bool CaseSensitive)
nodesList The short names of the objects to look for. Prefix and postfix wildcard * are supported.
caseSensitive Boolean which indicates to the search to be (or not) case sensitive.
A subset of this instance and its elements are the ones which their short name is the specified string, according to the specified comparison criteria.Where the caseSensitive value can be true for case sensitive and false for case insensitive.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrate the CxList.FindByShortName(CxList, bool) method.
The input source code is:
Expand source
class Program { static void Main(string[] args) { Customer c = new customer(); } } class Customer { } class User { }
The source code that uses CxList.FindByShortName method:
Expand source
CxList classes = All.FindByType(typeof(ClassDecl)); CxList types = All.FindByType(typeof(TypeRef)); CxList classesWithInstances = classes - classes.FindByShortName(types, true);
The result would consist of 3 items.
Expand source
Customer ( in class Customer{}) Program(in class Program) User ( in class User{})
Expand source
CxList classes = All.FindByType(typeof(ClassDecl)); CxList types = All.FindByType(typeof(TypeRef)); CxList classesWithInstances = classes - classes.FindByShortName(types, false);
The result would consist of 2 items:
Expand source
Program(in class Program) User ( in class User{})
Supported from v1.8.1
Returns a CxList which is a subset of this instance and its elements are the ones which their short name is the specified string.
Expand source
public CxList FindByShortName(string nodeName)
nodeName The short name of the objects to look for. Prefix and postfix wildcard * are supported.
A subset of this instance and its elements are the ones which their name is the specified string.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrate the CxList.FindByShortName(string) method.
The input source code is:
Expand source
MyClass a; int b; a.DataMember = 3; b = a.Method();
The source code that uses CxList.FindByShortName method:
Expand source
result = All.FindByShortName("Method");
The result would consist of 1 item:
Expand source
Method ( in b = a.Method() )
Supported from v1.8.1
Returns a CxList which is a subset of this instance and its elements are the ones which their short name is the specified string, according to the specified comparison criteria.
Expand source
public CxList FindByShortName(string nodeName, bool CaseSensitive)
nodeName The short name of the objects to look for. Prefix and postfix wildcard * are supported.
caseSensitive Boolean which indicates to the search to be (or not) case sensitive.
A subset of this instance and its elements are the ones which their short name is the specified string, according to the specified comparison criteria.Where the caseSensitive value can be true for case sensitive and false for case insensitive.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrate the CxList.FindByShortName(string, bool) method.
The input source code is:
Expand source
MyClass a; int b; a.DataMember = 3; b = a.Method();
The source code that uses CxList.FindByShortName method:
Expand source
result = All.FindByShortName("method", true);
The result would consist of 0 items.
Another example of this method:
Expand source
result = All.FindByShortName("method", false);
The result would consist of 1 item:
Expand source
a.Method (in b = a.Method() )
Supported from v1.8.1
FindByShortNames
Returns a CxList which is a subset of this instance and its elements are the ones which their short name is the specified list of strings.
Expand source
public CxList FindByShortNames(List<string> nodeNames)
nodeNames The short names of the objects to look for. Prefix and postfix wildcard * are supported.
A subset of this instance and its elements are the ones which their name listed in specified list of strings.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0). Works efficient if wildcard not present.
This example demonstrate the CxList.FindByShortNames(List<string>) method.
The input source code is:
Expand source
MyClass a; int b; a.DataMember = 3; b = a.Method(); c = a.Method1();
The source code that uses CxList.FindByShortNames method:
Expand source
result = All.FindByShortNames(new List<string> {"Method",”Method1”});
The result would consist of 2 items.
Expand source
Method ( in b = a.Method() ) Method1(in c = a.Method1())
Supported from v7.1.8
Returns a CxList which is a subset of this instance and its elements are the ones which their short name is the specified array of strings.
Expand source
public CxList FindByShortNames(string[] nodeNames)
nodeNames The short names of the objects to look for. Prefix and postfix wildcard * are supported.
A subset of this instance and its elements are the ones which their name listed in specified array of strings.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0). Works efficient if wildcard not present.
This example demonstrate the CxList.FindByShortNames(string[]) method.
The input source code is:
Expand source
MyClass a; int b; a.DataMember = 3; b = a.Method(); c = a.Method1();
The source code that uses CxList.FindByShortNames method:
Expand source
result = All.FindByShortNames(new string[] {"Method",”Method1”});
The result would consist of 2 items.
Expand source
Method ( in b = a.Method() ) Method1(in c = a.Method1())
Supported from v7.1.8
Returns a CxList which is a subset of this instance and its elements are the ones which their short name is the specified string, according to the specified comparison criteria.
Expand source
public CxList FindByShortNames(List<string> nodeNames, bool caseSensitive)
nodeNames The short names of the objects. Prefix and postfix wildcard * are supported.
caseSensitive Boolean which indicates to the search to be (or not) case sensitive.
A subset of this instance and its elements are the ones which their short name is the specified string, according to the specified comparison criteria.Where the caseSensitive value can be true for case sensitive and false for case insensitive.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0). Works efficient if wildcard not present.
This example demonstrate the CxList.FindByShortNames(List<string>, bool) method.
The input source code is:
Expand source
MyClass a; int b; a.DataMember = 3; b = a.Method(); c = a.Method1();
The source code that uses CxList.FindByShortNames method:
Expand source
result = All.FindByShortNames(new List<string> {"method",”Method1”}, true);
The result would consist of 1 item:
Expand source
c = a.method1();
Another example of this method:
Expand source
result = All.FindByShortNames(new List<string> {"method",”Method1”}, false);
The result would consist of 2 items:
Expand source
a.Method (in b = a.Method() ) a.method1 (in c = a.method1() )
Supported from v7.1.8
Returns a CxList which is a subset of this instance and its elements are the ones which their short name is the specified string, according to the specified comparison criteria.
Expand source
public CxList FindByShortNames(string[] nodeNames, bool CaseSensitive)
nodeNames Contains the short name of the objects. Prefix and postfix wildcard * are supported.
caseSensitive Boolean which indicates to the search to be (or not) case sensitive.
A subset of this instance and its elements are the ones which their short name is the specified string, according to the specified comparison criteria.Where the caseSensitive value can be true for case sensitive and false for case insensitive.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0). Works efficient if wildcard not present.
This example demonstrate the CxList.FindByShortNames(string[], bool) method.
The input source code is:
Expand source
MyClass a; int b; a.DataMember = 3; b = a.Method(); c = a.Method1();
The source code that uses CxList.FindByShortNames method:
Expand source
result = All.FindByShortNames(new string[] {"method",”Method1”}, true);
The result would consist of 1 item:
Expand source
c = a.method1();
Another example of this method:
Expand source
result = All.FindByShortNames(new string[] {"method",”Method1”}, false);
The result would consist of 2 items:
Expand source
a.Method (in b = a.Method() ) a.method1 (in c = a.method1() )
Supported from v7.1.8
FindByType
Returns a CxList which is a subset of this instance and its elements are of the specified type of code element.
Expand source
public CxList FindByType(Type type)
type The type of the objects to be found.
A subset of this instance and its elements are of the specified type of code element.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrate the CxList.FindByType(Type) method.
The input source code is:
Expand source
MyClass a; int b; a.DataMember = 3; b = a.Method();
The source code that uses CxList.FindByType method:
Expand source
result = All.FindByType (typeof(MemberAccess));
The result would consist of 2 items:
Expand source
a.DataMember (in a.DataMember = 3) a.Method (in b = a.Method())
Supported from v1.8.1
Returns a CxList which is a subset of this instance and its elements are of the given type of code element.
Expand source
public CxList FindByType<T>()
T
A subset of this instance and its elements are of the given type of code element.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrate the CxList.FindByType<T>() method.
The input source code is:
Expand source
MyClass a; int b; a.DataMember = 3; b = a.Method();
The source code that uses CxList.FindByType method:
Expand source
result = All.FindByType<MemberAccess>();
The result would consist of 2 items:
Expand source
a.DataMember (in a.DataMember = 3) a.Method (in b = a.Method())
Supported from v9.4
Returns a CxList which is a subset of this instance and its elements are of the specified type.
Expand source
public CxList FindByType(string type)
type The type of the objects to be found.
A subset of this instance and its elements are of the specified type.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrate the CxList.FindByType(string) method.
The input source code is:
Expand source
MyClass a; int b; a.DataMember = 3; b = a.Method();
The source code that uses CxList.FindByType method:
Expand source
result = All.FindByType ("MyClass");
The result would consist of 3 items:
Expand source
a (in MyClass a) a (in a.DataMember = 3) a (in b = a.Method())
Supported from v1.8.1
Returns a CxList which is a subset of this instance and its elements are of the specified type.
Expand source
public CxList FindByType(string type, bool CaseSensitive)
type The type of the objects to be found.
caseSensitive Ignore case true/false.
A subset of this instance and its elements are of the specified type.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrate the CxList.FindByType(string, bool) method.
The input source code is:
Expand source
MyClass a; int b; a.DataMember = 3; b = a.Method();
The source code that uses CxList.FindByType method:
Expand source
result = All.FindByType ("MyClass", true);
The result would consist of 3 items:
Expand source
a (in MyClass a) a (in a.DataMember = 3) a (in b = a.Method())
Supported from v1.8.1
The method returns ICxListProvider of elements that has a type delivered in first parameter
types Type of parameters that method will look for
None
FindByTypeModifiers
Returns a CxList which is a subset of this instance and its elements are of the specified type modifiers of code element.
Expand source
public CxList FindByTypeModifiers(TypeSignednessModifiers typeSignedness, TypeSizeModifiers TypeSize)
typeSignedness The type of the objects to be found. It can receive the following alternative values: TypeSignednessModifiers.Unknown, TypeSignednessModifiers.Signed, TypeSignednessModifiers.Unsigned.
typeSize The type of the objects to be found. It can receive the following alternative values: TypeSizeModifiers.Default, TypeSizeModifiers.Short, TypeSizeModifiers.Long, TypeSizeModifiers.LongLong.
A subset of this instance which elements type contains both modifiers provided.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrate the CxList.FindByTypeModifiers(TypeSignednessModifiers, TypeSizeModifiers) method.
The input source code is:
Expand source
unsigned long int a; int b; b = a++;
The source code that uses CxList.FindByTypeModifiers method:
Expand source
result = All.FindByTypeModifiers (TypeSignednessModifiers.Unsigned, TypeSizeModifiers.Long);
The result would consist of 3 items:
Expand source
int (in unsigned long int a) a(in unsigned long int a) a(in b = a++)
Supported from v8.8.0
Returns a CxList which is a subset of this instance and its elements are of the specified type modifiers of code element.
Expand source
public CxList FindByTypeModifiers(TypeSignednessModifiers typeSignedness)
typeSignedness The type of the objects to be found. It can receive the following alternative values: TypeSignednessModifiers.Unknown, TypeSignednessModifiers.Signed, TypeSignednessModifiers.Unsigned.
A subset of this instance which elements type contains the modifier provided.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrate the CxList.FindByTypeModifiers(TypeSignednessModifiers) method.
The input source code is:
Expand source
unsigned long int a; int b; b = a++;
The source code that uses CxList.FindByTypeModifiers method:
Expand source
result = All.FindByTypeModifiers (TypeSignednessModifiers.Unsigned);
The result would consist of 3 items:
Expand source
int (in unsigned long int a) a(in unsigned long int a) a(in b = a++)
Supported from v8.8.0
Returns a CxList which is a subset of this instance and its elements are of the specified type modifiers of code element.
Expand source
public CxList FindByTypeModifiers(TypeSizeModifiers typeSize)
typeSize The type of the objects to be found. It can receive the following alternative values: TypeSizeModifiers.Default, TypeSizeModifiers.Short, TypeSizeModifiers.Long, TypeSizeModifiers.LongLong.
A subset of this instance which elements type contains the modifier provided.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrate the CxList.FindByTypeModifiers(TypeSizeModifiers) method.
The input source code is:
Expand source
unsigned long int a; int b; b = a++;
The source code that uses CxList.FindByTypeModifiers method:
Expand source
result = All.FindByTypeModifiers (TypeSizeModifiers.Long);
The result would consist of 3 items:
Expand source
int (in unsigned long int a) a(in unsigned long int a) a(in b = a++)
Supported from v8.8.0
FindByTypeSignednessAndSizeModifiers
Returns a CxList which is a subset of "this" instance and its elements are the ones which their type modifier signedness and size is the specified parameters.
typeSignedness Attribute of the type signedness to be found (this parameter can be null).
typeSize Attribute of the type size to be found (this parameter can be null).
A subset of this instance and its elements are the ones which their type modifier signedness and size is the specified in the parameters, according to the specified comparison criteria.
FindByTypes
Returns a CxList which is a subset of this instance and its elements are of the specified list of types.
Expand source
public CxList FindByTypes(params Type[])
types The list of types of the objects to be found.
A subset of this instance and its elements are of the specified list of types.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrate the CxList.FindByTypes(Type[]) method.
The input source code is:
Expand source
MyClass a; a.DataMember = 3;
The source code that uses CxList.FindByTypes method:
Expand source
result = All.FindByTypes(typeof(MemberAccess), typeof(AssignExpr));
The result would consist of 2 items:
Expand source
a.DataMember (in a.DataMember = 3) // MemberAccess = (in a.DataMember = 3) // AssignExpr
Supported from v1.8.1
Returns a CxList which is a subset of this instance and its elements are of the specified types.
Expand source
public CxList FindByTypes(params string[] types)
types The types of the objects to be found.
A subset of this instance and its elements are of the specified types.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrate the CxList.FindByTypes(string[]) method.
The input source code is:
Expand source
MyClass a; int b; a.DataMember = 3; b = a.Method();
The source code that uses CxList.FindByTypes method:
Expand source
String[] arr = new String[]{"MyClass","int"}; result = All.FindByTypes(arr);
The result would consist of 6 items:
Expand source
a (in MyClass a) a (in a.DataMember = 3) a (in b = a.Method()) b (in int b) b (in b = a.Method()) MyClass (in MyClass a)
Supported from v1.8.1
Returns a CxList which is a subset of this instance and its elements are of the specified types.
Expand source
public CxList FindByTypes(params string[] types, bool CaseSensitive)
types The types of the objects to be found.
caseSensitive Ignore case true/false.
A subset of this instance and its elements are of the specified types.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrate the CxList.FindByTypes(string[], bool) method.
The input source code is:
Expand source
MyClass a; int b; a.DataMember = 3; b = a.Method();
The source code that uses CxList.FindByTypes method:
Expand source
String[] arr = new String[]{"MyClass","int"}; result = All.FindByTypes(arr, false);
The result would consist of 6 items:
Expand source
a (in MyClass a) a (in a.DataMember = 3) a (in b = a.Method()) b (in int b) b (in b = a.Method()) MyClass (in MyClass a)
Supported from v7.1.8
FindChildPropertiesByJsonPath
Returns a CxList with CxJsonProperty elements following the values defined by the parameters.
Expand source
public CxList FindChildPropertiesByJsonPath(string filesFilter, int language, string path)
filesFilter File extension pattern to be used as filter.
language Id of the language.
path The path in the Json file based on the JsonPath expression.
Returns a CxList with CxJsonProperty elements.
None
Expand source
{ "proxies": { "rootspath": { "httpProxy": "http://10.0.50.1:3128" }, "mypath": "http://10.0.50.1:8080" } }
The source code that uses FindChildPropertiesByJsonPath method:
Expand source
CxList res = cxJson.FindChildPropertiesByJsonPath ("*.json", 2, "$.proxies"); // Result now holds the rootpath and the mypath value (http://10.0.50.1:8080)
Supported from 9.2.0
FindCountByRegex
Not used
expression None
None
FindCustomAttributeParameterByKey
Returns a ICxListProvider that contains the parameter of the custom attribute and specified key.
name The attribute name.
keyName the parameter key
A ICxListProvider that contains the parameter of the custom attribute and corresponding parameter key of the specified name.
FindCustomAttributeParameters
Returns a ICxListProvider that contains the parameters of the custom attribute.
name The attribute name.
A ICxListProvider that contains the parameters of the custom attribute of the specified name.
FindDefinition
Returns a CxList which is a subset of "this" instance, with elements that are the definition locations of the first element in the given CxList.
Expand source
public CxList FindDefinition(CxList Ids)
ids Items whose definition to be found.
A subset of "this" instance, with elements that are the definition locations of the first element in the specified CxList.
ArgumentNullException : parameter is a null reference
The return value may be empty (Count = 0).
This example demonstrates the CxList.FindDefinition() method.
The input source code is:
Expand source
int b, a = 5; if (a > 3) b = a;
The source code that uses CxList.FindDefinition method:
Expand source
result = All.FindDefinition(All.FindByName("*b*"));
The result would consist of 1 item:
Expand source
b (in int b, a = 5)
Supported from v1.8.1
FindDefinitionTypeRef
Find the TypeRef for any reference received Can be used for TypeRef or PointerTypeRef
T Type of members to find definition type
graphValue The reference value to be searched the definition type
The Typeref object which is set at the definition of the given graph, or null if none is available
FindDefinition_old
Returns a ICxListProvider which is a subset of this instance, with elements that are the definition locations of the first element in the specified ICxListProvider.
ids Items whose definition to be found.
A subset of this instance, with elements that are the definition locations of the first element in the specified ICxListProvider.
FindDescendantsOfType
Returns a CxList which contains the direct descendants of a given instance members, filter by type.
Expand source
public CxList FindDescendantsOfType<T>(CxList ancs)
T the type to cast the DOM object to (must inherit from CSharpGraph)
ancs The Ancestors whose descendants are to be returned.
Return a CxList with the direct descendants of a given instance members, filter by type.
ArgumentNullException : parameter is a null reference
The return value may be empty (Count = 0).
This example demonstrates the CxList.InheritsFrom() method.
The input source code is:
Expand source
class BClass { Int field1; Float field2; }
The source code that uses CxList.FindDescendantsOfType method:
Expand source
classList = Find_ClassDecl(); result = All.FindDescendants<FieldDecl>(classList);
The result would consist of 2 items:
Expand source
field1, field2
Supported from v9.4
Returns a CxList which contains the direct descendants of a given instance members, filter by type.
Expand source
public CxList FindDescendantsOfType<T>(CxList ancs, Type type)
ancs The Ancestors whose descendants are to be returned.
type the type of the DOM object
Return a CxList with the direct descendants of a given instance members, filter by type.
ArgumentNullException : parameter is a null reference
The return value may be empty (Count = 0).
This example demonstrates the CxList.InheritsFrom() method.
The input source code is:
Expand source
class BClass { Int field1; Float field2; }
The source code that uses CxList.FindDescendantsOfType method:
Expand source
classList = Find_ClassDecl(); result = All.FindDescendants(classList, typeof(FieldDecl));
The result would consist of 2 items:
Expand source
field1, field2
Supported from v9.4
FindInScope
Returns a CxList which is a subset of “this” instance with the elements inside the scopes defined by the start nodes CxList and the end nodes CxList.
Expand source
public CxList FindInScope(CxList StartNodes, CxList EndNodes)
startNodes The nodes that define the start of the scope.
endNodes The nodes that define the start of the scope.
All nodes found inside the given scope.
This example demonstrates the CxList.FindInScope(CxList StartNodes, CxList EndNodes) method.
The input source code is:
Expand source
@{ Html.BeginForm(); } //Defines the start of the scope @Html.AntiForgeryToken(); @{ Html.EndForm(); } //Defines the end of the scope
The source code that uses CxList.FindInScope method:
Expand source
CxList BeginFormMethods = All.FindByShortName("BeginForm"); CxList EndFormMethods = All.FindByShortName("EndForm"); result = All.FindInScope(BeginFormMethods, EndFormMethods);
The result would consist of 1 item:
Expand source
AntiForgeryToken(@Html.AntiForgeryToken();)
The purpose of the query is to find anything that is contained inside a specific scope.
Supported from 8.5.0
FindInitialization
Returns a CxList which is a subset of “this” instance and the elements are the initialization values of the elements from the given CxList.
Expand source
public CxList FindInitialization(CxList declarators)
declaratorList A CxList of declarators.
A subset of “this” instance whose elements are the initialization values of the given CxList elements.
ArgumentNullException : parameter is a null reference
The return value may be empty (Count = 0).
This example demonstrates the CxList.FindInitialization() method.
The input source code is:
Expand source
int b = 5;
Expand source
CxList declarators = All.FindByType(typeof(Declarator)); result = All.FindInitialization(declarators);
The result would consist of 1 item:
Expand source
5
Supported from v1.8.1
FindJsonPropertyByName
Returns a CxList with CxJsonProperty elements following the values defined by the parameters.
Expand source
public CxList FindJsonPropertyByName (string filesFilter, int language, string name, bool usesRegex = false, bool ignoreCase = false)
filesFilter File extension pattern to be used as filter.
language Id of the language.
name The name of the property.
usesRegex Specifies whether the search should use regex. This field is not required and its default value is false
ignoreCase Specifies whether the search should be case sensitive. This field is not required and its default value is false.
Returns a CxList with CxJsonProperty elements
None
Expand source
{ "proxies": { "default": { "httpProxy": "http://10.0.50.1:3128", "httpsProxy": "http://10.0.50.1:3128" } } }
The source code that uses FindJsonPropertyByName method:
Expand source
result=cxJson.FindJsonPropertyByName("config.json", 8, "proxy$", true, true); // Result now holds two CxJsonProperty.
Supported from 9.2.0
FindJsonPropertyByNameAndValue
Returns a CxList with CxJsonProperty elements following the values defined by the parameters.
Expand source
public CxList FindJsonPropertyByNameAndValue (string filesFilter, int language, string name, string value, bool usesRegex = false, bool ignoreCase = false)
filesFilter File extension pattern to be used as filter.
language Id of the language.
name The name of the property.
value The value of the property.
usesRegex Specifies whether the search should use regex. This field is not required and its default value is false.
ignoreCase Specifies whether the search should be case sensitive. This field is not required and its default value is false.
Returns a CxList with CxJsonProperty elements
None
Expand source
{ "proxies": { "default": { "httpProxy": "http://10.0.50.1:3128", "httpsProxy": "http://10.0.50.1:3128" } } }
The source code that uses FindJsonPropertyByNameAndValue method:
Expand source
result=cxJson.FindJsonPropertyByNameAndValue("*.json", 8, "^https", “^http:”, true, false); // Result now holds one CxJsonProperty
Supported from 9.2.0
FindJsonPropertyByValue
Returns a CxList with CxJsonProperty elements following the values defined by the parameters.
Expand source
public CxList FindJsonPropertyByValue (string filesFilter, int language, string value, bool usesRegex = false, bool ignoreCase = false)
filesFilter File extension pattern to be used as filter.
language Id of the language.
value The value of the property.
usesRegex Specifies whether the search should use regex. This field is not required and its default value is false.
ignoreCase Specifies whether the search should be case sensitive. This field is not required and its default value is false.
Returns a CxList with CxJsonProperty elements
None
Expand source
{ "proxies": { "default": { "httpProxy": "http://10.0.50.1:3128", "httpsProxy": "http://10.0.50.1:3128" } } }
The source code that uses FindJsonPropertyByValue method:
Expand source
result=cxJson.FindJsonPropertyByValue("*.json", 8, "^http", true, false); // Result now holds two CxJsonProperty
Supported from 9.2.0
FindParameterByName
Returns a CxList which is a subset of “this” instance and its elements are parameters of the given CxList with the specified parameters.
Expand source
public CxList FindParameterByName (string paramName)
paramName String containing the name of the parameter.
caseSensitive Bool with case Sensitive option
A subset of "this" instance where its elements are Params and these contains arguments labelled according to 'paramName'.
ArgumentNullException : parameter is a null reference
The return may be empty (Count = 0)
This example demonstrates the CxList.FindParameterByName() method.
The input source code is:
Expand source
foo(param1:"myVar");
The source code that uses CxList.FindParameterByName method:
Expand source
result = All. FindParameterByName(“param1”);
The result would consist of 1 item.
Expand source
param1
Supported from v9.2.0
FindParsingIssues
Returns parsing errors
None
FindRegexMatches
Return a subset of 'this' instance which objects are of type Comment, and are equivalent to objects of type Comment in the comments CxList.
Expand source
public CxList FindRegexMatches(CxList comments)
comments A CxList of Comment type objects to find matches against the Comment objects in 'this'
Return a subset of 'this' instance which objects are of type Comment, and are equivalent to objects of type Comment in the comments CxList.
ArgumentNullException : Thrown when parameter is a null reference.
This example demonstrate the CxList.FindRegexMatches(CxList) method.
Expand source
// Each time a FindByRegexExt or FindByRegex generate Comment type objects, they are get a different NodeId, even if the represent the same string in the project code. CxList a = All.FindByRegexExt("http://"); a.Add(All.FindByRegexExt("https://")); CxList b = All.FindByRegexExt("http://"); // The strings that starts with http:// in 'a', now exist in 'b' but with a different NodeId number. Result = a.FindRegexMatches(b); // Return a subset of 'b' where the objects returned are equivalent to other objects in 'a'.
Supported from version 8.4.0
FindSQLInjections
Returns flow for SQL Injection from input to db that is not sanitized
Expand source
public CxList FindSQLInjections (CxList inputs, CxList db, CxList sanitize)
inputs CxList containing input elements
db CxList containing output elements (eg. database)
sanitize CxList containing sanitizing elements (cast to integer etc)
CxList containing flow of SQL injection from input to output which is not flowing through a sanitizer
Actually uses inputs.InfluencingOnAndNotSanitized(db, sanitize)
The source code that uses CxList.FindSQLInjections method:
Expand source
// Find the inputs for the SQL injection CxList inputs = All.FindByShortName("ReadLine"); //Find the entrance to the Database command CxList dbIn = All.GetParameters(All.FindByShortName("SqlCommand")); //Find a potential sanitizer CxList integerSanitizers = Find_Integers(); //Find sql injections using previous results result = All.FindSQLInjections(inputs, dbIn, integerSanitizers);
FindSubList
Returns a CxList which is a subset of “this”. The following interface provides the ability to extract N elements from the CxList. The main purpose of this interface is NOT use with member “data” of CxList.
Expand source
public CxList FindSubList(int count, bool fromStart)
count Number of nodes to extract.
fromStart If the parameter value is true it means get elements from start of the list If the parameter value is false it means get elements from end of the list
Requested count elements.
Example 1: Get last element and add it to result
Expand source
// Current Implementation CSharpGraph secondParam = secondParameterOfSetHeader.data.GetByIndex(secondParameterOfSetHeader.Count-1) as CSharpGraph; result.Add(secondParam.NodeId, secondParam); // New Implementation result.Add(secondParameterOfSetHeader.FindSubList (1,false));
Example 2: Get first and second element. Assume that all elements are BinaryExpr.
Expand source
// Current Implementation // currently not supporting logical conditions with more than two sons if (curSons.Count >= 2) { continue; } BinaryExpr firstOp = curSons.data.GetByIndex(0) as BinaryExpr; BinaryExpr secondOp = curSons.data.GetByIndex(1) as BinaryExpr; // New Implementation // currently not supporting logical conditions with more than two sons if (curSons.Count >= 2) { continue; } // get first 2 elements of the list CxList secondOpTemp = curSons.FindSubList(2, true)); // get first one BinaryExpr firstOp = secondOpTemp.FindSubList(1, true)).TryGetCSharpGraph<BinaryExpr>(); // get last one (second of original list) BinaryExpr secondOp = secondOpTemp.FindSubList(1, false)).TryGetCSharpGraph<BinaryExpr>();
Supported from 9.2.0
FindXSS
Returns flow for XSS from input to output that is not sanitized
Expand source
public abstract CxList FindXSS(CxList inputs, CxList outputs, CxList sanitize)
inputs CxList containing input elements
outputs CxList containing output elements for xss.
sanitize CxList containing sanitizing elements (cast to integer etc)
CxList containing flow of XSS from input to output which is not flowing through a sanitizer
Actually uses inputs.InfluencingOnAndNotSanitized(db, sanitize) The source code that uses CxList.FindXSS method:
CxList inputs = All.FindByShortName("request");
CxList outputs = All.FindByShortName("output");
CxList sanitize = All.FindByShortName("escape");
result = All.FindXSS(inputs, outputs, sanitize);
FindXmlAttributesByName
Returns a CxList with CxXmlNode elements that contain attributes with the same name defined in the parameters
Expand source
public CxList FindXmlAttributesByName(string xmlFilterFiles, int language, string attributeName, bool ignoreCase = false )
xmlFilterFiles File extension pattern to be used as filter.
language Id of the language.
attributeName The name of the attribute.
ignoreCase Specifies if the search should be case sensitive. This field is not required and its default value is false.
Return a CxList with CxXmlNode elements.
The input source code is:
Expand source
<div id="error-section"></div>
Expand source
The source code that uses FindXmlAttributesByName method: result = cxXPath. FindXmlAttributesByName("*.app", 8, "id", true);
Supported from 8.6.0
FindXmlAttributesByNameAndValue
Returns a CxList with CxXmlNode elements that contain attributes with the same name and value defined in the parameters.
Expand source
public CxList FindXmlAttributesByNameAndValue(string xmlFilterFiles, int language, string attributeName, string attributeValue, bool usesRegex = false, bool ignoreCase = false)
xmlFilterFiles File extension pattern to be used as filter.
language Id of the language.
attributeName The name of the attribute.
attributeValue The value of the attribute.
usesRegex Specifies if the search should use regex.
ignoreCase Specifies if the search should be case sensitive. This field is not required and its default value is false.
Return a CxList with CxXmlNode elements.
The input source code is:
Expand source
<div id="error-section"></div>
The source code that uses FindXmlAttributesByNameAndValue method:
Expand source
result = cxXPath.FindXmlAttributesByNameAndValue("*.app", 8, "id", "error-section", false, true); // Result now holds the entire tag block
Supported from 8.6.0
FindXmlAttributesByValue
Returns a CxList with CxXmlNode elements that contain attributes with the same value defined in the parameters.
Expand source
public CxList FindXmlAttributesByValue(string xmlFilterFiles, int language, string attributeValue, bool usesRegex = false)
xmlFilterFiles File extension pattern to be used as filter.
language Id of the language.
attributeValue The value of the attribute.
usesRegex Specifies if the search should use regex.
Return a CxList with CxXmlNode elements.
The input source code is:
Expand source
<div id="error-section"></div>
The source code that uses FindXmlAttributesByValue method:
Expand source
result = cxXPath.FindXmlAttributesByValue("*.app",8,"error-section", false); // Result now holds the entire tag block
FindXmlNodesByLocalName
Returns a CxList with CxXmlNode elements that contain the same local name defined in the parameters.
Expand source
public CxList FindXmlNodesByLocalName(string xmlFilterFiles, int language, string nodeName, bool includeAttributes = false, string attributeName = ””, string attributeValue = ””, bool usesRegex = false, bool ignoreCase = false)
xmlFilterFiles File extension pattern to be used as filter.
language Id of the language.
nodeName The name of the node.
includeAttributes Specifies whether the search includes the attributes. This field is not required and its default value is false.
attributeName The name of the attribute. This field is not required.
attributeValue The value of the attribute. This field is not required.
usesRegex Specifies if the search should use regex. This field is not required and its default value is false.
ignoreCase Specifies if the search should be case sensitive. This field is not required and its default value is false.
Return a CxList with CxXmlNode elements.
The input source code is:
Expand source
<a href="{!obj.href}">click me</a>
The source code that uses FindXmlNodesByLocalName method:
Expand source
result = cxXPath. FindXmlNodesByLocalName("*.cmp", 8, "a", true, "href", "[{][!][^}]+[}]", true, true); // Result now holds the entire tag block
Supported from 8.6.0
FindXmlNodesByLocalNameAndValue
Returns a CxList with CxXmlNode elements that contain the same local name and value defined in the parameters.
Expand source
public CxList FindXmlNodesByLocalNameAndValue(string xmlFilterFiles, int language, string nodeName, string nodeValue, bool usesRegexForNodeValue = false, bool ignoreCase = false)
xmlFilterFiles File extension pattern to be used as filter.
language Id of the language.
nodeName The name of the node.
nodeValue The value of the node.
usesRegexForNodeValue Specifies if the search should use regex for the node value. This field is not required and its default value is false.
ignoreCase Specifies if the search should be case sensitive. This field is not required and its default value is false
Returns a CxList with CxXmlNode elements.
The input source code is:
Expand source
<a href="{!obj.href}">click me</a>
The source code that uses FindXmlNodesByLocalNameAndValue method:
Expand source
result = cxXPath. FindXmlNodesByLocalNameAndValue("*.cmp", 8, "a", “click me”, true, "href", "[{][!][^}]+[}]", true, true);
Supported from 8.6.0
FindXmlNodesByQualifiedName
Return a CxList with CxXmlNode elements following the values defined by the parameters.
Expand source
public CxList FindXmlNodesByQualifiedName(string xmlFilterFiles, int language, string prefix, string nodeName, bool includeAttributes, string attributeName “”, string attributeValue = “”, bool usesRegex = false, bool ignoreCase = false)
xmlFilterFiles File extension pattern to be used as filter.
language Id of the language.
prefix The name of the prefix.
nodeName The name of the node.
includeAttributes Specifies if the search should include attributes. This field is not required and its default value is false.
attributeName The name of the attribute. This field is not required.
attributeValue The value of the attribute. This field is not required.
usesRegex Specifies if the search should use regex. This field is not required and its default value is false.
ignoreCase Specifies if the search should be case sensitive. This field is not required and its default value is false.
Return a CxList with CxXmlNode elements.
The input source code is:
Expand source
<aura:attribute name="href" type="String" default="null"/>
The source code that uses FindXmlNodesByQualifiedName method:
Expand source
result = cxXPath.FindXmlNodesByQualifiedName("*Test.app",8, "aura", "type"); // Result now holds the entire tag block
Supported from 8.6.0
FindXmlNodesByQualifiedNameAndValue
Returns a CxList with CxXmlNode elements that contain the same name and value defined in the parameters.
xmlFilterFiles File extension pattern to be used as filter.
language Id of the language.
prefix The name of the prefix
nodeName The name of the node.
nodeValue The value of the node.
usesRegexForNodeValue Specifies if the search should use regex for the node value. This field is not required and its default value is false.
ignoreCase Specifies if the search should be case sensitive. This field is not required and its default value is false.
Return a CxList with CxXmlNode elements.
The input source code is:
Expand source
<aura:attribute name="href" type="String" default="null"> {!userManager.isAuthorized} <aura:attribute/>
The source code that uses FindXmlNodesByQualifiedNameAndValue method:
Expand source
result = cxXPath. FindXmlNodesByQualifiedNameAndValue("*Test.app", 8, "aura", "attribute", “userManager.isAuthorized”); // Result now holds the entire tag block
FindYamlNodesByKey
Returns a CxList with CxYamlNode elements following the key defined in the parameters.
Expand source
public CxList FindYamlNodesByKey(string filesFilter, int language, string key, bool usesRegex = false, bool ignoreCase = false)
filesFilter File extension pattern to be used as filter.
language Id of the language.
key The key of the property.
usesRegex Specifies whether the search should use regex. This field is not required and its default value is false
ignoreCase Specifies whether the search should be case sensitive. This field is not required and its default value is false.
Returns a CxList with CxYamlNode elements
None
Expand source
spring: application: name: "myapp" config: import: "optional:file:./dev.properties" additionalProperty1: "smtp.host: smtp.example.com" additionalProperty2: "api.key: your-api-key-here"
The source code that uses FindYamlNodesByKey method:
Expand source
result=cxYaml.FindYamlNodesByKey("config.yaml", 2, "^additional", true, true); // Result now holds two CxYamlNode.
Supported from 9.6.2
FindYamlNodesByKeyAndValue
Returns a CxList with CxYamlNode elements following the key adn value defined in the parameters.
Expand source
public CxList FindYamlNodesByKeyAndValue(string filesFilter, int language, string key, string value, bool usesRegex = false, bool ignoreCase = false)
filesFilter File extension pattern to be used as filter.
language Id of the language.
key The key of the property.
value The value of the property.
usesRegex Specifies whether the search should use regex. This field is not required and its default value is false
ignoreCase Specifies whether the search should be case sensitive. This field is not required and its default value is false.
Returns a CxList with CxYamlNode elements
None
Expand source
spring: application: name: "myapp" config: import: "optional:file:./dev.properties" additionalProperty1: "smtp.host: smtp.example.com" additionalProperty2: "api.key: your-api-key-here"
The source code that uses FindYamlNodesByKeyAndValue method:
Expand source
result=cxYaml.FindYamlNodesByKeyAndValue("config.yaml", 2, "name", "myapp"); // Result now holds one CxYamlNode.
Supported from 9.6.2
FindYamlNodesByValue
Returns a CxList with CxYamlNode elements following the value defined in the parameters.
Expand source
public CxList FindYamlNodesByValue(string filesFilter, int language, string value, bool usesRegex = false, bool ignoreCase = false)
filesFilter File extension pattern to be used as filter.
language Id of the language.
value The value of the property.
usesRegex Specifies whether the search should use regex. This field is not required and its default value is false
ignoreCase Specifies whether the search should be case sensitive. This field is not required and its default value is false.
Returns a CxList with CxYamlNode elements
None
Expand source
spring: application: name: "myapp" config: import: "optional:file:./dev.properties" additionalProperty1: "smtp.host: smtp.example.com" additionalProperty2: "api.key: your-api-key-here"
The source code that uses FindYamlNodesByValue method:
Expand source
result=cxYaml.FindYamlNodesByValue("config.yaml", 2, "myapp"); // Result now holds one CxYamlNode.
Supported from 9.6.2
GetAllExpressionDescendents
Returns a CxList which is all descendants of the descendentExpressionGroup.
Expand source
public CxList GetAllExpressionDescendents(CxList descendentExpressionGroup, int language)
descendentExpressionGroup The Ancestors whose descendants are to be returned
language Id of the language.
Returns all elements that descends any of the elements in the descendentExpressionGroup parameter
The input source code is:
Expand source
<iframe src="{!'https:' + v.frameSrc}">iframe</iframe>
Expand source
CxList attr=cxXPath.FindAllAttributesThatHoldExpressions("*.cmp", 8, "Lightning"); CxList expr = cxXPath.GetExpressionsByAttributes(attr); result=cxXPath.GetAllExpressionDescendents(expr,8); // Will return 4 results (binaryExpr, StringLiteral, MemberAccess, UnknownReference).
Supported from 8.9.0
GetAllFlow
get all paths of current node
None
GetAllInternalPath
get all paths in internal representation. each path can appears only once. key of those paths shouldn't be an object with an empty name (like =)
pathData The path data.
None
GetAllVertexes
Get list of all vertexes in DOM units according input parameters. last parameter use to convert from DFG to DOM coordinate.
None
GetAncOfType
Returns a CxList with all the elements that are CxDOM first ancestor of the calling CxList and which are of type t.First ancestor means that it searches upward in the CxDOM graph until the first ancestor matching the condition (type t), and NOT that it searches only for fathers.
Expand source
public CxList GetAncOfType(Type t)
type The type of DOM object the methods looks for
Returns a CxList with all the CxDOM elements of type t, which are first ancestor, of some element in the calling CxList.
ArgumentNullException : parameter is a null reference
The return value may be empty (Count = 0). This command does not return a subset of the CxList, but a subset of All.
The following code example shows how you can use the GetAncOfType method.
This example demonstrates the CxList.GetAncOfType() method.
The input source code is:
Expand source
if (a>b) { c = 100; } else { if (a<100) { d = 200; } }
The source code that uses CxList.GetAncOfType method:
Expand source
result = All.FindByName("d"). GetAncOfType(typeof(IfStmt));
The result would be -
Expand source
1 item found if (in if(a<100))
Supported from v2.0.5
Returns a CxList with all the elements that are CxDOM first ancestor of the calling CxList and which are of type "T". First ancestor means that it searches upward in the CxDOM graph until the first ancestor matching the generic type "T", and NOT that it searches only for fathers. Gets the first ancestors found of Type1 or Type2 or ... (etc) of the giving generic types
types The giving CxList generic types to search.
Returns the first ancestors of the giving CxList generic types.
Get the ancestors of the giving generic type.
Expand source
public CxList GetAncOfType<T>()
T The type to cast the DOM object to (must inherit from CSharpGraph)
Returns a CxList with all the CxDOM elements of type “T”, which are first ancestor, of some element in the calling CxList.
The return value may be empty (Count = 0). This command does not return a subset of the CxList, but a subset of All.
This example demonstrates the CxList.GetAncOfType<CSharpGraph>() method.
The input source code is:
Expand source
if (a>b) { c = 100; } else { if(a<100) { d = 200; } }
The source code that uses CxList.GetAncOfType method:
Expand source
result = All.FindByName("d").GetAncOfType<IfStmt>();
The result would be -
Expand source
1 item found: if (in if(a<100))
Supported from v9.4.0
GetArrayOfNodeIds
Returns an ArrayList which is a set of all elements IDs All this CxList.
Expand source
public ArrayList GetArrayOfNodeIds()
ArrayList which is a set of all elements IDs All this CxList.
ArgumentNullException : parameter is a null reference
The following code example shows how you can use the FindByReturnType method.
This example demonstrates the CxList.GetArrayOfNodeIds() method.
The input source code is:
Expand source
public class a { void foo() { MyClass a; int b; a.DataMember = 3; b = a.Method(); } }
The source code that uses CxList.GetArrayOfNodeIds method:
Expand source
CxList ls = All; foreach(int NodeId in ls.GetArrayOfNodeIds()) { if(NodeId !=1) { result = All.FindById(NodeId); } }
Supported from : v1.8.1
GetAssignee
For each 'a=b' statement, for every 'b' in 'this', find it's 'a'
None
For each DOM object in 'this' which is on the right side of an assignment, return the left side of the assignment, which are in the others CxList.
Expand source
public CxList GetAssignee(CxList others = null)
others CxList containing the left side of the assignment. If null – treat it as if it was All.
For each DOM object in 'this' which is on the right side of an assignment, return the left side of the assignment, which are in the others CxList.
This example demonstrates the CxList.GetAssignee(CxList) method.
The input source code is:
Expand source
int a = 0; int b = a; b = 2; int c = b > 1 ? 3 : a;
The source code that uses CxList.GetAssignee method:
Expand source
CxList a = All.FindByShortName("a"); CxList b = All.FindByShortName("b"); CxList c = All.FindByShortName("c"); result = a.GetAssignee(); // result now holds 'b' in int b = a; and c in int c = b > 1 ? 3 : a; result = a.GetAssignee(b); // result now holds 'b' in int b = a;
Supported from version 8.4.0
For each DOM object in 'this' which is on the right side of an assignment, return the left side of the assignment, which are in the others CxList, or the corresponding node that is the assigneeNo given on the left side of the assignment.
Expand source
public CxList GetAssignee(CxList others = null, int assigneeNo = -1)
others CxList containing the left side of the assignment. If null – treat it as if it was All.
assigneeNo int corresponding to the index of the assignees on the left – if -1 treat as All.
For each DOM object in 'this' which is on the right side of an assignment, return the left side of the assignment, which are in the others CxList, or the corresponding node that is the assigneeNo given on the left side of the assignment.
This examples demonstrate the CxList.GetAssignee(CxList, int) method.
The input source code is:
Expand source
int a = 0; int b = a; b = 2; int c = b > 1 ? 3 : a; d,e := something();
The source code that uses CxList.GetAssignee method:
Expand source
CxList a = All.FindByShortName("a"); CxList b = All.FindByShortName("b"); CxList c = All.FindByShortName("c"); result = a.GetAssignee(); // result now holds 'b' in int b = a; and c in int c = b > 1 ? 3 : a; result = a.GetAssignee(b); // result now holds 'b' in int b = a; CxList d = All.FindByShortName("something"); result = d.GetAssignee(0); // result now holds 'd' in d,e := something(); result = d.GetAssignee(1); // result now holds 'b' in d,e := something(); result = d.GetAssignee(-1); // result now holds ‘d’ and 'e' in d,e := something(); result = d.GetAssignee(2); // result now is empty in d,e := something();
Supported from version 9.4.0
For each DOM object in 'this' which is on the right side of an assignment, return the corresponding node that is the assigneeNo given on the left side of the assignment.
Expand source
public CxList GetAssignee(int assigneeNo)
assigneeNo int corresponding to the index of the assignees on the left – if -1 treat as All.
For each DOM object in 'this' which is on the right side of an assignment, return the corresponding node that is the assigneeNo given on the left side of the assignment.
This example demonstrate the CxList.GetAssignee(int) method.
The input source code is:
Expand source
a,b := something();
The source code that uses CxList.GetAssignee method:
Expand source
CxList a = All.FindByShortName("something"); result = a.GetAssignee(0); // result now holds 'a' in a,b := something(); result = a.GetAssignee(1); // result now holds 'b' in a,b := something(); result = a.GetAssignee(-1); // result now holds ‘a’ and 'b' in a,b := something(); result = a.GetAssignee(2); // result now is empty in a,b := something();
Supported from version 9.4.0
GetAssigner
For each 'a=b' statement, for every 'a' in 'this', find it's 'b'
None
For each DOM object in 'this' which is on the left side of an assignment, return the right side of the assignment, which are in the others CxList.
Expand source
public CxList GetAssigner(CxList others = null)
others CxList containing the right side of the assignment. If null – treat it as if it was All.
For each DOM object in 'this' which is on the left side of an assignment, return the right side of the assignment, which are in the others CxList.
This example demonstrates the CxList.GetAssigner(CxList) method.
The input source code is:
Expand source
int a = 0; int b = a; b = 2; int c = b > 1 ? 3 : a;
The source code that uses CxList.GetAssigner method:
Expand source
CxList a = All.FindByShortName("a"); CxList b = All.FindByShortName("b"); CxList c = All.FindByShortName("c"); result = b.GetAssigner(); // result now holds 'a' in int b = a; and 2 in b = 2; result = c.GetAssigner(); // result now holds 3 and 'a' in int c = b > 1 ? 3 : a; result = c.GetAssigner(a); // result now holds 'a' in int c = b > 1 ? 3 : a;
Supported from version 8.4.0
GetAttributeByExpression
returns a set of attribute keys for the expressions that set as their values
expressions the expressions to link from
a CxList that contains all CxXMLNodes that represent the attribute keys that are linked to the expressions as value
GetBackwardDepthCountFlowEdge
This query helps investigate results that are missing due to the flow extending beyond the edge of our 'Depth Count'. The query returns the flow to nodes where future flow is cut off due to depth count (that is, the last reachable node). Hopefully, this will clearly show the 'edge' of the depth count.
GetBlocksOfIfStatements
Returns a CxList with all the true/false blocks of the if statements inside the calling CxList according the provided boolean parameter.The true block of an if statement is the block which is ran if the condition is verified, whereas the false block is the else block(if it exists).
Expand source
public CxList GetBlocksOfIfStatements(Boolean block)
block A Boolean describing weather you want to retrieve the true or false blocks of the if statements inside the calling CxList.
Returns a CxList containing all the true/false blocks of the if statements contained in the calling CxList.
ArgumentNullException : parameter is a null reference
The return value may be empty (Count = 0). This command does not return a subset of the CxList, but a subset of All.
This example demonstrates the CxList.GetBlocksOfIfStatements(block) method.
The input source code is:
Expand source
int a = 5; if ( a > 3) { a = 4; } else if (a > 2) { a = 2; } else { a = 8; }
The source code that uses CxList.GetBlocksOfIfStatements method:
Expand source
result = All.GetBlocksOfIfStatements(true);
The result would consist of 2 items: the braces after if(a>3) (true block of
the if), and the braces after the else if (true block of the if).
Expand source
result = All.GetBlocksOfIfStatements(false);
This query ran on the same sample code would return the if(a>2) (false block of
the else in else if), and the braces after the else.
Supported from v8.5.0
GetBlocksOfIterationStatements
Returns a CxList with the blocks of all the iterations contained in the calling CxList.
Expand source
public CxList GetBranchesOfTernaryExpression(Boolean branch)
Returns a CxList containing all the blocks of the iterations in the calling CxList.
The return value may be empty (Count = 0). This command does not return a subset of the CxList, but a subset of All.
The following code example shows how you can use the GetBlocksOfIterationStatements method.
This example demonstrates the CxList.GetBlocksOfIterationStatements() method.
The input source code is:
Expand source
while (condition) { a = 1; } for (int i = 0; i<10; i++) { // code }
The source code that uses CxList.GetBlocksOfIterationStatements method:
Expand source
result = All.GetBlocksOfIterationStatements();
The result would consist of 2 item: the 2 opening braces in the code.
Supported from v8.5.0
GetBranchesOfTernaryExpressions
Returns a CxList with all the true/false branches of the ternary expressions inside the calling CxList according the provided boolean parameter.The true branch of a ternary expression is the value given if the condition is true, whereas the false block is the value given if the condition is false.
Expand source
public CxList GetBranchesOfTernaryExpression(Boolean branch)
branch A boolean describing weather you want to retrieve the true or false branches of the ternary expressions inside the calling CxList.
Returns a CxList containing all the true/false branches of the ternary expressions in the calling CxList.
ArgumentNullException : parameter is a null reference
The return value may be empty (Count = 0). This command does not return a subset of the CxList, but a subset of All.
The following code example shows how you can use the GetBranchesOfTernaryExpressions method.
This example demonstrates the CxList.GetBranchesOfTernaryExpressions(branch) method.
The input source code is:
Expand source
int a = someCondition ? 1:0;
The source code that uses CxList.GetBranchesOfTernaryExpressions method:
Expand source
result = All.GetBranchesOfTernaryExpressions(true);
The result would consist of 1 item: the number 1.
Expand source
result = All.GetBranchesOfTernaryExpressions(false);
The result would consist of 1 item: the number 0.
Supported from v8.5.0
GetByAncs
Returns all elements in this instance that is a CxDOM descendant of an element of the parameter.
Expand source
public CxList GetByAncs(CxList ancs)
ancs The Ancestors whose descendants are to be returned
Returns all elements in this instance that descends any of the elements in the parameter
The following code example shows how you can use the GetByAncs method.
This example demonstrates the CxList.GetByAncs() method.
The input source code is:
Expand source
public notMuch (boolean tf) { boolean localBoolean = tf; }
The source code that uses CxList.GetByAncs method:
Expand source
result = All.GetByAncs(All.FindByName("notMuch"));
The result would be -
Expand source
6 items found: notMuch boolean (in boolean tf) tf boolean localBoolean = tf (in localBoolean = tf)
Supported from v2.0.5
GetByBinaryOperator
Returns a CxList which is a subset of this instance and its elements are binary expressions with a given binary operator.
Expand source
public CxList GetByBinaryOperator(BinaryOperator opr)
opr Enum type of binary operators.
A subset of this instance with binary expressions which have a given binary operator.
ArgumentNullException : parameter is a null reference
The return value may be empty (Count = 0).
The following code example shows how you can use the GetByBinaryOperator method.
This example demonstrates the CxList.GetByBinaryOperator() method.
The input source code is:
Expand source
int i; if (i < 1) ...
The source code that uses CxList.GetByBinaryOperator method:
Expand source
result = All.GetByBinaryOperator(BinaryOperator.LessThan);
The result would be -
Expand source
1 item found - <
Supported from v1.8.1
GetByClass
Returns all elements in "this" instance that belong to any of the classes in the parameter.
Expand source
public CxList GetByClass(CxList classList);
classList The classes whose elements to be returned.
Returns all elements in this instance that belong to any of the classes in the parameter.
This example demonstrates the CxList.GetByClass() method.
The input source code is:
Expand source
class cl1 { void foo() { int a = 3; int b = 5; } } class cl2 { void foo2() { int c = 3; } }
The source code that uses CxList.GetByClass method:
Expand source
result = All.GetByClass(All.FindByName("*.cl1")).FindByName("3");
The result would consist of 1 item found:
Expand source
3 (in int a = 3)
Notice that 3 (in int c = 3) doesn't appear in the results, since it is not in the "cl1" class
GetByMethod
Returns all elements in this instance that belong to any of the methods in the parameter.
Expand source
public CxList GetByMethod(CxList methodList)
methodList The methods whose elements to be returned
Returns all elements in this instance that belong to any of the methods in the parameter
The following code example shows how you can use the GetByMethod method.
The input source code is:
Expand source
class cl1 { void foo() { int a = 3; int b = 5; } void foo2() { int c = 3; } }
The source code that uses CxList.GetByMethod method:
Expand source
result = All.GetByMethod(All.FindByName("foo2")).FindByName("3");
The result would be -
Expand source
1 item found 3 (in int c = 3)
Notice that 3 (in int a = 3) doesn't appear in the results, since it is not in the "foo2" method
Supported from v2.0.5
GetClass
Returns the classes of this instance containing the objects in the parameter.
Expand source
public CxList GetClass(CxList list)
list The elements whose classes to be returned
Returns the classes of this instance containing the objects in the parameter.
The following code example shows how you can use the GetClass method.
This example demonstrates the CxList.GetClass() method.
The input source code is:
Expand source
class cl1 { void foo() { int a = 3; int b = 5; } }
The source code that uses CxList.GetClass method:
Expand source
result = All.GetClass(All.FindByName ("5"));
The result would be found -
Expand source
1 item found: cl1 (in class cl1)
Supported from v2.0.5
GetCxListByPath
Create enumerator on CxList that enumerate on all existing paths.
Expand source
public abstract IEnumerable<CxList> GetCxListByPath(bool isSortFlows = false)
isSortFlows If true, Flows are sorted by NodeId to provide a consistent result
None
ArgumentNullException : parameter is a null reference
This example demonstrates the IEnumerable<CxList> GetCxListByPath() method.
Expand source
foreach (CxList thisCxList in this.GetCxListByPath()) { // thisCxList shall include one node and one path. If in “this” exists nodes without // paths than thisCxList will have only one node. }
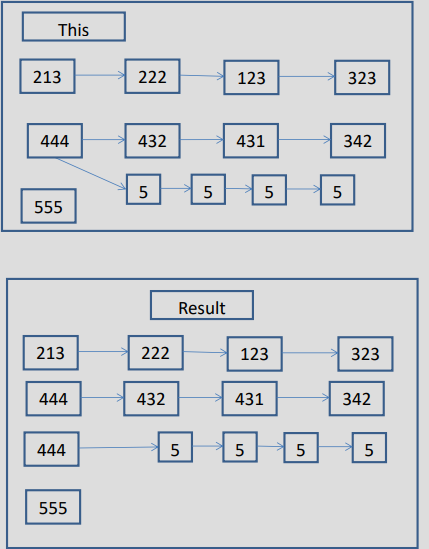
Supported from v7.1.3
GetDOMPropertiesOfFirst
This method implements DTO of CSharpGraph dom object. It returns DTO of first dom object in CxList. Important reason for this interface is to hide internal structure of CSharpGraph dom object.
Expand source
public DOMProperties GetDOMProperties()
A DTO of first element of the given CxList.
ArgumentNullException : parameter is a null reference
Supported from v9.2.0
GetDeclarationsDefinedBy
Returns declarations in the membersList defined by the members definitions contained in the membersDefinitionsList
membersList List of possible candidates to find members in
membersDefinitionsList List of definitions from which the members we want to find
None
GetElementByExpression
returns a CxList that holds the elements for a given expression
expressions the set of expressions to look from
CxList that will hold all elements in which the expression is located
GetEnumerator
Return IEnumerator of CxList.Data
Expand source
public IEnumerator GetEnumerator()
Enumerator of CxList.Data.
ArgumentNullException : parameter is a null reference
Not in use (deprecated). A simpler implementation is by:
Expand source
foreach (CxList cxItem in resultList) { ... }
This example demonstrates the GetEnumerator, cxLog.WriteDebugMessage and GetFirstGraph method.
The input source code is:
Expand source
class cl1 { void foo() { int a = 3; int b = 5; } }
This example demonstrates the GetEnumerator() method.
Expand source
IEnumerator ieNum = All.GetEnumerator(); bool finish = false; int i = 1; while (!finish) { if (!ieNum.MoveNext()) { finish = true; } else { CxList curr = (CxList)ieNum.Current; if (curr.GetFirstGraph() != null) { cxLog.WriteDebugMessage("#=" + i.ToString() + " curr name = " + curr.GetName() + " type = " + curr.GetFirstGraph().GraphType.ToString()); i++; } } }
The result would be on DebugMessage tab in CxAudit program:

Supported from v1.8.1
GetExpressionsByAttributes
Given an attribute CxList will find the expression that is linked to it
attributes the set of attributes
CxList that will hold the expressions that are the values of the given attributes
GetFathers
Returns a CxList which contains the direct fathers of the elements of "this" instance.
Expand source
public CxList GetFathers()
A CxList which contains the direct fathers of the element of "this" instance.
The return value may be empty (Count = 0).
This example demonstrates the CxList.GetFathers () method.
The input source code is:
Expand source
int b, a = 5; if (a > 3) b = 6;
The source code that uses CxList.GetFathers method:
Expand source
CxList six = All.FindByName("6"); result = six.GetFathers();
The result would consist of 1 item found:
Expand source
=
GetFinallyClause
Returns a CxList which is a subset of this instance and its elements are finally clauses of the specified CxList of try statements.
Expand source
public CxList GetFinallyClause (CxList TryList)
tryList CxList of try statements.
A subset of this instance with finally clauses.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0)
This example demonstrates the CxList.GetFinallyClause(CxList) method.
The input source code is:
Expand source
void foo() int j; try { int i = 0; j = 1 / i; } finally { j = 1; }
The source code that uses CxList.GetFinallyClause method:
Expand source
CxList Try = All.FindByType(typeof(TryCatchFinallyStmt)); result = All.GetFinallyClause(Try);
The result would consist of 1 item:
Expand source
finally
Supported from v1.8.1
GetFirstGraph
Returns a first data element in requested CxList. Using to get internal data of first object in requested CxList.
Expand source
public CSharpGraph GetFirstGraph()
A first element in Data. If CxList empty return null.
This example demonstrates using of CxList.GetFirstGraph() method.
Expand source
class cl1 { void foo() { int a = 3; int b = 5; } }
The source code that uses CxList.GetFirstGraph method:
Expand source
result = All.FindByShortName("foo"); if (result.Count > 0) cxLog.WriteDebugMessage(result.GetFirstGraph().ShortName);
The result would be on DebugMessage tab in CxAudit program:
Expand source
foo
Supported from v1.8.1
GetFirstNodesInPath
Returns CxList which is a subset of instance CxList and contains start nodes of path.
Expand source
public CxList GetFirstNodesInPath ()
Returns CxList which is a start nodes of path.
The return value may be empty (Count = 0).
This example demonstrates the CxList.GetFirstNodesInPath() method.
The input source code is:
Expand source
public void setString (String str) { lst.add(str); }
The source code that uses CxList.GetFirstNodesInPath method:
Expand source
CxList paths = All.DataInfluencingOn(All.FindByShortName("add")); // similar to paths.GetStartAndEndNodes(CxList.GetStartEndNodesType.StartNodesOnly); result = paths.GetFirstNodesInPath();
The result would consist of 2 items:
Expand source
lst (in lst.add(str);) str (in (String str);)
Supported from 9.4.0
GetFlow
get one path according its key (start*maxint + end)
Key None
None
GetFollowingStatements
Returns a CxList of the statements that are directly following all the statements of the calling CxList and in the same statement collection.
Expand source
public CxList GetFollowingStatements()
Returns a CxList containing all the statements that are following the statements in the calling CxList.
The return value may be empty (Count = 0). This command does not return a subset of the CxList, but a subset of All.
This example demonstrates the CxList.GetFollowingStatements() method.
The input source code is:
Expand source
if (a > 3) { a = 4; } if(a != 4) { a = 0; b = 5; } c = 2; d = 3;
The source code that uses CxList.GetFollowingStatements method:
Expand source
result = All.GetFollowingStatements();
The result would consist of 2 items:
Expand source
The assignment b=5 (following a=0), and d = 3(following c = 2). The assignment a=4 doesn't have any following statement in its scope.
Supported from v8.5.0
GetForwardDepthCountFlowEdge
This query helps investigate results that are missing due to the flow extending beyond the edge of our 'Depth Count'. The query returns the flow to nodes where future flow is cut off due to depth count (that is, the last reachable node). Hopefully, this will clearly show the 'edge' of the depth count.
GetHashCode
This method is to create the hashCode for each XML Node of XPathNavigator, as the inerent version from XPathNavigator was assigning the same hashCode to different nodes
input Provides a cursor model for navigating XML data
language Id of the language
depth Deapth of search
Unique HashCode
This method is to create the hashCode for each XML Node of XElement
input Represents a XML Element
language Id of the language
depth Deapth of search
Unique HashCode
This method uses the line info of the relevant input to create a new unique hashcode
inputLineInfo Line Info of relevant input
language Id of the language
depth Deapth of search
Unique HashCode
GetIndexOfParameter
For a single Param or ParamDecl returns the index of the parameter 0 based.
Expand source
public int GetIndexOfParameter ()
Integer containing the index of the parameter zero based, or -1 if not a parameter or list empty or contains multiple nodes.Note that the CxList must contain exactly one node, and the node must be of type Param or ParamDecl.
This example demonstrates the CxList.GetIndexOfParameterMethod() method.
It prints out to the log the index of each of the parameters.
Expand source
result = All.FindByType(typeof(Param));
GetLastNodesInPath
Returns CxList which is a subset of instance CxList and contains end nodes of path.
Expand source
public CxList GetLastNodesInPath ()
Returns CxList which is a end nodes of path.
The return value may be empty (Count = 0).
This example demonstrates the CxList.GetLastNodesInPath() method.
The input source code is:
Expand source
public void setString (String str) { lst.add(str); }
The source code that uses CxList.GetLastNodesInPath method:
Expand source
CxList paths = All.DataInfluencingOn(All.FindByShortName("add")); // similar to paths.GetStartAndEndNodes(CxList.GetStartEndNodesType.EndNodesOnly); result = paths.GetLastNodesInPath();
The result would consist of 2 items:
Expand source
add (in lst.add(str);)
Supported from 9.4.0
GetLeftmostTarget
Returns a CxList with the leftmost target of the specified member.
Expand source
public CxList GetLeftmostTarget()
A CxList with the leftmost target of a given member.
The return value may be empty (Count = 0).
These examples demonstrate the CxList.GetLeftmostTarget() method.
The input source code is:
Expand source
int i = foo().Bar().a.b;
The source code that uses CxList.GetLeftmostTarget method:
Expand source
CxList b = All.FindByName("b"); result = b.GetLeftmostTarget();
The result would consist of 1 item:
Expand source
foo
Supported from 8.0
GetLinePragmaKey
calc pragma key according to name,filename and line
nodeId None
None
GetMembersOfTarget
Returns a CxList with all found members of the specified target.
Expand source
public CxList GetMembersOfTarget()
A CxList with members of a given target
The return value may be empty (Count = 0).
These examples demonstrate the CxList.GetMembersOfTarget() method.
The input source code is:
Expand source
StreamWriter sw = new StreamWriter(); sw.Write("");
The source code that uses CxList.GetMembersOfTarget method:
Expand source
CxList sWriter = All.FindByType("StreamWriter"); result = sWriter.GetMembersOfTarget();
The result would consist of 1 item:
Expand source
write
Supported from 1.8.1
GetMembersWithTargets
Returns a CxList which is a subset of “this” instance with nodes that are part of a member/target pair (typical example: target.member) and have a direct target in the CxList parameter "targets".
Expand source
public CxList GetMembersWithTargets(CxList targets)
targets CxList of DOM objects which might be the target(s) of elements in "this".
Returns a CxList which is a subset of “this” instance with nodes that have a direct target in "targets" parameter.
The return value may be empty (Count = 0). If targets is null – returns an empty CxList.
These examples demonstrate the CxList.GetMembersWithTargets(CxList) method.
The input source code is:
Expand source
int num = 55; string Str = num.ToString().ToUpper().PadLeft(5, ' ');
The source code that uses CxList.GetMembersWithTargets method:
Expand source
CxList methods = All.FindByType(typeof(MethodInvokeExpr)); CxList num = All.FindByShortName("num"); result = methods.GetMembersWithTargets(num);
The result would consist of 1 item:
Expand source
ToString in num.ToString().ToUpper().PadLeft(5, ' ');
Supported from 1.8.1
Returns a CxList which is a subset of “this” instance with nodes that are part of a member/target pair (typical example: target.member) and have a direct target in "targets" parameter, or a target of target, a target of a target of a target …. Up to depthLimit depth.
Expand source
public CxList GetMembersWithTargets(CxList targets, int depthLimit)
targets CxList of DOM objects which might be the target(s) of elements in "this", or the target of a target of this.
depthLimit The number of iterations to look for targets.
Returns a CxList which is a subset of “this” instance with nodes that have a direct target.
The return value may be empty (Count = 0). If targets is null – returns an empty CxList.
These examples demonstrate the CxList.GetMembersWithTargets(CxList, int) method.
The input source code is:
Expand source
int num = 55; string Str = num.ToString().ToUpper().PadLeft(5, ' ');
The source code that uses CxList.GetMembersWithTargets method:
Expand source
CxList methods = All.FindByType(typeof(MethodInvokeExpr)); CxList num = All.FindByShortName("num"); result = methods.GetMembersWithTargets(num, 2);
The result would consist of 1 item:
Expand source
ToString, ToUpper in num.ToString().ToUpper().PadLeft(5, ' ');
Supported from 1.8.1
Returns a CxList which is a subset of “this” instance with nodes that are part of a member/target pair (typical example: target.member) and have a direct target(i.e.they are the member).
Expand source
public CxList GetMembersWithTargets()
Returns a CxList which is a subset of “this” instance with nodes that have a direct target.
The return value may be empty (Count = 0).
These examples demonstrate the CxList.GetMembersWithTargets() method.
The input source code is:
Expand source
int num = 55; string Str = num.ToString().ToUpper().PadLeft(5, ' ');
The source code that uses CxList.GetMembersWithTargets method:
Expand source
CxList methods = All.FindByType(typeof(MethodInvokeExpr)); result = methods.GetMembersWithTargets();
The result would consist of 3 items:
Expand source
PadLeft, ToUpper, ToString in num.ToString().ToUpper().PadLeft(5, '');
Supported from 1.8.1
GetMethod
Returns CxList which is a subset of this instance and its elements are methods of the specified CxList.
Expand source
public CxList GetMethod(CxList list)
list CxList of any DOM objects.
A subset of this instance which contains methods of the specified CxList.
ArgumentNullException : Thrown when parameter is a null reference.
This example demonstrates the CxList.GetMethod(CxList) method.
The input source code is:
Expand source
Class Cl { void foo() { int i = 1; i++; } }
The source code that uses CxList.GetMethod method:
Expand source
CxList I_var = All.FindByShortName("i"); result = All.GetMethod(I_var);
The result would consist of 1 item:
Expand source
foo
Supported from v1.8.1
GetName
Returns a first data element name in requested CxList. Using to get internal data of first object in requested CxList.
Expand source
public string GetName()
A name of the first element in Data. If CxList empty return null.
The return value may be empty (Count = 0).
This example demonstrates the CxList.GetName() method.
The input source code is:
Expand source
class cl1 { void foo() { int a = 3; int b = 5; } }
The source code that uses CxList.GetName method:
Expand source
result = All.FindByShortName("foo"); if (result.Count > 0) cxLog.WriteDebugMessage(result.GetName());
The result would be on DebugMessage tab in CxAudit program:
Expand source
foo
Supported from v1.8.1
GetNextString
Next token or null if no more tokens are available.
GetNonDuplicateNodes
Gets the non duplicate nodes.
origin The origininal CxList
May return null if origin is null
GetNumberOfPaths
return number of paths
None
GetOneNodePathsNumber
get number of paths that the input node is thier root
rootVertex None
None
GetParameters
Returns a CxList which is a subset of this instance and its elements are parameters of methods elements provided in CxList.
Expand source
public CxList GetParameters (CxList MethodsList)
methodsList CxList of methods.
Returns a CxList with all the parameters, from instance CxList, of the methods in MethodsList.
ArgumentNullException : Thrown when parameter is a null reference.
This example demonstrates the CxList.GetParameters(CxList) method.
The input source code is:
Expand source
foo(1, 3, i);
The source code that uses CxList.GetParameters method:
Expand source
CxList methods = All.FindByType(typeof(MethodInvokeExpr)); result = All.GetParameters(methods);
The result would consist of 3 items:
Expand source
1, 3, i
Supported from v1.8.1
Returns a CxList which is a subset of instance CxList and its elements are parameters of methods elements provided in CxList. The index integer is the position of the desired parameter.The direction refers to the index direction. If forward, 0 is the first parameter.If backwards, 0 is the last parameter.
Expand source
public CxList GetParameters (CxList MethodsList, int paramNo, ParameterIndexDirection direction)
methodsList CxList of methods.
paramNo The number of parameter to return (begins with 0).
direction The direction for the parameter position. Can be ParameterIndexDirection.Forward or ParameterIndexDirection.Backward
Returns a CxList with paramNo parameters, from instance CxList, of the methods in MethodsList.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrates the CxList.GetParameters(CxList, int, ParameterIndexDirection) method.
The input source code is:
Expand source
foo(1, 3, i);
The source code that uses CxList.GetParameters method:
Expand source
CxList methods = All.FindByType<MethodInvokeExpr>(); result = All.GetParameters(methods, 0, CxList.ParameterIndexDirection.Backward);
The result would consist of 1 items:
Expand source
1
Supported from v9.5.4
GetPathsOrigins
Returns a CxList which is a subset of instance CxList and contains end nodes of paths.
Expand source
public CxList GetPathsOrigins ()
Returns CxList that contains end nodes of paths.
The return value may be empty (Count = 0).
This example demonstrates the CxList.GetPathsOrigins() method.
The input source code is:
Expand source
public void setString (String str) { if (str.length >0) { lst.add(str); } }
The source code that uses CxList.GetPathsOrigins method:
Expand source
CxList paths = All.DataInfluencingOn(All.FindByShortName("add")); result = paths.GetPathsOrigins();
The result would consist of 3 items:
Expand source
lst (in lst.add(str);) str(in lst.add(str);) str(in (String str);)
GetQueryParam
Try to get a value for a query parameter using the key paramName. Returns an empty string if the key was not found and on errors.
Expand source
public string GetQueryParam(string paramName)
paramName The parameter name (key)
The value of the received key, or an empty string if the key was not found.
The source code that uses CxList.GetQueryParam method:
Expand source
string val = All.GetQueryParam("Param"); // If the key "Param" was found in the configuration, than val now holds its value, otherwise, val is an empty string if (string.IsNullOrEmpty(val)) { // Use val }
Try to get a value for a query parameter using the key paramName and parse the returned string value to type T. Returns defaultVal if the key was not found and on errors.
Expand source
public string GetQueryParam<T>(string paramName, T defaultVal = default(T))
T The type of defaultVal and the returned value.
paramName The parameter name (key).
defaultVal The value to return on errors.
The value for the received key parsed to type T, or defaultVal if the key was not found or if the value returned cannot be parsed to type T.
ArgumentNullException : Thrown when parameter is a null reference.
This example demonstrates the CxList.GetQueryParam<T>(string, T) method.
Expand source
int val = All.GetQueryParam<int>("Param", 0); // If the key "Param" was found in the configuration, than val now holds its value, otherwise, val is 0 if (val > 0) { // Use val }
Supported from 8.4.0
GetRightmostMember
Returns a CxList with the rightmost members of the specified target.
Expand source
public CxList GetRightmostMember()
A CxList with the rightmost members of a given target.
The return value may be empty (Count = 0).
These examples demonstrate the CxList.GetRightmostMember() method.
The input source code is:
Expand source
int i = foo().Bar().a.b;
The source code that uses CxList.GetRightmostMember method:
Expand source
CxList foo = All.FindByName("foo"); result = foo.GetRightmostMember();
The result would consist of 1 item:
Expand source
b
Supported from 8.0
GetRootVertexes
get list of root vertexes in DOM units
None
GetSanitizerByMethodInCondition
Internal use only
For each input method, finds all the calls inside a "if" condition and returns all the references, of the methods parameters, that are inside the "if" statement.
Expand source
public CxList GetSanitizerByMethodInCondition(CxList MethodCallsInCondition)
methodCallsInCondition method call list inside "if" condition (must be of type MethodInvoke)
all references of a method call parameter in the scope of the if statement
This example demonstrates the CxList.GetSanitizerByMethodInCondition(CxList
MethodCallsInCondition) method.
The input source code is:
Expand source
String a = getInput(); if(Valid(a)) { Print(a); }
The source code that uses GetSanitizerByMethodInCondition method:
Expand source
CxList valid = All.FindByShortName("Valid"); result = All.GetSanitizerByMethodInCondition(valid);
The result would consist of 1 item:
Expand source
a (in Print(a))
The purpose of the query is to mark 'a' as a sanitizer, because the flow doesn't
pass through the condition.
Supported from 7.1.2
Internal use only
For each method, finds all the calls inside a "if" condition and returns all the references, of the methods parameters, that are inside the "if" block(the IfBlock input parameter) statement.
Expand source
public CxList GetSanitizerByMethodInCondition(CxList MethodCallsInCondition, IfBlock block)
methodCallsInCondition method call list inside "if" condition (must be of type MethodInvoke)
block select only "true", only "false" or both scopes.
all references of a method call parameter in the scope of the if statement
This example demonstrates the CxList.GetSanitizerByMethodInCondition(CxList
MethodCallsInCondition, IfBlock block) method.
The input source code is:
Expand source
String a = getInput(); if(Valid(a)) { Print(a); }
The source code that uses GetSanitizerByMethodInCondition method:
Expand source
CxList valid = All.FindByShortName("Valid"); result = All.GetSanitizerByMethodInCondition(valid,CxList.IfBlock.True);
The result would consist of 1 item:
Expand source
a (in Print(a))
The purpose of the query is to mark 'a' as a sanitizer, because the flow doesn't
pass through the condition.
Supported from 7.1.2
GetScanProperty
Returns string with a value of requested property.
Expand source
public string GetScanProperty (string key)
key Name of requested property, currently only projectPath property supported.
If requested property exists -> value of requested property will be returned.
Otherwise empty string will be returned.
None
Expand source
string projectPath = cxScan.GetScanProperty("projectPath"); // string projectPath contains value of “projectPath” property
Supported from 8.6.0
GetSortedCxListById
Returns the CxList sorted by Id.
None
GetStartAndEndNodes
Returns CxList which is a subset of instance CxList and contains start nodes or end nodes or both start and nodes of path or all nodes in path.
Expand source
public CxList GetStartAndEndNodes (GetStartEndNodesType type)
requestedType The type of nodes to be returned: CxList.GetStartEndNodesType.StartNodesOnly, CxList.GetStartEndNodesType.EndNodesOnly, CxList.GetStartEndNodesType.StartAndEndNodes, CxList.GetStartEndNodesType.AllNodes.
Returns CxList which is a start nodes or end nodes or both start and nodes of path or all nodes in path.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrates the CxList.GetStartAndEndNodes(GetStartEndNodesType) method.
The input source code is:
Expand source
public void setString (String str) { lst.add(str); }
Expand source
CxList paths = All.DataInfluencingOn(All.FindByShortName("add")); result = paths.GetStartAndEndNodes(CxList.GetStartEndNodesType.StartNodesOnly);
The result would consist of 2 items:
Expand source
lst (in lst.add(str);) str (in (String str);)
Expand source
CxList paths = All.DataInfluencingOn(All.FindByShortName("add")); result = paths.GetStartsAndEndNodes(CxList.GetStartEndNodesType.EndNodesOnly);
The result would consist of 1 item:
Expand source
lst (in lst.add(str);)
Expand source
CxList paths = All.DataInfluencingOn(All.FindByShortName("add")); result = paths.GetStartAndEndNodes(CxList.GetStartEndNodesType.StartAndEndNodes);
The result would consist of 3 items:
Expand source
lst (in lst.add(str);) str (in (String str);) add (in lst.add(str);)
Expand source
CxList paths = All.DataInfluencingOn(All.FindByShortName("add")); result = paths.GetStartAndEndNodes(CxList.GetStartEndNodesType.AllNodes);
The result would consist of 4 items:
Expand source
lst (in lst.add(str);) str (in (String str);) add (in lst.add(str);) str (in lst.add(str);)
Expand source
CxList paths = All.DataInfluencingOn(All.FindByShortName("add")); result = paths.GetStartAndEndNodes(CxList.GetStartEndNodesType.AllButNotStartAndEnd);
The result would consist of 2 items:
Expand source
lst (in lst.add(str);) str (in lst.add(str);)
Supported from 7.1.2
GetStartEndVertexes
get list of keys that includes start and end (key = start * maxint + end
None
GetStartOrEndPaths
get dictionary of paths sorted by start or end nodeId
firstNode None
None
GetStartOrEndVertexes
get list of start or end or (start+end) vertexes
GetTargetOfMembers
Returns the list of elements which are the targets from the members of “this” instance.
Expand source
public CxList GetTargetOfMembers()
A list of objects from which “this” instance elements are member of.
These examples demonstrate the CxList.GetTargetOfMembers() method.
The input source code is:
Expand source
class cl1 { void foo() { int a = obj.func(); } }
The source code that uses CxList.GetMembersWithTargets method:
Expand source
result = All.FindByName("*.func").GetTargetOfMembers();
The result would consist of 1 item:
Expand source
obj (in int a = obj.func())
GetTargetsWithMembers
Return a subset of 'this' that have a direct member in 'members'
Expand source
public CxList GetTargetsWithMembers(CxList members)
members members - CxList of DOM objects which might be the member(s) of elements in "this"
Returns a CxList which is a subset of “this” instance with nodes that have a direct member in members parameter.
The return value may be empty (Count = 0). If members is null – returns an empty CxList
The following code example shows how you can use the GetTargetsWithMembers method.
This example demonstrates the CxList.GetTargetsWithMembers() method.
The input source code is:
Expand source
int num = 55; string Str = num.ToString().ToUpper().PadLeft(5, ' ');
The source code that uses CxList.GetTargetsWithMembers method:
Expand source
CxList methods = All.FindByType(typeof(MethodInvokeExpr)); CxList member = All.FindByShortName("PadLeft"); result = methods.GetTargetsWithMembers(member);
The result would be -
Expand source
1 item found: ToUpper in num.ToString().ToUpper().PadLeft(5, ' ');
Supported from v1.8.1
Returns a CxList which is a subset of "this" instance with nodes that are part of a member/target pair (typical example: target.member), and have a direct member in CxList parameter "members", or a member of a member… up to depth depthLimit
Expand source
public CxList GetTargetsWithMembers(CxList targets, int depthLimit)
members CxList of DOM objects which might be the member(s) of elements in "this", or the member of member of this, …
depthLimit The number of iterations to look for members
Returns a CxList which is a subset of “this” instance with nodes that have a direct /chain member.
The return value may be empty (Count = 0). If members is null – returns an empty CxList
The following code example shows how you can use the GetTargetsWithMembers method.
This example demonstrates the CxList.GetTargetsWithMembers() method.
The input source code is:
Expand source
string Str = "sample".ToString().ToUpper().PadLeft(5, ' ');
The source code that uses CxList.GetTargetsWithMembers method:
Expand source
CxList methods = All.FindByType(typeof(MethodInvokeExpr)); CxList member = All.FindByShortName("PadLeft"); result = methods.GetTargetsWithMembers(member, 2);
The result would be -
Expand source
2 items found ToString, ToUpper in num.ToString().ToUpper().PadLeft(5, ' ');
Supported from v1.8.1
Returns a CxList which is a subset of “this” instance with nodes that are part of a member/target pair (typical example: target.member) and have a direct member(i.e.they are the target).
Expand source
public CxList GetTargetsWithMembers()
Returns a CxList which is a subset of “this” instance with nodes that have a direct member.
The return value may be empty (Count = 0).
These examples demonstrate the CxList.GetTargetsWithMembers() method.
The input source code is:
Expand source
int num = 55; string Str = num.ToString().ToUpper().PadLeft(5, ' ');
The source code that uses CxList.GetMembersWithTargets method:
Expand source
CxList methods = All.FindByType(typeof(MethodInvokeExpr)); result = methods.GetTargetsWithMembers();
The result would consist of 2 items:
Expand source
ToUpper, ToString in num.ToString().ToUpper().PadLeft(5, ' ');
Supported from v1.8.1
GetTextNodesExpressions
Creates a CxList that will hold all the expressions per framework that are located in a text node
xmlFilterFiles the extensions to look in
framework the name of the framework
language the language
CxList of expressions for a given framework located in text nodes
GetXMLNodeDescendents
Get Descendent expressions located under origin nodes DOM wise out of group of given expressions
originNodes the ancestors
descendentExpressionGroup the group of descendents to look from
a subset of the descendentExpressionGroup that is a descendent of originNodes
GetXmlFiles
Returns an enumerated list of XML files filtered according to the first parameter.
Expand source
public IEnumerable<CxXmlDoc> GetXmlFiles (string filter, bool IgnoreNamespaces = false)
filter File extension pattern to be used as filter.
IgnoreNamespaces Specifies whether the search should ignore the namespaces. This field is not required and its default value is false.
Returns an enumerated list of XML files.
None
Expand source
IEnumerable xmlDoc = cxXpath.GetXmlFiles("*.cx", true); // Returns an enumerated list of XML files filtered by "*.cx"
Supported from 8.6.0
InfluencedBy
Returns a CxList which is a subset of this instance and its elements are influenced (either data or control) by the CxList specified in the first parameter using the influence algorithm specified in the second parameter.
Expand source
public CxList InfluencedBy(CxList influencing, InfluenceAlgorithmCalculation algorithm)
list ICxListProvider data-influencing on this instance.
currAlg Influence algorithm to use. An enum matching the relevant options which are: OldAlgorithm, NewAlgorithm
A subset of this instance influenced by (either data or control) the specified CxList.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrates the CxList.InfluencedBy() method.
Notice the difference between DataInfluencedBy and InfluencedBy
The input source code is:
Expand source
void main() { int b = 2, a = 5, c; if (a > b) b = 3; c = b; }
The source code that uses CxList.InfluencedBy method:
Expand source
result = All.InfluencedBy(All.FindById(43), CxList.InfluenceAlgorithmCalculation.NewAlgorithm); // Id 43 is 5 from a = 5; // Notice that among all the results also c (in c = b) appears because c is data-dependant on b=3, which in turn is control dependant on a > b, which itself is data-dependant on a = 5. result = All.DataInfluencedBy(All.FindById(43)); // 5 // Notice that now c (in c = b) doesn't appear because its value is not influenced by 5.
Supported from 7.1.2
Returns a CxList which is a subset of this instance and its elements are influenced (either data or control) by the CxList specified in parameter. This call is equivalent to the following calls and it is recommended to use the short call format by default: - InfluencedBy(list, InfluenceAlgorithmCalculation.OldAlgorithm)
Expand source
public CxList InfluencedBy(CxList list)
list CxList data-influencing on this instance.
A subset of this instance influenced by (either data or control) the specified CxList.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrates the CxList.InfluencedBy() method.
Notice the difference between DataInfluencedBy and InfluencedBy
The input source code is:
Expand source
void main() { int b = 2, a = 5, c; if (a > b) b = 3; c = b; }
The source code that uses CxList.InfluencedBy method:
Expand source
result = All.InfluencedBy(All.FindById(43)); // Id 43 is 5 from a = 5; // Notice that among all the results also c (in c = b) appears because c is data-dependant on b=3, which in turn is control dependant on a > b, which itself is data-dependant on a = 5. result = All.DataInfluencedBy(All.FindById(43)); // 5 // Notice that now c (in c = b) doesn't appear because its value is not influenced by 5.
Supported from 7.1.2
InfluencedByAndNotSanitized
Returns a CxList which is a subset of this instance and its elements are influenced by the CxList specified in the first parameter, and their influencing path doesn't contain elements from the CxList specified in the second parameter, using the influence algorithm specified in the third parameter.
Expand source
public CxList InfluencedByAndNotSanitized(CxList influencing, CxList sanitization, InfluenceAlgorithmCalculation algorithm)
red CxList influencing on this instance.
green CxList that cuts the influencing path
currAlg Influence algorithm to use. An enum matching the relevant options which are: OldAlgorithm, NewAlgorithm
A subset of this instance and its elements are influenced by the first specified parameter, and their influencing path doesn't contain element from the second CxList.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrates the CxList.InfluencedByAndNotSanitized() method.
The input source code is:
Expand source
string s = input(); string s1 = fixSql(s); string s2 = s + s1; execute(s); (*) execute(s1); execute(s2); (*) s = s1; execute(s); execute(s1); execute(s2); (*) s2 = s; execute(s); execute(s1); execute(s2);
The source code that uses CxList.InfluencedByAndNotSanitized method:
Expand source
CxList execute = All.FindByName("execute"); CxList input = All.FindByName("input"); CxList fixSql = All.FindByName("fixSql"); result = execute.InfluencedByAndNotSanitized(input, fixSql, CxList.InfluenceAlgorithmCalculation.NewAlgorithm);
Notice that only the lines marked with a * are returned. These are the
only statements that have an influencing path from the input() command,
without being completely sanitized by fixSql().
Supported from 7.1.2
Returns a CxList which is a subset of this instance and its elements are influenced by the CxList specified in the first parameter, and their influencing path doesn't contain elements from the CxList specified in the second parameter. This call is equivalent to the following calls and it is recommended to use the short call format by default: - InfluencedByAndNotSanitized(influencing, sanitized, InfluenceAlgorithmCalculation.OldAlgorithm)
Expand source
public CxList InfluencedByAndNotSanitized(CxList influencing, CxList sanitization)
red CxList influencing on this instance.
green CxList that cuts the influencing path
A subset of this instance and its elements are influenced by the first specified parameter, and their influencing path doesn't contain element from the second CxList.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrates the CxList.InfluencedByAndNotSanitized() method.
The input source code is:
Expand source
string s = input(); string s1 = fixSql(s); string s2 = s + s1; execute(s); (*) execute(s1); execute(s2); (*) s = s1; execute(s); execute(s1); execute(s2); (*) s2 = s; execute(s); execute(s1); execute(s2);
The source code that uses CxList.InfluencedByAndNotSanitized method:
Expand source
CxList execute = All.FindByName("execute"); CxList input = All.FindByName("input"); CxList fixSql = All.FindByName("fixSql"); result = execute.InfluencedByAndNotSanitized(input, fixSql); Notice that only the lines marked with a (*) are returned. These are the only statements that have an influencing path from the input() command, without being completely sanitized by fixSql().
Supported from 7.1.2
InfluencingOn
Returns a CxList which is a subset of this instance and its elements are influencing (data and control) on the CxList specified in the first parameter using the influence algorithm specified in the second parameter.
Expand source
public CxList InfluencingOn (CxList influenced, InfluenceAlgorithmCalculation algorithm)
list CxList influenced by this instance.
currAlg Influence algorithm to use. An enum matching the relevant options which are: OldAlgorithm, NewAlgorithm
A subset of this instance influencing on the specified CxList.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrates the CxList.InfluencingOn() method´.
The input source code is:
Expand source
int a; a = 5; b = a;
The source code that uses CxList.InfluencingOn method:
Expand source
CxList b_var = All.FindByShortName("b"); result = All.InfluencingOn(b_var, CxList.InfluenceAlgorithmCalculation.NewAlgorithm);
The result would be:
Expand source
3 items found: 5, a, a
Supported from 7.1.2
Returns a CxList which is a subset of this instance and its elements are influencing (data and control) on the CxList specified in parameter. This call is equivalent to the following calls and it is recommended to use the short call format by default: - InfluencingOn(influenced, InfluenceAlgorithmCalculation.OldAlgorithm)
Expand source
public CxList InfluencingOn (CxList influenced)
list CxList influenced by this instance.
A subset of this instance influencing on the specified CxList.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrates the CxList.InfluencingOn() method´.
The input source code is:
Expand source
int a; a = 5; b = a;
The source code that uses CxList.InfluencingOn method:
Expand source
CxList b_var = All.FindByShortName("b"); result = All.InfluencingOn(b_var);
The result would be:
Expand source
3 items found: 5, a, a
Supported from 7.1.2
InfluencingOnAndNotSanitized
Returns a CxList which is a subset of this instance and its elements are influencing on (Data or Control), and an influencing path exists which doesn't contain elements from the sanitization using the influence algorithm specified in the third parameter.
Expand source
public CxList InfluencingOnAndNotSanitized(CxList influencing, CxList sanitization, InfluenceAlgorithmCalculation algorithm)
red CxList influencing on this instance.
green CxList that cuts the influencing path
currAlg An enum matching the relevant InfluenceAlgorithmCalculation options which are: OldAlgorithm, NewAlgorithm
A subset of this instance and its elements are influencing on the first specified parameter, and their influencing path doesn't contain elements from the CxList specified in the second parameter.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrates the CxList.InfluencedByAndNotSanitized() method.
The input source code is:
Expand source
string s = input(); string s1 = fixSql(s); string s2 = s + s1; execute(s); (*) execute(s1); execute(s2); (*) s = s1; execute(s); execute(s1); execute(s2); (*) s2 = s; execute(s); execute(s1); execute(s2);
The source code that uses CxList.InfluencedByAndNotSanitized method:
Expand source
CxList execute = All.FindByName("execute"); CxList input = All.FindByName("input"); CxList fixSql = All.FindByName("fixSql"); result = input.InfluencingOnAndNotSanitized(execute, fixSql, CxList.InfluenceAlgorithmCalculation.NewAlgorithm);
Notice that only the first line is returned (string s = input(); )
This query would return 1 result with a path from p declaration to the Console.WriteLine,
passing through the MemberAccess p.X
Supported from 7.1.2
Returns a CxList which is a subset of this instance and its elements are influencing on (Data or Control), and an influencing path exists which doesn't contain elements from the sanitization. This call is equivalent to the following calls and it is recommended to use the short call format by default: - InfluencingOnAndNotSanitized(influencing, sanitized, InfluenceAlgorithmCalculation.OldAlgorithm)
Expand source
public CxList InfluencingOnAndNotSanitized(CxList influencing, CxList sanitization)
red CxList influencing on this instance.
green CxList that cuts the influencing path
A subset of this instance and its elements are influencing on the first specified parameter, and their influencing path doesn't contain elements from the CxList specified in second parameter.
ArgumentNullException : Thrown when parameter is a null reference.
The return value may be empty (Count = 0).
This example demonstrates the CxList.InfluencedByAndNotSanitized() method.
The input source code is:
Expand source
string s = input(); string s1 = fixSql(s); string s2 = s + s1; execute(s); (*) execute(s1); execute(s2); (*) s = s1; execute(s); execute(s1); execute(s2); (*) s2 = s; execute(s); execute(s1); execute(s2);
The source code that uses CxList.InfluencedByAndNotSanitized method:
Expand source
CxList execute = All.FindByName("execute"); CxList input = All.FindByName("input"); CxList fixSql = All.FindByName("fixSql"); result = input.InfluencingOnAndNotSanitized(execute, fixSql);
Notice that only the first line is returned (string s = input(); )
Supported from 7.1.2
InfluencingOnBackwardSearchDisplayAllNodes
By default, the lazy flow won't show some of the nodes, since these nodes only make the flow longer and less clear. You can use this query if you want to see all the nodes.
InfluencingOnBackwardSearchGetLongPaths
By default, the lazy flow always returns the shortest path between two dots. This query returns the longest path between those nodes.This can be very useful when investigating performance issues..
InfluencingOnForwardSearchDisplayAllNodes
By default, the lazy flow won't show some of the nodes, since these nodes only make the flow longer and less clear. You can use this query if you want to see all the nodes.
InfluencingOnForwardSearchGetLongPaths
By default, the lazy flow always returns the shortest path between two dots. This query returns the longest path between those nodes.This can be very useful when investigating performance issues..
InheritsFrom
Returns a CxList which is a subset of "this" instance and its elements are inherited from the given class name.
Expand source
public CxList InheritsFrom(string baseClassName)
baseClassName The name of the base class.
A subset of "this" instance which elements are inherited from the given base class name.
ArgumentNullException : parameter is a null reference
This example demonstrates the CxList.InheritsFrom() method.
The input source code is:
Expand source
class BClass { } class CClass : BClass { }
The source code that uses CxList.InheritsFrom method:
Expand source
result = All.InheritsFrom("BClass");
The result would consist of 1 item:
Expand source
CClass
Supported from v1.8.1
Returns a CxList which is a subset of “this” instance and its elements are inherited from the given CxList of classes.
Expand source
public CxList InheritsFrom(CxList baseClassList)
baseClassList The CxList of base classes.
A subset of "this" instance which elements are inherited from the given base classes.
ArgumentNullException : parameter is a null reference
The return value may be empty (Count = 0).
This example demonstrates the CxList.InheritsFrom() method.
The input source code is:
Expand source
class BClass { } class CClass : BClass { }
Expand source
CxList cl = All.FindByName("BClass"); result = All.InheritsFrom(cl);
The result would consist of 1 item:
Expand source
CClass
Supported from v1.8.1
Returns a CxList which is a subset of this instance and its elements are inherits from the specified CxList of classes.
baseClassNames List of base classes.
A subset of this instance inherits from specified base classes.
Returns a CxList which is a subset of this instance and its elements are inherits from the specified List of classes.
Expand source
public CxList InheritsFrom(params string[] baseClassNames)
baseClassNames List of the base classes.
inheritsFromAll By default is false. The instance should inherit from all list baseClassNames, works at the first level of inheritance.
A subset of this instance inherits from specified base classes.
The return value may be empty (Count = 0).
This example demonstrates the CxList.InheritsFrom() method.
The input source code is:
Expand source
class BClass { } class AClass { } class CClass : BClass { } class DClass : BClass, AClass { }
The source code that uses CxList.InheritsFrom method:
Expand source
result = All.InheritsFrom(new List<string>{"BClass"});
The result would consist of 2 items:
Expand source
CClass, DClass
Expand source
result = All.InheritsFrom(new List<string>{"BClass", “AClass”});
The result would consist of 1 item:
Expand source
DClass
Supported from v9.4
Intersect
return all paths that present in this and in second
second None
None
calculate intersect of this flow and data2 flow
data2 None
None
IntersectWithNodes
create new PathData with paths that contains node in input parameter
intersectNodes in DFG format
None
Returns a CxList which is a subset of paths, which are the instance CxList, that includes elements of intersected CxList.
Expand source
public CxList IntersectWithNodes (CxList intersectData, IntersectionType type)
intersectData None
type The type of intersection to be made: CxList.IntersectionType.AllNodes CxList.IntersectionType.AnyNodes(default)
Returns a CxList which is a subset of the "this" instance , that includes at least one element of intersected CxList when the type is "AnyNodes", and includes all elements of intersected CxList when the type is "Allnodes".
ArgumentNullException : parameter is a null reference
The return value may be empty (Count = 0).
This example demonstrates the CxList.IntersectWithNodes() method.
The input source code is:
Expand source
public void setString (String str) { if (str.length >0) { lst.add(str); } else { String otherStr = "string is empty"; lst.add(otherStr); } }
The source code that uses CxList.IntersectWithNodes method:
Expand source
CxList intersect = All.FindByShortName("otherStr"); intersect.Add(All.FindByType(typeof(StringLiteral))); CxList paths = All.DataInfluencingOn(All.FindByShortName("lst")); result = paths.IntersectWithNodes(intersect, CxList.IntersectionType.AllNodes);
The result would consist of 1 item:
a flow starting on "string is empty", passing on the 2 occurrences of
"otherStr" and the "add" method, and ending on the "lst" UnknownReference.
Supported from 9.1.0
IsApexCommentLanguage
Tests if the a given file is written in APEX language comment: // and /* */
curFile The file to be tested
A boolean indicating if the given file is from one of the languages listed below (vb-based comment)
IsCobolLanguage
Tests if the a given file is written in a COBOL comment language: *...
curFile The file to be tested
A boolean indicating if the given file is a Cobol one
IsEmpty
return true if no path exists
None
IsFrameworkActive
Returns bool if requested framework present in scaned project..
Expand source
public bool IsFrameworkActive (string frameworkName)
key Name of requested framework
True -> requested framework present in this project.
False -> othewise
Implementation of query JavaScript Kony_Code_Injection
Expand source
if(cxScan.IsFrameworkActive("Kony")) { CxList inputs = Kony_UI_Inputs(); CxList Eval = Find_Outputs_CodeInjection(); CxList sanitize = Code_Injection_Sanitize(); and etc. } // If "Kony" framework present -> this query do something, otherwise do nothing // You can test existing of every framework.
Supported from 8.6.0
IsHashedCommentLanguage
Tests if the a given file is written in a hashed-comment language: #...
curFile The file to be tested
A boolean indicating if the given file is from one of the languages listed below (hash-comment based)
IsSQLCommentLanguage
Tests if the a given file is written in sql language comment: '
curFile The file to be tested
A boolean indicating if the given file is from one of the languages listed below (sql-based comment)
IsVbCommentLanguage
Tests if the a given file is written in vb language comment: '
curFile The file to be tested
A boolean indicating if the given file is from one of the languages listed below (vb-based comment)
IsXmlCommentLanguage
Tests if the a given file is written in a xml based language comment:
curFile The file to be tested
A boolean indicating if the given file is from one of the languages listed below (xml-comment based)
LineDicPragmaComparer
A comparer function for the LinePragma class - for use with Dictionary
LinePragmaComparer
A comparer function for the LinePragma class - for use with SortedList
Minus
return "minus" of this - second
second None
None
calculate this from minus parameter flow
data2 None
None
NewCxList
Create new empty CxList.
Expand source
public CxList NewCxList()
New CxList.
This example demonstrates the CxList.NewCxList() method.
The input source code is:
Expand source
int b, a = 5; if (a == 33) b = 6;
The source code that uses CxList.NewCxList method:
Expand source
CxList list_a = All.NewCxList(); list_a.Add(All.FindByName(“A”)); CxList list_b = All.FindByName(“b”); list_a.Add(list_b); result = list_a;
The resulting list will contain 4 elements.
NotInfluencedBy
Returns a ICxListProvider which is a subset of this instance and its elements are not influenced (either data or control) by the specified ICxListProvider.
list ICxListProvider data on this instance.
A subset of this instance not influenced by (either data or control) the specified ICxListProvider.
NotInfluencingOn
Returns a ICxListProvider which is a subset of this instance and its elements are not influencing (data and control) on the specified ICxListProvider
list ICxListProvider data on this instance.
A subset of this instance not influencing on the specified ICxListProvider.
OneNodePath
class to represent flow from one root
OneReducePath
internal representation of one flow()
PathData
this class is inteface od paths collection of one Cxlist
Plus
return union of two "OneNodeFlow"
second None
pathComperer None
None
calculate union of this flow and data2 flow
data2 None
None
PragmaComparer
A comparer function for the LinePragma class - for use with SortedList
PrintData
print numbers of nodes in CxList
data None
Query
The query that originated the debug message
QueryParamsProvider
Thread safe. Parameter name format: QP_name.type.vendor[.lang.details] type - in|ex vendor - cx|cust
QueryParamsProviderCache
Cache wraper for a IQueryParamsProvider. Thread safe if underlying IQueryParamsProvider (provided in the constructor) is thread safe.
ReduceFlow
Returns CxList which is a subset of instance CxList and consists of longest paths to/from destination element for CxList.ReduceFlowType.ReduceSmallFlow parameter or shortest paths to/from destination element for CxList.ReduceFlowType.ReduceBigFlow parameter.
Expand source
public CxList ReduceFlow (CxList.ReduceFlowType flowType)
flowType The type of flow for reduce: CxList.ReduceFlowType.ReduceBigFlow CxList.ReduceFlowType.ReduceSmallFlow
Returns CxList which is a subset of paths that consists of longest paths or shortest paths to/from destination element, depending on ReduceFlow methods parameter.
ArgumentNullException : parameter is a null reference
The return value may be empty (Count = 0).
This example demonstrates the CxList. ReduceFlow () method.
The input source code is:
Expand source
ArrayList<String> lst = new ArrayList<String>(); public void setString(String str) { if (str.length > 0) { lst.add(str); } else { String otherStr = "string is empty"; lst.add(otherStr); } }
The source code that uses CxList.ReduceFlow method:
Expand source
CxList paths = All.DataInfluencingOn(All.FindByShortName("add"));
1.
Expand source
result = paths.ReduceFlow(CxList.ReduceFlowType.ReduceBigFlow);
The result would consist of 4 items:
Expand source
all ending at add (in lst.add(otherStr);) starting lst(in lst.add(str)) str(in lst.add(str)) lst(in lst.add(otherStr);) otherStr(in lst.add(otherStr);)
2.
Expand source
result = paths.ReduceFlow(CxList.ReduceFlowType.ReduceSmallFlow);
The result would consist of 4 items:
Expand source
all ending at add (in lst.add(otherStr);) starting lst(in ArrayList<String> lst = new ArrayList<String>();) ending add(in lst.add(str);) starting lst(in lst.add(str)) ending add(in lst.add(otherStr);) starting str(in (String str)) ending add(in lst.add(str);) starting "string is empty" (in String otherStr = "string is empty";) ending add(in lst.add(otherStr);)
Supported from 7.1.2
ReduceFlowByPragma
Returns a CxList which is a subset of instance CxList and consists of shortest paths from path starting line to path end line.
Expand source
public CxList ReduceFlowByPragma ()
Returns a CxList which are shortest paths from path starting line to path end line.
ArgumentNullException : parameter is a null reference
The return value may be empty (Count = 0).
This example demonstrates the CxList. ReduceFlowByPragma () method.
The input source code is:
Expand source
public void setString () { String otherStr = otherStr; lst.add(otherStr); }
The source code that uses CxList.ReduceFlowByPragma method:
Expand source
CxList paths = All.DataInfluencedBy(All.FindByShortName("otherStr")); result = paths.ReduceFlowByPragma();
The result would consist of 4 items:
Expand source
starts in otherStr of (String otherStr) ends in otherStr of (lst.add(otherStr);) starts in otherStr of(= otherStr;) ends in otherStr of(String otherStr) starts in otherStr of(String otherStr) ends in add of(lst.add(otherStr);) starts in otherStr of(lst.add(otherStr);) ends in add of(lst.add(otherStr);)
Supported from 7.1.2
reduce path that its start and end equivalent by line pragma to another by path if exists data without paths and with the same line pragma then all double nodes will be removed Algorithm include 4 stages 1. Find all data with the same line pragma and remove them if there are no paths "connected" to the data. 2. Remove all paths with the same line pragma and add to result remained paths. 3. Add to result "anchor" of those paths. 4. Add to result all data that left after stage 1 without paths.
reduced CxListImpl
ReducePaths
remove all flows that NOT begun with input parameter vertexes currently not in use
interfaceVertexes None
None
create new PathData with reduced paths according input parameter
reduceType None
None
ReducePathsByLinePragma
create new PathData with reduced paths
None
create new PathData with reduced paths
None
RemoveCommentCode
Replaces comments of specific languages with spaces.
fileContent The contents of the file
pattern The patter to search and replace with white spaces
The contents of the file with comments replaced with white spaces
RemoveNonCommentCode
Replaces non comment code with white spaces
fileStr The content of the file
pattern the pattern to search and replace with spaces
The content of the file where non comment code is replaced with spaces
ReplaceWithEmpty
Replace input with empty chars, maintaining the line terminators
SanitizeCxList
Returns a CxList which is a subset of paths, which are the instance CxList, that doesn't include sanitize nodes.
Expand source
public CxList SanitizeCxList (CxList sanitizer)
sanitizer CxList of sanitizer nodes.
Returns a CxList which is a subset of paths that doesn't include sanitize nodes.
ArgumentNullException : parameter is a null reference
The return value may be empty (Count = 0).
This example demonstrates the CxList. SanitizeCxList () method.
The input source code is:
Expand source
public void setString (String input) { String otherStr = input; lst.add(otherStr); }
The source code that uses CxList.SanitizeCxList method:
Expand source
CxList paths = All.DataInfluencingOn(All.FindByShortName("add")); CxList sanitizeNodes = All.FindByShortName("input"); result = paths.SanitizeCxList(sanitizeNodes);
The result would consist of 3 items:
Expand source
all ends with add in lst.add(otherStr); starts: otherStr(in String otherStr = input;) lst(in lst.add(otherStr);) otherStr(in lst.add(otherStr);)
Supported from 7.1.2
SanitizePathData
return all paths that their nodes not exists in delta (sanitizer)
delta None
None
SearchOnlyInComments
Replaces non comment code with white spaces, distinguishing between the type of comments (hash based, xml based or regular).
curFile The name of the file to process
fileStr The content of the file
The content of the file where non comment code is replaced with spaces
SetTopLevelQueryFlag
Create this object and call this method at the beginning of every query.
If this is the top level query.
StringListTokenizer
Tokenize a list of strings seperated by semicolon (; ). A semicolon sign can be escaped by a backslash (\; ). A backslash can be escaped by another backslash (
). Any other lonely backslash is removed.
TopLevelQueryFlag
Create this object at the beginning of every query code.
TryGetCSharpGraph
Try to extract the DOM object from the first node in 'this' CxList and cast it to type 'T'. Returns null if the CxList is empty, or if the casting fails.
Expand source
public CxList TryGetCSharpGraph<T>()
T The type to cast the DOM object to (must inherit from CSharpGraph)
The DOM object after casting
The source code that uses CxList.TryGetCSharpGraph method:
Expand source
CxList A = All.FindById(10); CSharpGraph cs = A.TryGetCSharpGraph<CSharpGraph>(); // If A contains at least 1 node, cs will contain its DOM object
Supported from 8.4.0
WriteDebugMessage
Display string to DebugMessages tab in CxAudit program
Expand source
public void cxLog.WriteDebugMessage(object obj)
obj Object to be displayed.
ArgumentNullException : parameter is a null reference
All calling to cxLog.WriteDebugMessage should be removed from production version!!! It also prints the debug message into the CxSAST log file(even outside CxAudit).
This example demonstrates the cxLog.WriteDebugMessage method.
The input source code is:
Expand source
class cl1 { void foo() { int a = 3; int b = 5; } }
The source code that uses WriteDebugMessage method:
Expand source
result = All.FindByShortName("foo"); if (result.Count > 0) cxLog.WriteDebugMessage(result.GetFirstGraph().ShortName); cxLog.WriteDebugMessage("number of DOM elements =" + All.Count);
The result would be - on DebugMessage tab in CxAudit program
Expand source
foo number of DOM elements = 14
Supported from v1.8.1
Not to be commented.
Saves a debug message, specifying the query that generated it
query The query name that includes the call to the WriteDebugMessage
line The line the call to the WriteDebugMessage was issued
obj The object to log
Saves a debug message, specifying the query that generated it
query The query name that includes the call to the WriteDebugMessage
line The line the call to the WriteDebugMessage was issued
obj The object to log
containGraph
Calculate if first graph contains second or wise versa “Contains” means that first and last vertexec of graph presents in another graph.
first None
second None
None
getAllInternalPath
get all paths in internal representation. each path can appears only once. key of those paths shouldn't be an object with an empty name (like =)
None